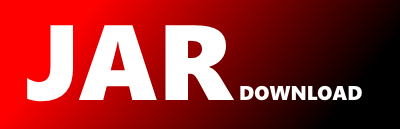
org.appops.cache.helper.GuavaCacheHelper Maven / Gradle / Ivy
/*
* AppOps is a Java framework to develop, deploy microservices with ease and is available for free
* and common use developed by AinoSoft ( www.ainosoft.com )
*
* AppOps and AinoSoft are registered trademarks of Aino Softwares private limited, India.
*
* Copyright (C) <2016>
*
* This program is free software: you can redistribute it and/or modify it under the terms of the
* GNU General Public License as published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version along with applicable additional terms as
* provisioned by GPL 3.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without
* even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* General Public License for more details.
*
* You should have received a copy of the GNU General Public License and applicable additional terms
* along with this program.
*
* If not, see and
*/
package org.appops.cache.helper;
import com.google.inject.Inject;
import java.util.HashMap;
import org.appops.cache.impl.GuavaCacheImpl;
import org.appops.cache.util.GuavaMetricUtils;
/**
* Helper class for {@link org.appops.cache.impl.GuavaCacheImpl}.
*
* @author suraj
* @version $Id: $Id
*/
public class GuavaCacheHelper {
private GuavaMetricUtils guavaMetricUtils;
/**
* Default constructor.
*/
public GuavaCacheHelper() {
}
/**
* Parameterized constructor.
*
* @param guavaMetricUtils instance of {@link org.appops.cache.util.GuavaMetricUtils}
*/
@Inject
public GuavaCacheHelper(GuavaMetricUtils guavaMetricUtils) {
this.guavaMetricUtils = guavaMetricUtils;
}
/**
* Associates given value with given key in the cache. If the cache previously contained a value
* associated with given key, the old value is replaced by given value.
*
* @param key key value
* @param value value associated with given key
*/
public void put(String key, String value) {
guavaMetricUtils.put(key, value);
}
/**
* It copies all of the mappings from the specified map to the cache.
*
* @param map the map which copy into cache.
*/
public void putAll(HashMap map) {
guavaMetricUtils.putAll(map);
}
/**
* It returns the value associated with given key in this cache.
*
* @param key key value
* @return return the value associated with given key otherwise null.
*/
public String getIfPresent(String key) {
return guavaMetricUtils.getIfPresent(key);
}
/**
* Discards any cached value for key.
*
* @param key discards cache value
*/
public void invalidate(String key) {
guavaMetricUtils.invalidate(key);
}
/**
* It discards the all entries from cache.
*/
public void invalidateAll() {
guavaMetricUtils.invalidateAll();
}
/**
* It discards the values from cache of given keys.
*
* @param keys multiple keys
*/
public void invalidateAll(Iterable keys) {
guavaMetricUtils.invalidateAll(keys);
}
/**
* Performs any pending maintenance operations needed by the cache. Exactly which activities are
* performed -- if any -- is implementation-dependent.
*/
public void cleanUp() {
guavaMetricUtils.cleanUp();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy