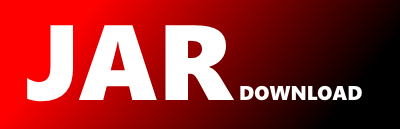
org.appdapter.gui.util.InitialBoxedContext Maven / Gradle / Ivy
Show all versions of org.appdapter.lib.gui Show documentation
package org.appdapter.gui.util;
import static org.appdapter.gui.util.CollectionSetUtils.addIfNew;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Hashtable;
import java.util.LinkedList;
import javax.naming.Binding;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.Name;
import javax.naming.NameClassPair;
import javax.naming.NameParser;
import javax.naming.NamingEnumeration;
import javax.naming.NamingException;
import javax.naming.NoInitialContextException;
import javax.naming.spi.NamingManager;
import org.appdapter.core.debug.UIAnnotations.HRKRefinement;
import org.appdapter.core.log.Debuggable;
import org.appdapter.gui.util.NamingResolver.SavedFromResolverMap;
import org.appdapter.gui.util.ObjectFinder.Found;
/**
*
* NoInitialContextException is thrown when an initial context cannot be
* instantiated. This exception can be thrown during any interaction with the
* InitialContext, not only when the InitialContext is constructed. For example,
* the implementation of the initial context might lazily retrieve the context
* only when actual methods are invoked on it. The application should not have
* any dependency on when the existence of an initial context is determined.
*
*
* @author Administrator
*
*/
public class InitialBoxedContext extends InitialContext implements Context, HRKRefinement {
Context ctx;
public Context getRealCtx() {
if (ctx == null)
try {
return getDefaultInitCtx();
} catch (NamingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return ctx;
}
/**
* Retrieves a context for resolving the string name name
. If
* name
name is a URL string, then attempt to find a URL
* context for it. If none is found, or if name
is not a URL
* string, then return getDefaultInitCtx()
.
*
* See getURLOrDefaultInitCtx(Name) for description of how a subclass should
* use this method.
*
* @param name
* The non-null name for which to get the context.
* @return A URL context for name
or the cached initial
* context. The result cannot be null.
* @exception NoInitialContextException
* If cannot find an initial context.
* @exception NamingException
* In a naming exception is encountered.
* @see javax.naming.spi.NamingManager#getURLContext
*/
@Override public Context getURLOrDefaultInitCtx(String name) throws NamingException {
if (NamingManager.hasInitialContextFactoryBuilder()) {
return getDefaultInitCtx();
}
String scheme = getURLScheme(name);
if (scheme != null) {
Context ctx0 = NamingManager.getURLContext(scheme, myProps);
if (ctx0 != null) {
return ctx0;
}
}
return getDefaultInitCtx();
}
/**
* Retrieves a context for resolving name
. If the first
* component of name
name is a URL string, then attempt to find
* a URL context for it. If none is found, or if the first component of
* name
is not a URL string, then return
* getDefaultInitCtx()
.
*
* When creating a subclass of InitialContext, use this method as follows.
* Define a new method that uses this method to get an initial context of
* the desired subclass.
*
*
*
*
* protected XXXContext getURLOrDefaultInitXXXCtx(Name name)
* throws NamingException {
* Context answer = getURLOrDefaultInitCtx(name);
* if (!(answer instanceof XXXContext)) {
* if (answer == null) {
* throw new NoInitialContextException();
* } else {
* throw new NotContextException("Not an XXXContext");
* }
* }
* return (XXXContext) answer;
* }
*
*
*
When providing implementations for the new methods in the
* subclass, use this newly defined method to get the initial context.
*
*
*
*
* public Object XXXMethod1(Name name, ...) {
* throws NamingException {
* return getURLOrDefaultInitXXXCtx(name).XXXMethod1(name, ...);
* }
*
*
*
*
* @param name
* The non-null name for which to get the context.
* @return A URL context for name
or the cached initial
* context. The result cannot be null.
* @exception NoInitialContextException
* If cannot find an initial context.
* @exception NamingException
* In a naming exception is encountered.
*
* @see javax.naming.spi.NamingManager#getURLContext
*/
@Override public Context getURLOrDefaultInitCtx(Name name) throws NamingException {
if (NamingManager.hasInitialContextFactoryBuilder()) {
return getDefaultInitCtx();
}
if (name.size() > 0) {
String first = name.get(0);
String scheme = getURLScheme(first);
if (scheme != null) {
Context ctxL = NamingManager.getURLContext(scheme, myProps);
if (ctxL != null) {
return ctxL;
}
}
}
return getDefaultInitCtx();
}
private static String getURLScheme(String str) {
int colon_posn = str.indexOf(':');
int slash_posn = str.indexOf('/');
if (colon_posn > 0 && (slash_posn == -1 || colon_posn < slash_posn))
return str.substring(0, colon_posn);
return null;
}
/**
* Retrieves the initial context by calling
* NamingManager.getInitialContext()
and cache it in
* defaultInitCtx. Set gotDefault
so that we know we've tried
* this before.
*
* @return The non-null cached initial context.
* @exception NoInitialContextException
* If cannot find an initial context.
* @exception NamingException
* If a naming exception was encountered.
*/
@Override final protected Context getDefaultInitCtx() throws NamingException {
if (!gotDefault) {
defaultInitCtx = NamingManager.getInitialContext(myProps);
gotDefault = true;
}
if (defaultInitCtx == null)
throw new NoInitialContextException();
return defaultInitCtx;
}
public InitialBoxedContext() throws NamingException {
super();
ctx = new InitialContext();
initAtLeastOnce();
// TODO Auto-generated constructor stub
}
public InitialBoxedContext(Hashtable, ?> environment) throws NamingException {
super(environment);
ctx = new InitialContext(environment);
initAtLeastOnce();
// TODO Auto-generated constructor stub
}
private void initAtLeastOnce() {
Debuggable.notImplemented();
}
protected InitialBoxedContext(boolean lazy) throws NamingException {
super(lazy);
ctx = null;
initAtLeastOnce();
// TODO Auto-generated constructor stub
}
public InitialBoxedContext(Context context) throws NamingException {
super();
context = ctx;
initAtLeastOnce();
}
@Override public Object lookup(String name) throws NamingException {
return lookup(getURLOrDefaultInitCtx(name), name, null);
}
public O lookup(Class class1) throws NamingException {
String name = class1.getSimpleName();
return lookup(getURLOrDefaultInitCtx(name), name, class1);
}
public static O lookup(Context context, String name, Class class1) throws NamingException {
O obj = lookup(context, name, class1, false);
if (obj != null)
return obj;
obj = lookup(context, name, class1, true);
if (obj != null)
return obj;
return obj;
}
@SuppressWarnings("unchecked") public static O lookup(Context context, String name, Class class1, boolean createIfNotFound) throws NamingException {
String lname = name;
if (name == null) {
name = class1.getSimpleName();
}
try {
return (O) context.lookup(lname);
} catch (javax.naming.ServiceUnavailableException e) {
for (NamingResolver res : namingResolvers) {
Collection