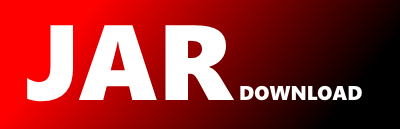
com.argot.compiler.ArgotVisitor Maven / Gradle / Ivy
// Generated from com/argot/compiler/Argot.g4 by ANTLR 4.7.2
package com.argot.compiler;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link ArgotParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface ArgotVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link ArgotParser#file}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFile(ArgotParser.FileContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#headers}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHeaders(ArgotParser.HeadersContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#headerline}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHeaderline(ArgotParser.HeaderlineContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#cluster}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCluster(ArgotParser.ClusterContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#definition}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDefinition(ArgotParser.DefinitionContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#relation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRelation(ArgotParser.RelationContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#sequence}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSequence(ArgotParser.SequenceContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#tag}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTag(ArgotParser.TagContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#reserve}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReserve(ArgotParser.ReserveContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#load}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLoad(ArgotParser.LoadContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#importl}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImportl(ArgotParser.ImportlContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpression(ArgotParser.ExpressionContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#expressionIdentifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpressionIdentifier(ArgotParser.ExpressionIdentifierContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#primary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPrimary(ArgotParser.PrimaryContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#array}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArray(ArgotParser.ArrayContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#typeIdentifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeIdentifier(ArgotParser.TypeIdentifierContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#value}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValue(ArgotParser.ValueContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#mappedIdentifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMappedIdentifier(ArgotParser.MappedIdentifierContext ctx);
/**
* Visit a parse tree produced by {@link ArgotParser#identifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIdentifier(ArgotParser.IdentifierContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy