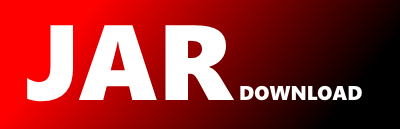
org.appops.service.request.RestResponseWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appops-br-service-base Show documentation
Show all versions of appops-br-service-base Show documentation
appops black rhino service base
/*
* AppOps is a Java framework to develop, deploy microservices with ease and is available for free
* and common use developed by AinoSoft ( www.ainosoft.com )
*
* AppOps and AinoSoft are registered trademarks of Aino Softwares private limited, India.
*
* Copyright (C) <2016>
*
* This program is free software: you can redistribute it and/or modify it under the terms of the
* GNU General Public License as published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version along with applicable additional terms as
* provisioned by GPL 3.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without
* even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* General Public License for more details.
*
* You should have received a copy of the GNU General Public License and applicable additional terms
* along with this program.
*
* If not, see and
*/
package org.appops.service.request;
import com.google.inject.Inject;
import com.google.inject.Provider;
import java.io.IOException;
import java.io.PrintWriter;
import java.nio.charset.StandardCharsets;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.lang.ArrayUtils;
import org.appops.core.mime.MimeType;
import org.appops.core.response.BlobResponse;
import org.appops.marshaller.DescriptorType;
import org.appops.marshaller.Marshaller;
import org.appops.service.client.handler.ClientHandler;
/**
* Converts sand sends appropriate http response.
*
* @author deba
* @version $Id: $Id
*/
public class RestResponseWriter {
private Marshaller marshaller;
private Provider clientHandlerProvider;
/**
* it sends the response.
*
* @param response it is the http servlet response.
* @param result is the final result
* @param resultMimeType is the result of mime type.
* @throws java.io.IOException if any.
*/
public void sendResponse(HttpServletResponse response, Object result, String resultMimeType)
throws IOException {
Object resultRet = clientHandlerProvider.get().constructResult(result);
response.setCharacterEncoding(StandardCharsets.UTF_8.name());
if (MimeType.XML.value().equals(resultMimeType)) {
sendXml(resultRet, response);
} else if (MimeType.TEXT.value().equals(resultMimeType)) {
sendText(result, response);
} else if (MimeType.BLOB.value().equals(resultMimeType)) {
sendBlob((BlobResponse) result, response);
} else {
sendJson(resultRet, response);
}
}
/**
* It sends the blob by looking their result mime type.
*
* @param result instance of {@link BlobResponse} contains final result
* @param response it is the http servlet response
* @throws IOException occurred when the is i/0 error
*/
private void sendBlob(BlobResponse result, HttpServletResponse response) throws IOException {
response.setContentType(result.getMimeType());
String fileName = result.getFileName();
if (fileName != null && !fileName.isEmpty()) {
response.setHeader("Content-Disposition", "filename=" + fileName);
}
Byte[] blobArrayObj = result.getByteArray();
byte[] bytes = ArrayUtils.toPrimitive(blobArrayObj);
response.setContentLength(bytes.length);
response.getOutputStream().write(bytes);
response.getOutputStream().flush();
response.getOutputStream().close();
}
/**
* it sends the text.
*
* @param result it is the final result.
* @param response it is the http servlet response.
* @throws IOException occurred when there is i/o error.
*/
void sendText(Object result, HttpServletResponse response) throws IOException {
PrintWriter writer = response.getWriter();
String text = String.valueOf(result);
response.setContentType("text/plain");
writer.println(text);
writer.close();
}
/**
* it sends the JSON.
*
* @param result it is the final result.
* @param response it is the http servlet response.
* @throws IOException occurred when there is i/o error.
*/
void sendJson(Object result, HttpServletResponse response) throws IOException {
PrintWriter writer = response.getWriter();
response.setContentType("application/json");
String json = "";
if (result instanceof String) {
json = (String) result;
} else {
json = getMarshaller().marshall(result, DescriptorType.JSON);
}
writer.println(json);
writer.flush();
writer.close();
}
/**
* it sends the xml.
*
* @param result it is the final result.
* @param response it is the http servlet response.
* @throws IOException occurred when there is i/o error.
*/
void sendXml(Object result, HttpServletResponse response) throws IOException {
PrintWriter writer = response.getWriter();
response.setContentType("text/xml");
String xml = getMarshaller().marshall(result, DescriptorType.XML);
writer.println(xml);
writer.flush();
writer.close();
}
/**
*
* Getter for the field marshaller
.
*
*
* @return a {@link org.appops.marshaller.Marshaller} object.
*/
public Marshaller getMarshaller() {
return marshaller;
}
/**
*
* Setter for the field marshaller
.
*
*
* @param marshaller a {@link org.appops.marshaller.Marshaller} object.
*/
@Inject
public void setMarshaller(Marshaller marshaller) {
this.marshaller = marshaller;
}
/**
*
* Getter for the field clientHandlerProvider
.
*
*
* @return a {@link com.google.inject.Provider} object.
*/
public Provider getClientHandlerProvider() {
return clientHandlerProvider;
}
/**
*
* Setter for the field clientHandlerProvider
.
*
*
* @param clientHandlerProvider a {@link com.google.inject.Provider} object.
*/
@Inject
public void setClientHandlerProvider(Provider clientHandlerProvider) {
this.clientHandlerProvider = clientHandlerProvider;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy