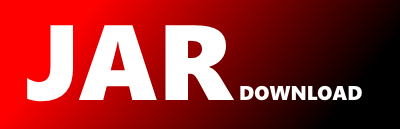
org.appops.cache.impl.ServiceBusImpl Maven / Gradle / Ivy
/*
* AppOps is a Java framework to develop, deploy microservices with ease and is available for free
* and common use developed by AinoSoft ( www.ainosoft.com )
*
* AppOps and AinoSoft are registered trademarks of Aino Softwares private limited, India.
*
* Copyright (C) <2016>
*
* This program is free software: you can redistribute it and/or modify it under the terms of the
* GNU General Public License as published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version along with applicable additional terms as
* provisioned by GPL 3.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without
* even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* General Public License for more details.
*
* You should have received a copy of the GNU General Public License and applicable additional terms
* along with this program.
*
* If not, see and
*/
package org.appops.cache.impl;
import com.google.inject.Inject;
import java.util.Set;
import org.appops.cache.event.Condition;
import org.appops.cache.event.EventSubscriber;
import org.appops.cache.event.ServiceEvent;
import org.appops.cache.event.SubscriberRegistry;
import org.appops.core.annotation.SubscriberListener;
import org.appops.core.mime.MimeType;
import org.appops.core.service.OpParameterMap;
import org.appops.marshaller.DescriptorType;
import org.appops.marshaller.Marshaller;
import org.appops.web.common.client.WebClient;
/**
* A centralized event bus to manage and process all events in appops framework.
*
* @author deba
* @version $Id: $Id
*/
public class ServiceBusImpl implements org.appops.cache.slim.ServiceBus {
private SubscriberRegistry subscriberRegistry;
private Marshaller marshaller;
private WebClient> webClient;
/**
* Constructor for ServiceBusImpl.
*/
public ServiceBusImpl() {
}
@Inject
/**
* Constructor for ServiceBusImpl.
*
* @param subscriberRegistry a {@link org.appops.cache.event.SubscriberRegistry} object.
*/
public ServiceBusImpl(SubscriberRegistry subscriberRegistry) {
this.subscriberRegistry = subscriberRegistry;
}
/**
* {@inheritDoc}
*
* Registers all listener methods i.e. methods annotated with {@link SubscriberListener} listener
* instance passed.
*/
@Override
public Boolean register(EventSubscriber eventSubscriber) {
return subscriberRegistry.register(eventSubscriber);
}
/**
* {@inheritDoc}
*
* Passes event to all registered listeners to process.
*/
@Override
public void fireEvent(ServiceEvent event) {
fireEvent(event, null);
}
/**
* {@inheritDoc}
*
* Passes event to all registered listeners.
*/
@Override
public void fireEvent(ServiceEvent event, Condition condition) {
String eventType = event.getEventType();
Set subscribers = subscriberRegistry.getSubscribers(eventType, condition);
String eventResultJson = marshaller.marshall(event, DescriptorType.JSON);
for (String subscriberUrl : subscribers) {
String urlParameters = populateOpParamMapJson(eventResultJson);
this.webClient.post(subscriberUrl, urlParameters, MimeType.JSON);
}
}
/**
* Populate {@link OpParameterMap } from job token and encrypt {@link OpParameterMap }.
*
* @param token job token which to be process.
* @return encrypted job token .
*/
private String populateOpParamMapJson(String json) {
OpParameterMap map = new OpParameterMap();
map.addParameter(0, "eventResult", json);
return getMarshaller().marshall(map, DescriptorType.JSON);
}
/**
* Getter for the field marshaller
.
*
* @return a {@link org.appops.marshaller.Marshaller} object.
*/
public Marshaller getMarshaller() {
return marshaller;
}
/**
* Setter for the field marshaller
.
*
* @param marshaller a {@link org.appops.marshaller.Marshaller} object.
*/
@Inject
public void setMarshaller(Marshaller marshaller) {
this.marshaller = marshaller;
}
/**
* Getter for the field webClient
.
*
* @return a {@link org.appops.web.common.client.WebClient} object.
*/
public WebClient> getWebClient() {
return webClient;
}
/**
* Setter for the field webClient
.
*
* @param webClient a {@link org.appops.web.common.client.WebClient} object.
*/
@Inject
public void setWebClient(WebClient webClient) {
this.webClient = webClient;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy