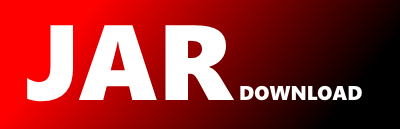
org.appops.service.job.pool.JobPool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appops-br-service-base Show documentation
Show all versions of appops-br-service-base Show documentation
appops black rhino service base
/*
* AppOps is a Java framework to develop, deploy microservices with ease and is available for free
* and common use developed by AinoSoft ( www.ainosoft.com )
*
* AppOps and AinoSoft are registered trademarks of Aino Softwares private limited, India.
*
* Copyright (C) <2016>
*
* This program is free software: you can redistribute it and/or modify it under the terms of the
* GNU General Public License as published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version along with applicable additional terms as
* provisioned by GPL 3.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without
* even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* General Public License for more details.
*
* You should have received a copy of the GNU General Public License and applicable additional terms
* along with this program.
*
* If not, see and
*/
package org.appops.service.job.pool;
import com.google.inject.Inject;
import com.google.inject.Injector;
import java.lang.reflect.Method;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import javax.inject.Provider;
import org.appops.cache.event.ServiceEvent;
import org.appops.cache.slim.ServiceBus;
import org.appops.core.ClassPathAnalyser;
import org.appops.core.annotation.Config;
import org.appops.core.annotation.JobEntry;
import org.appops.core.job.token.JobToken;
import org.appops.service.event.JobEvent;
import org.appops.service.exception.InvocationException;
/**
* A pool responsible for job scheduling and execution.
*
* @author deba
* @version $Id: $Id
*/
public class JobPool {
private ExecutorService execService;
@Inject
private Injector injector;
private Provider serviceBus;
/**
*
* setConfig.
*
*
* @param config a {@link org.appops.service.job.pool.JobPoolConfig} object.
*/
@Inject
public void setConfig(@Config JobPoolConfig config) {
execService = initPool(config.getMaxPoolSize());
}
private ExecutorService initPool(int maxPoolSize) {
return Executors.newFixedThreadPool(maxPoolSize);
}
/**
* Submits jobs for execution.
*
* @param job Job to be executed.
* @param parameters job token paramaters.
* @param jobToken job token to be submit for execution.
* @param Type of result.
* @return Future instance containing result.
*/
public Future submitJob(Object job, Object[] parameters, JobToken jobToken) {
synchronized (this) {
Future future = getExecService().submit(new Callable() {
@Override
public R call() throws Exception {
Method method = getJobEntryMethod(job.getClass());
fireJobStartedEvent(jobToken);
Object returnValue = method.invoke(job, parameters);
fireJobCompletedEvent(returnValue, jobToken);
return (R) returnValue;
}
});
return future;
}
}
/**
* It constructs the job event data information and fires job started event.
*
* @param jobToken instance of {@link org.appops.core.job.token.JobToken}
*/
protected void fireJobStartedEvent(JobToken jobToken) {
ServiceEvent serviceEvent = new ServiceEvent();
serviceEvent.setEventType(JobEvent.class.getCanonicalName());
serviceEvent.setEventStatus(org.appops.service.event.JobEvent.STARTED);
org.appops.service.event.JobEvent jobEvent = new JobEvent();
jobEvent.withToken(jobToken);
serviceEvent.setEventData(jobEvent.getEventData());
getServiceBus().get().fireEvent(serviceEvent);
}
/**
* It constructs the job event data and fires job completed event.
*
* @param returnValue invoked method return value
* @param jobToken instance of {@link org.appops.core.job.token.JobToken}
*/
protected void fireJobCompletedEvent(Object returnValue, JobToken jobToken) {
ServiceEvent serviceEvent = new ServiceEvent();
serviceEvent.setEventType(JobEvent.class.getCanonicalName());
serviceEvent.setEventStatus(JobEvent.COMPLETED);
org.appops.service.event.JobEvent jobEvent = new JobEvent();
jobEvent.withToken(jobToken);
jobEvent.withJobResult(returnValue);
serviceEvent.setEventData(jobEvent.getEventData());
getServiceBus().get().fireEvent(serviceEvent);
}
/**
* Find method from provided job class whcih have {@link JobEntry} annotation.if more than one
* method has {@link JobEntry} annotation then it will throw an exception.
*
* @param jobClazz job class name which operation to be invoke.
* @return method which have {@link JobEntry} annotation.
*/
private Method getJobEntryMethod(Class> jobClazz) {
List methods =
injector.getInstance(ClassPathAnalyser.class).getAnnotatedMethods(jobClazz, JobEntry.class);
if (methods.isEmpty()) {
throw new InvocationException(
"Method must have " + JobEntry.class.getName() + " annotation for execution.");
}
if (methods.size() > 1) {
throw new InvocationException(
"Found more than one method with " + JobEntry.class.getName() + " annotation.");
}
return methods.get(0);
}
/**
*
* Getter for the field execService
.
*
*
* @return a {@link java.util.concurrent.ExecutorService} object.
*/
public ExecutorService getExecService() {
return execService;
}
/**
*
* Getter for the field serviceBus
.
*
*
* @return a {@link javax.inject.Provider} object.
*/
public Provider getServiceBus() {
return serviceBus;
}
/**
*
* Setter for the field serviceBus
.
*
*
* @param serviceBus a {@link javax.inject.Provider} object.
*/
@Inject
public void setServiceBus(Provider serviceBus) {
this.serviceBus = serviceBus;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy