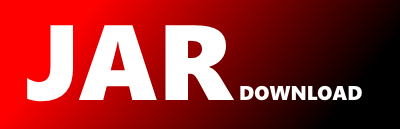
org.appspy.perf.data.PerfTimerData Maven / Gradle / Ivy
/*
* Copyright (c) 2008 appspy.org, Contributors.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software distributed under the
* License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language governing permissions
* and limitations under the License. */
package org.appspy.perf.data;
import org.appspy.core.data.AbstractCollectedData;
import org.appspy.core.data.CollectedData;
/**
* @author Olivier HEDIN / [email protected]
*/
public abstract class PerfTimerData extends AbstractCollectedData implements CollectedData
{
protected long mTimerDelay = 0;
protected long mBlockingDelay = 0;
protected long mBlockingCount = 0;
protected long mCPUTime = 0;
protected long mUserCPUTime = 0;
protected long mWaitDelay = 0;
protected long mWaitCount = 0;
protected int mTimerType = PerfTimerType.SERVER;
protected int mResult = 0;
protected String mOrganization = null;
protected String mEnvironment = null;
protected String mApplication = null;
protected String mVersion = null;
protected String mHost = null;
/**
* @return the timerDelay
*/
public long getTimerDelay() {
return mTimerDelay;
}
/**
* @param timerDelay the timerDelay to set
*/
public void setTimerDelay(long timerDelay) {
mTimerDelay = timerDelay;
}
/**
* @return the blockingDelay
*/
public long getBlockingDelay() {
return mBlockingDelay;
}
/**
* @param blockingDelay the blockingDelay to set
*/
public void setBlockingDelay(long blockingDelay) {
mBlockingDelay = blockingDelay;
}
/**
* @return the blockingCount
*/
public long getBlockingCount() {
return mBlockingCount;
}
/**
* @param blockingCount the blockingCount to set
*/
public void setBlockingCount(long blockingCount) {
mBlockingCount = blockingCount;
}
/**
* @return the cPUTime
*/
public long getCPUTime() {
return mCPUTime;
}
/**
* @param time the cPUTime to set
*/
public void setCPUTime(long time) {
mCPUTime = time;
}
/**
* @return the userCPUTime
*/
public long getUserCPUTime() {
return mUserCPUTime;
}
/**
* @param userCPUTime the userCPUTime to set
*/
public void setUserCPUTime(long userCPUTime) {
mUserCPUTime = userCPUTime;
}
/**
* @return the waitDelay
*/
public long getWaitDelay() {
return mWaitDelay;
}
/**
* @param waitDelay the waitDelay to set
*/
public void setWaitDelay(long waitDelay) {
mWaitDelay = waitDelay;
}
/**
* @return the waitCount
*/
public long getWaitCount() {
return mWaitCount;
}
/**
* @param waitCount the waitCount to set
*/
public void setWaitCount(long waitCount) {
mWaitCount = waitCount;
}
/**
* @return the timerType
*/
public int getTimerType() {
return mTimerType;
}
/**
* @param timerType the timerType to set
*/
public void setTimerType(int timerType) {
mTimerType = timerType;
}
/**
* @return the organization
*/
public String getOrganization() {
return mOrganization;
}
/**
* @param organization the organization to set
*/
public void setOrganization(String organization) {
mOrganization = organization;
}
/**
* @return the environment
*/
public String getEnvironment() {
return mEnvironment;
}
/**
* @param environment the environment to set
*/
public void setEnvironment(String environment) {
mEnvironment = environment;
}
/**
* @return the application
*/
public String getApplication() {
return mApplication;
}
/**
* @param application the application to set
*/
public void setApplication(String application) {
mApplication = application;
}
/**
* @return the version
*/
public String getVersion() {
return mVersion;
}
/**
* @param version the version to set
*/
public void setVersion(String version) {
mVersion = version;
}
/**
* @return the host
*/
public String getHost() {
return mHost;
}
/**
* @param host the host to set
*/
public void setHost(String host) {
mHost = host;
}
/**
* @return the result
*/
public int getResult() {
return mResult;
}
/**
* @param result the result to set
*/
public void setResult(int result) {
mResult = result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy