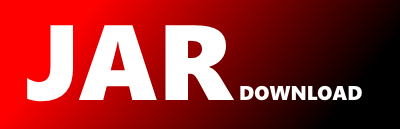
org.arquillian.spacelift.process.ProcessInteractionBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arquillian-spacelift-api Show documentation
Show all versions of arquillian-spacelift-api Show documentation
Arquillian Process and Package Manager
/*
* JBoss, Home of Professional Open Source
* Copyright 2013, Red Hat Middleware LLC, and individual contributors
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.arquillian.spacelift.process;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Pattern;
/**
* Builder API for process interaction. It uses regular expression to match allowed and error output.
*
* @author Karel Piwko
* @see ProcessInteraction
*/
public class ProcessInteractionBuilder {
/**
* No interaction instance
*/
public static final ProcessInteraction NO_INTERACTION = new ProcessInteractionBuilder().build();
private String textTypedIn;
private Map replyMap;
private List allowedOutput;
private List errorOutput;
private List terminatingOutput;
private OutputTransformer transformer;
private Pattern lastPattern;
/**
* Creates empty interaction builder
*/
public ProcessInteractionBuilder() {
this.replyMap = new LinkedHashMap();
this.allowedOutput = new ArrayList();
this.errorOutput = new ArrayList();
this.terminatingOutput = new ArrayList();
this.transformer = null;
}
/**
* Defines an interaction when {@code pattern} is matched
*
* @param pattern
* the line
*
* @return current instance to allow chaining
*/
public MatchedOutputProcessInteractionBuilder when(String pattern) {
this.lastPattern = Pattern.compile(pattern);
return new MatchedOutputProcessInteractionBuilder();
}
/**
* Defines an interaction when process is started
*
* @return current instance to allow chaining
*/
public StartingProcessInteractionBuilder whenStarts() {
return new StartingProcessInteractionBuilder();
}
/**
* Defines a prefix for standard output and standard error output. Might be {@code null} or empty string, in such case
* no
* prefix is added and process outputs cannot be distinguished
*
* @param prefix
* the prefix
*
* @return current instance to allow chaining
*/
public ProcessInteractionBuilder outputPrefix(final String prefix) {
if (prefix == null || "".equals(prefix)) {
this.transformer = new OutputTransformer() {
@Override
public Sentence transform(Sentence output) {
return output;
}
};
} else {
// sets prefix output transformer
this.transformer = new OutputTransformer() {
@Override
public Sentence transform(Sentence output) {
return output.prepend(prefix);
}
};
}
return this;
}
/**
* Builds {@link ProcessInteraction} object from defined data
*
* @return {@link ProcessInteraction}
*/
public ProcessInteraction build() {
return new ProcessInteractionImpl(replyMap, transformer, allowedOutput, errorOutput, terminatingOutput,
textTypedIn);
}
private static class ProcessInteractionImpl implements ProcessInteraction {
private final String textTypedIn;
private final Map replyMap;
private final List allowedOutput;
private final List errorOutput;
private final List terminatingOutput;
private final OutputTransformer transformer;
public ProcessInteractionImpl(Map replyMap, OutputTransformer outputTransformer,
List allowedOutput,
List errorOutput, List terminatingOutput, String textTypedIn) {
this.replyMap = replyMap;
this.transformer = outputTransformer;
this.allowedOutput = allowedOutput;
this.errorOutput = errorOutput;
this.terminatingOutput = terminatingOutput;
this.textTypedIn = textTypedIn;
}
@Override
public List allowedOutput() {
return allowedOutput;
}
@Override
public List errorOutput() {
return errorOutput;
}
@Override
public Map replyMap() {
return replyMap;
}
@Override
public List terminatingOutput() {
return terminatingOutput;
}
@Override
public String textTypedIn() {
return textTypedIn;
}
@Override
public OutputTransformer transformer() {
return transformer;
}
}
/**
* Definition of allowed actions when process starts
*
* @author Karel Piwko
*/
public class StartingProcessInteractionBuilder {
/**
* Types in the {@code sentence} when process is started
*
* @param sentence
* the sentence
*
* @return current instance to allow chaining
*/
public ProcessInteractionBuilder typeIn(String sentence) {
textTypedIn = sentence;
return ProcessInteractionBuilder.this;
}
}
/**
* Definition of allowed actions when output is matched
*
* @author Karel Piwko
*/
public class MatchedOutputProcessInteractionBuilder {
/**
* Prints the {@code response} to stdin of the process
*
* @param response
* the response
*
* @return current instance to allow chaining
*/
public ProcessInteractionBuilder replyWith(String response) {
replyMap.put(lastPattern, response);
return ProcessInteractionBuilder.this;
}
/**
* Forces current process to terminate
*
* @return current instance to allow chaining
*/
public ProcessInteractionBuilder terminate() {
terminatingOutput.add(lastPattern);
return ProcessInteractionBuilder.this;
}
/**
* Echoes the line to standard output of the process running Spacelift
*
* @return current instance to allow chaining
*/
public ProcessInteractionBuilder printToOut() {
allowedOutput.add(lastPattern);
return ProcessInteractionBuilder.this;
}
/**
* Echoes the line to error output of the process running Spacelift
*
* @return current instance to allow chaining
*/
public ProcessInteractionBuilder printToErr() {
errorOutput.add(lastPattern);
return ProcessInteractionBuilder.this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy