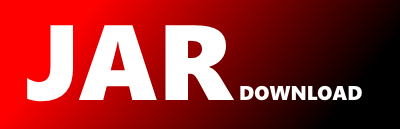
org.appops.service.crypto.ContentCrypto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appops-br-service-base Show documentation
Show all versions of appops-br-service-base Show documentation
appops black rhino service base
/*
* AppOps is a Java framework to develop, deploy microservices with ease and is available for free
* and common use developed by AinoSoft ( www.ainosoft.com )
*
* AppOps and AinoSoft are registered trademarks of Aino Softwares private limited, India.
*
* Copyright (C) <2016>
*
* This program is free software: you can redistribute it and/or modify it under the terms of the
* GNU General Public License as published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version along with applicable additional terms as
* provisioned by GPL 3.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without
* even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* General Public License for more details.
*
* You should have received a copy of the GNU General Public License and applicable additional terms
* along with this program.
*
* If not, see and
*/
package org.appops.service.crypto;
import java.nio.charset.StandardCharsets;
import java.security.Key;
import java.security.spec.KeySpec;
import java.util.Base64;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.crypto.Cipher;
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.PBEKeySpec;
import javax.crypto.spec.SecretKeySpec;
/**
* A class which use AES algorithm for content encryption and decryption.
*
* @author deba
* @version $Id: $Id
*/
public class ContentCrypto {
private static Logger logger = Logger.getLogger(ContentCrypto.class.getCanonicalName());
private static final String Key = "Foo12345Foo12345";
/**
* method used for encrypt the text.
*
* @param textToEncrypt is the type of String.
* @return encrypted string
*/
public String encrypt(String textToEncrypt) {
String str = null;
try {
Key aesKey = new SecretKeySpec(Key.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, aesKey);
byte[] encrypted = cipher.doFinal(textToEncrypt.getBytes((StandardCharsets.UTF_8)));
str = Base64.getEncoder().encodeToString(encrypted);
} catch (Exception e) {
logger.log(Level.SEVERE, "Exception occurred in encrypt() :: ", e);
}
return str;
}
/**
* method used for decrypt the text.
*
* @param encrypted is the type String
* @return decrypted string
*/
public String decrypt(String encrypted) {
String decrypted = null;
try {
byte[] encrypt = Base64.getDecoder().decode(encrypted);
Key aesKey = new SecretKeySpec(Key.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, aesKey);
decrypted = new String(cipher.doFinal(encrypt));
} catch (Exception e) {
decrypted = encrypted;
logger.log(Level.WARNING,
"Unable to decrypt string, may be it was not encrypted properly :: " + e.getMessage());
}
return decrypted;
}
/**
* method used for encrypt the text using provided key. Here key is considered as date and time
* format.
*
* @param textToEncrypt is the type of String.
* @param key which is use for encryption
* @return encrypted string
*/
public static String encryptUsingKey(String textToEncrypt, String key) {
String str = null;
try {
key = getPlainTextFromDateString(key);
// key length should be 16 bytes
key = ("0000000000000000" + key).substring(key.length());
Key aesKey = new SecretKeySpec(key.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, aesKey);
byte[] encrypted = cipher.doFinal(textToEncrypt.getBytes((StandardCharsets.UTF_8)));
str = Base64.getEncoder().encodeToString(encrypted);
} catch (Exception e) {
logger.log(Level.SEVERE, "Exception occurred in encryptUsingKey() :: ", e);
}
return str;
}
/**
* method used for decrypt the text using provided key.Here key is considered as date and time
* format.
*
* @param encrypted is the type String
* @param key which is use for decryption
* @return decrypted string
*/
public static String decryptUsingKey(String encrypted, String key) {
String decrypted = null;
try {
key = getPlainTextFromDateString(key);
// key length should be 16 bytes
key = ("0000000000000000" + key).substring(key.length());
byte[] encrypt = Base64.getDecoder().decode(encrypted);
Key aesKey = new SecretKeySpec(key.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, aesKey);
decrypted = new String(cipher.doFinal(encrypt));
} catch (Exception e) {
decrypted = encrypted;
logger.log(Level.WARNING,
"Unable to decrypt string, may be it was not encrypted properly :: " + e.getMessage());
}
return decrypted;
}
/**
* It encrypts the given image content using given dateString as a key and return encrypted image
* content.
*
* @param dateStringKey date in string which is used as a key
* @param byteArrayInputStream array of bytes contains image content
* @return encrypted content
*/
public static byte[] encryptImageFileContent(String dateStringKey, byte[] byteArrayInputStream) {
try {
Cipher cipher;
byte[] encrypted = null;
byte[] salt = new byte[16];
dateStringKey = getPlainTextFromDateString(dateStringKey);
byte[] dateStringB =
Base64.getDecoder().decode(dateStringKey.getBytes(StandardCharsets.UTF_8));
KeySpec spec = new PBEKeySpec(new String(dateStringB).toCharArray(), salt, 65536, 256);
SecretKeyFactory f = SecretKeyFactory.getInstance("PBKDF2WithHmacSHA1");
byte[] key = f.generateSecret(spec).getEncoded();
SecretKeySpec keySpec = new SecretKeySpec(key, "AES");
try {
cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, keySpec);
encrypted = cipher.doFinal(byteArrayInputStream);
} catch (Exception e) {
logger.log(Level.SEVERE,
"Exception occurred while encrypting a text in encryptImageFile()::" + e);
}
return encrypted;
} catch (Exception e) {
logger.log(Level.SEVERE,
"Exception occurred creating secret key in encryptImageFile()::" + e);
}
return null;
}
/**
* It decryptes the given image content using given dateString as a key and return decrypted image
* content.
*
* @param dateStringKey date in string which is used as a key
* @param bytesOfInputStream array of bytes
* @return decrypted content
*/
public static byte[] decryptImageFileContent(String dateStringKey, byte[] bytesOfInputStream) {
try {
Cipher cipher;
byte[] decrypted = null;
byte[] salt = new byte[16];
dateStringKey = getPlainTextFromDateString(dateStringKey);
byte[] dateStringB =
Base64.getDecoder().decode(dateStringKey.getBytes(StandardCharsets.UTF_8));
KeySpec spec = new PBEKeySpec(new String(dateStringB).toCharArray(), salt, 65536, 256);
SecretKeyFactory f = SecretKeyFactory.getInstance("PBKDF2WithHmacSHA1");
byte[] key = f.generateSecret(spec).getEncoded();
SecretKeySpec keySpec = new SecretKeySpec(key, "AES");
try {
cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, keySpec);
decrypted = cipher.doFinal(bytesOfInputStream);
} catch (Exception e) {
logger.log(Level.SEVERE,
"Exception occurred while decrypting a text in decryptImageFile()::" + e);
}
return decrypted;
} catch (Exception e) {
logger.log(Level.SEVERE,
"Exception occurred creating secret key in decryptImageFile()::" + e);
}
return null;
}
private static String getPlainTextFromDateString(String dateString) {
String plainText = null;
plainText = dateString.replaceAll("\\s", "");
plainText = plainText.replaceAll("[\\-\\:,]", "");
return plainText;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy