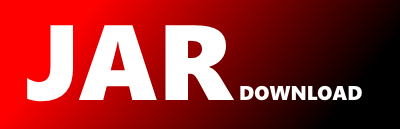
org.arquillian.spacelift.loader.ServiceRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arquillian-spacelift-api Show documentation
Show all versions of arquillian-spacelift-api Show documentation
Arquillian Process and Package Manager
/*
* JBoss, Home of Professional Open Source
* Copyright 2012, Red Hat Middleware LLC, and individual contributors
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.arquillian.spacelift.loader;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
/**
* A simple singleton (application scoped} registry that fulfills {@link ServiceLoader} contract. This registry uses
* underlying
* {@link ServiceLoader} implementation to load the service while storing cached instances in a local map.
*
* @author Karel Piwko
*/
public class ServiceRegistry implements ServiceLoader {
private static ServiceRegistry instance;
private ServiceLoader serviceLoader;
private Map, Collection>> cachedServiceInstances;
/**
* Creates a service registry with a {@link ServiceLoader} to load service instances until they are cached
*/
public ServiceRegistry(ServiceLoader serviceLoader) {
this.serviceLoader = serviceLoader;
this.cachedServiceInstances = Collections.synchronizedMap(new HashMap, Collection>>());
}
/**
* Returns {@link ServiceRegistry} instance. Instance must be registered first.
*
* @throws IllegalStateException
* If no service registry was registered yet
*/
public static synchronized ServiceRegistry getInstance() throws IllegalStateException {
if (instance == null) {
throw new IllegalStateException("Unable to get instance of Service Registry, it was not initialized.");
}
return instance;
}
/**
* Registers an instance of {@link ServiceRegistry}.
*/
public static synchronized void register(ServiceRegistry registry) {
instance = registry;
}
/**
* Loads all registered services for given {@code serviceClass}
*
* @throws IllegalArgumentException
* If {@code serviceClass} is {@code null}
*/
@SuppressWarnings("unchecked")
@Override
public Collection all(Class serviceClass) throws IllegalArgumentException {
if (serviceClass == null) {
throw new IllegalArgumentException("ServiceClass must be provided");
}
synchronized (cachedServiceInstances) {
if (cachedServiceInstances.containsKey(serviceClass)) {
return (Collection) cachedServiceInstances.get(serviceClass);
}
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy