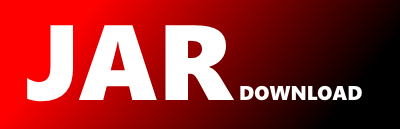
org.appspy.perf.data.ServletTimerData Maven / Gradle / Ivy
/*
* Copyright (c) 2008 appspy.org, Contributors.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software distributed under the
* License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language governing permissions
* and limitations under the License. */
package org.appspy.perf.data;
import java.util.ArrayList;
import java.util.List;
/**
* @author Olivier HEDIN / [email protected]
*/
public class ServletTimerData extends PerfTimerData {
/**
*
*/
private static final long serialVersionUID = 1L;
protected String mContextPath = null;
protected String mURL = null;
protected int mResponseCode = 200;
protected String mHttpMethod = null;
protected String mRemoteUser = null;
protected String mSessionId = null;
protected boolean mIsNewSession = false;
protected String mRemoteIP = null;
protected int mQueryStringSize = 0;
protected long mInputHeaderSize = 0;
protected long mInputStreamSize = 0;
protected long mOutputHeaderSize = 0;
protected long mOutputStreamSize = 0;
protected List mParameters = new ArrayList();
/**
* @return the contextPath
*/
public String getContextPath() {
return mContextPath;
}
/**
* @param contextPath the contextPath to set
*/
public void setContextPath(String contextPath) {
mContextPath = contextPath;
}
/**
* @return the uRL
*/
public String getURL() {
return mURL;
}
/**
* @param url the uRL to set
*/
public void setURL(String url) {
mURL = url;
}
/**
* @return the responseCode
*/
public int getResponseCode() {
return mResponseCode;
}
/**
* @param responseCode the responseCode to set
*/
public void setResponseCode(int responseCode) {
mResponseCode = responseCode;
}
/**
* @return the httpMethod
*/
public String getHttpMethod() {
return mHttpMethod;
}
/**
* @param httpMethod the httpMethod to set
*/
public void setHttpMethod(String httpMethod) {
mHttpMethod = httpMethod;
}
/**
* @return the remoteUser
*/
public String getRemoteUser() {
return mRemoteUser;
}
/**
* @param remoteUser the remoteUser to set
*/
public void setRemoteUser(String remoteUser) {
mRemoteUser = remoteUser;
}
/**
* @return the sessionId
*/
public String getSessionId() {
return mSessionId;
}
/**
* @param sessionId the sessionId to set
*/
public void setSessionId(String sessionId) {
mSessionId = sessionId;
}
/**
* @return the isNewSession
*/
public boolean isNewSession() {
return mIsNewSession;
}
/**
* @param isNewSession the isNewSession to set
*/
public void setNewSession(boolean isNewSession) {
mIsNewSession = isNewSession;
}
/**
* @return the remoteIP
*/
public String getRemoteIP() {
return mRemoteIP;
}
/**
* @param remoteIP the remoteIP to set
*/
public void setRemoteIP(String remoteIP) {
mRemoteIP = remoteIP;
}
/**
* @return the queryStringSize
*/
public int getQueryStringSize() {
return mQueryStringSize;
}
/**
* @param queryStringSize the queryStringSize to set
*/
public void setQueryStringSize(int queryStringSize) {
mQueryStringSize = queryStringSize;
}
/**
* @return the inputHeaderSize
*/
public long getInputHeaderSize() {
return mInputHeaderSize;
}
/**
* @param inputHeaderSize the inputHeaderSize to set
*/
public void setInputHeaderSize(long inputHeaderSize) {
mInputHeaderSize = inputHeaderSize;
}
/**
* @return the inputStreamSize
*/
public long getInputStreamSize() {
return mInputStreamSize;
}
/**
* @param inputStreamSize the inputStreamSize to set
*/
public void setInputStreamSize(long inputStreamSize) {
mInputStreamSize = inputStreamSize;
}
/**
* @return the outputHeaderSize
*/
public long getOutputHeaderSize() {
return mOutputHeaderSize;
}
/**
* @param outputHeaderSize the outputHeaderSize to set
*/
public void setOutputHeaderSize(long outputHeaderSize) {
mOutputHeaderSize = outputHeaderSize;
}
/**
* @return the outputStreamSize
*/
public long getOutputStreamSize() {
return mOutputStreamSize;
}
/**
* @param outputStreamSize the outputStreamSize to set
*/
public void setOutputStreamSize(long outputStreamSize) {
mOutputStreamSize = outputStreamSize;
}
/**
* @return the parameters
*/
public List getParameters() {
return mParameters;
}
/**
* @param parameters the parameters to set
*/
public void setParameters(List parameters) {
mParameters = parameters;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy