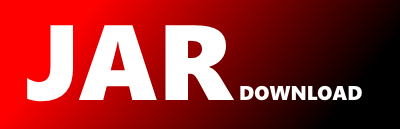
org.appdapter.registry.basic.BasicFinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.appdapter.lib.registry Show documentation
Show all versions of org.appdapter.lib.registry Show documentation
Pluggable object registration + query service
/*
* Copyright 2012 by The Appdapter Project (www.appdapter.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.appdapter.registry.basic;
import java.util.ArrayList;
import java.util.List;
import org.appdapter.api.registry.Pattern;
import org.appdapter.api.registry.Receiver;
import org.appdapter.api.registry.ResultSequence;
import org.appdapter.api.registry.SimpleFinder;
/**
* @author Stu B.
*/
public class BasicFinder implements SimpleFinder {
private BasicRegistry myBasicRegistry;
private Class myObjClz;
public BasicFinder(BasicRegistry br, Class otClz) {
myBasicRegistry = br;
myObjClz = otClz;
}
protected ResultSequence makeResultSequence(Pattern p, Receiver r) {
ResultSequence rseq = new ResultSequence();
rseq.myFinder = this;
rseq.myPattern = p;
rseq.myReceiver = r;
return rseq;
}
@Override public ResultSequence deliverMatchesUntilDone(Pattern p, Receiver r) {
ResultSequence rseq = makeResultSequence(p, r);
List brutishMatches = myBasicRegistry.brutishlyCollectAllMatches(myObjClz, p);
Receiver.Status stat = Receiver.Status.SEEKING;
for (OT obj : brutishMatches) {
stat = rseq.deliverResult(obj);
if (stat.equals(Receiver.Status.DONE)) {
break;
}
}
return rseq;
}
protected List collectMatches(Pattern p) {
final List matches = new ArrayList();
Receiver collector = new Receiver() {
@Override public Status receiveMatch(OT match, ResultSequence seq, long seqIndex) {
matches.add(match);
return Status.SEEKING;
}
};
deliverMatchesUntilDone(p, collector);
return matches;
}
protected void verifyMatchCount(int matchCount, int minAllowed, int maxAllowed, Pattern patForError) throws Exception {
if ((matchCount < minAllowed) || (matchCount > maxAllowed)) {
throw new Exception("Expected between " + minAllowed + " and " + maxAllowed + " matches for "
+ patForError + ", but got " + matchCount);
}
}
@Override public OT findFirstMatch(Pattern p, int minAllowed, int maxAllowed) throws Exception {
// TODO: Do not need to iterate further than maxAllowed, which is usually not higher than 2.
// TODO: Sanity checks on minAllowed + maxAllowed args.
List matches = collectMatches(p);
int matchCount = matches.size();
verifyMatchCount(matchCount, minAllowed, maxAllowed, p);
return (matchCount > 0) ? matches.get(0) : null;
}
@Override public List findAllMatches(Pattern p, int minAllowed, int maxAllowed) throws Exception {
List matches = collectMatches(p);
int matchCount = matches.size();
verifyMatchCount(matchCount, minAllowed, maxAllowed, p);
return matches;
}
@Override public long countMatches(Pattern p, int maxAllowed) throws Exception {
// TODO: this can be done much more efficiently by delivering to a null receiver (for starters!)
List matches = collectMatches(p);
int matchCount = matches.size();
verifyMatchCount(matchCount, 0, maxAllowed, p);
return matches.size();
}
@Override public void killDeliverySequence(ResultSequence resultSeq) {
throw new UnsupportedOperationException("Not supported yet.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy