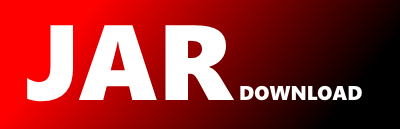
org.appng.api.support.OptionFactory Maven / Gradle / Ivy
/*
* Copyright 2011-2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.appng.api.support;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import org.appng.api.model.Identifiable;
import org.appng.api.model.NameProvider;
import org.appng.api.model.Named;
import org.appng.api.support.OptionOwner.HitCounter;
import org.appng.api.support.OptionOwner.Selector;
import org.appng.xml.platform.Option;
abstract class OptionFactory {
protected abstract T getOwner(String id, String titleId);
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Named} elements. For the {@link Option}'s id,
* {@link Named#getId()} is used. If no {@link NameProvider} is given, {@link Named#getName()()} is used for the
* {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElements
* the selected elements
* @param nameProvider
* a {@link NameProvider} (optional)
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromNamed(String id, String titleId, Iterable extends N> allElements,
Collection extends N> selectedElements, NameProvider nameProvider) {
T owner = getOwner(id, titleId);
addNamedOptions(allElements, selectedElements, owner, nameProvider);
return owner;
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Named} elements. For the {@link Option}'s id,
* {@link Named#getId()} is used. If no {@link NameProvider} is given, {@link Named#getName()()} is used for the
* {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElement
* the selected element
* @param nameProvider
* a {@link NameProvider} (optional)
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromNamed(String id, String titleId, Iterable extends N> allElements,
N selectedElement, NameProvider nameProvider) {
T owner = getOwner(id, titleId);
addNamedOptions(allElements, Arrays.asList(selectedElement), owner, nameProvider);
return owner;
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Named} elements. For the {@link Option}'s id,
* {@link Named#getId()} is used. {@link Named#getName()()} is used for the {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElements
* the selected elements
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromNamed(String id, String titleId, Iterable extends N> allElements,
Collection extends N> selectedElements) {
return fromNamed(id, titleId, allElements, selectedElements, null);
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Named} elements. For the {@link Option}'s id,
* {@link Named#getId()} is used. {@link Named#getName()()} is used for the {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElement
* the selected element
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromNamed(String id, String titleId, Iterable extends N> allElements,
N selectedElement) {
return fromNamed(id, titleId, allElements, Arrays.asList(selectedElement), null);
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Named} elements. For the {@link Option}'s id,
* {@link Named#getId()} is used. {@link Named#getName()()} is used for the {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selector
* a {@link org.appng.api.support.OptionOwner.Selector}
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromNamed(String id, String titleId, Iterable extends N> allElements,
Selector selector) {
return fromNamed(id, titleId, allElements, selector, null);
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Named} elements. For the {@link Option}'s id,
* {@link Named#getId()} is used. If no {@link NameProvider} is given, {@link Named#getName()()} is used for the
* {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selector
* a {@link org.appng.api.support.OptionOwner.Selector}
* @param nameProvider
* a {@link NameProvider} (optional)
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromNamed(String id, String titleId, Iterable extends N> allElements,
Selector selector, NameProvider nameProvider) {
T owner = getOwner(id, titleId);
addNamedOptions(allElements, new ArrayList<>(), owner, nameProvider);
applySelector(owner, selector);
return owner;
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Identifiable} elements. For the
* {@link Option}'s id, {@link Identifiable#getId()} is used, for the value {@link #toString()}.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElements
* the selected elements
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromIdentifiable(String id, String titleId, Iterable extends I> allElements,
Collection extends I> selectedElements) {
T owner = getOwner(id, titleId);
addIdentifiableOptions(allElements, selectedElements, owner, null);
return owner;
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Identifiable} elements. For the
* {@link Option}'s id, {@link Identifiable#getId()} is used, for the value {@link #toString()}.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElement
* the selected element
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromIdentifiable(String id, String titleId, Iterable extends I> allElements,
I selectedElement) {
return fromIdentifiable(id, titleId, allElements, selectedElement, null);
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Identifiable} elements. For the
* {@link Option}'s id, {@link Identifiable#getId()} is used. If no {@link NameProvider} is given,
* {@link #toString()} is used for the {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElement
* the selected element
* @param nameProvider
* a {@link NameProvider} (optional)
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromIdentifiable(String id, String titleId, Iterable extends I> allElements,
I selectedElement, NameProvider nameProvider) {
return fromIdentifiable(id, titleId, allElements, Arrays.asList(selectedElement), nameProvider);
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Identifiable} elements. For the
* {@link Option}'s id, {@link Identifiable#getId()} is used. If no {@link NameProvider} is given,
* {@link #toString()} is used for the {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElements
* the selected elements
* @param nameProvider
* a {@link NameProvider} (optional)
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromIdentifiable(String id, String titleId, Iterable extends I> allElements,
Collection extends I> selectedElements, NameProvider nameProvider) {
T owner = getOwner(id, titleId);
addIdentifiableOptions(allElements, selectedElements, owner, nameProvider);
return owner;
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Identifiable} elements. For the
* {@link Option}'s id, {@link Identifiable#getId()} is used, for the value {@link #toString()}.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selector
* a {@link org.appng.api.support.OptionOwner.Selector}
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromIdentifiable(String id, String titleId, Iterable extends I> allElements,
Selector selector) {
return fromIdentifiable(id, titleId, allElements, selector, null);
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Identifiable} elements. For the
* {@link Option}'s id, {@link Identifiable#getId()} is used. If no {@link NameProvider} is given,
* {@link #toString()} is used for the {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selector
* a {@link org.appng.api.support.OptionOwner.Selector}
* @param nameProvider
* a {@link NameProvider} (optional)
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromIdentifiable(String id, String titleId, Iterable extends I> allElements,
Selector selector, NameProvider nameProvider) {
T owner = getOwner(id, titleId);
addIdentifiableOptions(allElements, new ArrayList<>(), owner, nameProvider);
applySelector(owner, selector);
return owner;
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Enum} elements. For both, the {@link Option}
* 's id and value, {@link Enum#name()} is used.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElements
* the selected elements
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromEnum(String id, String titleId, E[] allElements, Collection selectedElements) {
return fromEnum(id, titleId, allElements, selectedElements, null);
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Enum} elements. For both, the {@link Option}
* 's id and value, {@link Enum#name()} is used.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElement
* the selected element
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromEnum(String id, String titleId, E[] allElements, E selectedElement) {
return fromEnum(id, titleId, allElements, selectedElement, null);
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Enum} elements. For the {@link Option}'s id,
* {@link Enum#name()} is used. If no {@link NameProvider} is given, {@link Enum#name()} is also used for the
* {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElements
* the selected elements
* @param nameProvider
* a {@link NameProvider} (optional)
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromEnum(String id, String titleId, E[] allElements, Collection selectedElements,
NameProvider nameProvider) {
T owner = getOwner(id, titleId);
addEnumOptions(allElements, selectedElements, owner, nameProvider);
return owner;
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Enum} elements. For the {@link Option}'s id,
* {@link Enum#name()} is used. If no {@link NameProvider} is given, {@link Enum#name()} is also used for the
* {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElement
* the selected element
* @param nameProvider
* a {@link NameProvider} (optional)
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public > T fromEnum(String id, String titleId, E[] allElements, E selectedElement,
NameProvider nameProvider) {
return fromEnum(id, titleId, allElements, Arrays.asList(selectedElement), nameProvider);
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Object}s. For both, the {@link Option}'s id
* and value, {@link Object#toString()} is used.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selector
* a {@link org.appng.api.support.OptionOwner.Selector}
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public T fromObjects(String id, String titleId, S[] allElements, Selector selector) {
return fromObjects(id, titleId, allElements, selector, null);
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Object}s. For the {@link Option}'s id,
* {@link Object#toString()} is used. If no {@link NameProvider} is given, {@link Object#toString()} is also used
* for the {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selector
* a {@link org.appng.api.support.OptionOwner.Selector}
* @param nameProvider
* a {@link NameProvider} (optional)
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public T fromObjects(String id, String titleId, S[] allElements, Selector selector,
NameProvider nameProvider) {
T owner = getOwner(id, titleId);
addOptions(allElements, new ArrayList<>(), owner, nameProvider);
applySelector(owner, selector);
return owner;
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Object}s. For both, the {@link Option}'s id
* and value, {@link Object#toString()} is used.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElements
* the selected elements
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public T fromObjects(String id, String titleId, S[] allElements, S... selectedElements) {
return fromObjects(id, titleId, allElements, null, selectedElements);
}
/**
* Builds and returns a new {@link OptionOwner} using the given {@link Object}s. For the {@link Option}'s id,
* {@link Object#toString()} is used. If no {@link NameProvider} is given, {@link Object#toString()} is also used
* for the {@link Option}'s value.
*
* @param id
* the id of the {@link OptionOwner}
* @param titleId
* the title-id of the {@link OptionOwner} (see {@link org.appng.xml.platform.Label#getId()}).
* @param allElements
* the elements to create {@link Option}s from
* @param selectedElements
* the selected elements
* @param nameProvider
* a {@link NameProvider} (optional)
* @return a new {@link OptionOwner} (actually a {@link org.appng.xml.platform.Selection} or a
* {@link org.appng.xml.platform.OptionGroup})
*/
public T fromObjects(String id, String titleId, S[] allElements, NameProvider nameProvider,
S... selectedElements) {
T owner = getOwner(id, titleId);
addOptions(allElements, Arrays.asList(selectedElements), owner, nameProvider);
return owner;
}
/**
* Counts and sets the hits for the options based on their value.
*
* @param options
* some options
* @param counter
* a {@link HitCounter}
*
* @see Option#getValue()
* @see Option#getHits()
*/
public void countHits(Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy