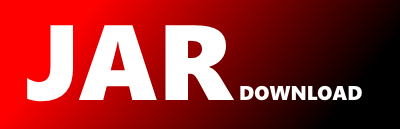
legolas.async.api.interfaces.Promise Maven / Gradle / Ivy
package legolas.async.api.interfaces;
import java.util.concurrent.CompletableFuture;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Supplier;
public class Promise {
private final CompletableFuture future;
private Throwable cause;
private Boolean succeded = Boolean.FALSE;
private Boolean failed = Boolean.FALSE;
private T result;
private Promise(Supplier supplier) {
this(CompletableFuture.supplyAsync(supplier));
}
Promise(CompletableFuture future) {
this.future = future;
BiConsumer completionConsumer = (result, cause) -> {
if (cause != null) {
this.failed = Boolean.TRUE;
this.cause = cause;
} else {
this.succeded = Boolean.TRUE;
this.result = result;
}
};
this.future.whenComplete(completionConsumer).whenCompleteAsync(completionConsumer);
}
public static Promise create() {
return new Promise<>(new CompletableFuture<>());
}
public static Promise create(Supplier supplier) {
return new Promise<>(supplier);
}
public static Promise failed(Throwable throwable) {
CompletableFuture future = new CompletableFuture();
future.completeExceptionally(throwable);
return new Promise(future);
}
public static Promise succeeded() {
return succeeded(null);
}
public static Promise succeeded(T value) {
CompletableFuture future = new CompletableFuture();
future.complete(value);
return new Promise(future);
}
public void complete() {
this.future.complete(null);
}
public void complete(T value) {
this.future.complete(value);
}
public void fail(Throwable throwable) {
this.future.completeExceptionally(throwable);
}
public T get() {
try {
return this.future.get();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public Boolean succeded() {
return this.succeded;
}
public Boolean failed() {
return failed;
}
public Throwable cause() {
return cause;
}
public T result() {
return result;
}
CompletableFuture asFuture() {
return future;
}
static class EmptyConsumer implements Consumer {
@Override
public void accept(Object o) {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy