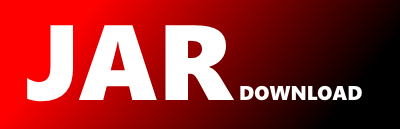
org.appfuse.service.impl.GenericManagerImpl Maven / Gradle / Ivy
package org.appfuse.service.impl;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.appfuse.dao.GenericDao;
import org.appfuse.service.GenericManager;
import org.springframework.beans.factory.annotation.Autowired;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
/**
* This class serves as the Base class for all other Managers - namely to hold
* common CRUD methods that they might all use. You should only need to extend
* this class when your require custom CRUD logic.
*
* To register this class in your Spring context file, use the following XML.
*
* <bean id="userManager" class="org.appfuse.service.impl.GenericManagerImpl">
* <constructor-arg>
* <bean class="org.appfuse.dao.hibernate.GenericDaoHibernate">
* <constructor-arg value="org.appfuse.model.User"/>
* <property name="sessionFactory" ref="sessionFactory"/>
* </bean>
* </constructor-arg>
* </bean>
*
*
* If you're using iBATIS instead of Hibernate, use:
*
* <bean id="userManager" class="org.appfuse.service.impl.GenericManagerImpl">
* <constructor-arg>
* <bean class="org.appfuse.dao.ibatis.GenericDaoiBatis">
* <constructor-arg value="org.appfuse.model.User"/>
* <property name="dataSource" ref="dataSource"/>
* <property name="sqlMapClient" ref="sqlMapClient"/>
* </bean>
* </constructor-arg>
* </bean>
*
*
* @param a type variable
* @param the primary key for that type
* @author Matt Raible
* Updated by jgarcia: added full text search + reindexing
*/
public class GenericManagerImpl implements GenericManager {
/**
* Log variable for all child classes. Uses LogFactory.getLog(getClass()) from Commons Logging
*/
protected final Log log = LogFactory.getLog(getClass());
/**
* GenericDao instance, set by constructor of child classes
*/
protected GenericDao dao;
public GenericManagerImpl() {
}
public GenericManagerImpl(GenericDao genericDao) {
this.dao = genericDao;
}
/**
* {@inheritDoc}
*/
public List getAll() {
return dao.getAll();
}
/**
* {@inheritDoc}
*/
public T get(PK id) {
return dao.get(id);
}
/**
* {@inheritDoc}
*/
public boolean exists(PK id) {
return dao.exists(id);
}
/**
* {@inheritDoc}
*/
public T save(T object) {
return dao.save(object);
}
/**
* {@inheritDoc}
*/
public void remove(T object) {
dao.remove(object);
}
/**
* {@inheritDoc}
*/
public void remove(PK id) {
dao.remove(id);
}
/**
* {@inheritDoc}
*
* Search implementation using Hibernate Search.
*/
@SuppressWarnings("unchecked")
public List search(String q, Class clazz) {
if (q == null || "".equals(q.trim())) {
return getAll();
}
return dao.search(q);
}
/**
* {@inheritDoc}
*/
public void reindex() {
dao.reindex();
}
/**
* {@inheritDoc}
*/
public void reindexAll(boolean async) {
dao.reindexAll(async);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy