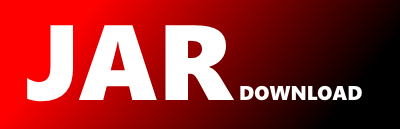
org.asciidoctor.ast.StructuralNode Maven / Gradle / Ivy
package org.asciidoctor.ast;
import java.util.List;
import java.util.Map;
public interface StructuralNode extends ContentNode {
/**
* Constant for special character replacement substitution like {@code <} to {@code <}.
* @see Special Character Substitutions
*/
String SUBSTITUTION_SPECIAL_CHARACTERS = "specialcharacters";
/**
* Constant for quote replacements like {@code *bold*} to {@code bold}.
* @see Quotes Substitutions
*/
String SUBSTITUTION_QUOTES = "quotes";
/**
* Constant for attribute replacements like {@code {foo}}.
* @see Attribute References Substitution
*/
String SUBSTITUTION_ATTRIBUTES = "attributes";
/**
* Constant for replacements like {@code (C)} to {@code ©}.
* @see Character Replacement Substitutions
*/
String SUBSTITUTION_REPLACEMENTS = "replacements";
/**
* Constant for macro replacements like {@code mymacro:target[]}.
* @see Macro Substitutions
*/
String SUBSTITUTION_MACROS = "macros";
/**
* Constant for post replacements like creating line breaks from a trailing {@code +} in a line.
* @see Post Replacement Substitutions
*/
String SUBSTITUTION_POST_REPLACEMENTS = "post_replacements";
/**
* @deprecated Please use {@linkplain #getTitle()} instead
*/
@Deprecated
String title();
String getTitle();
void setTitle(String title);
String getCaption();
void setCaption(String caption);
/**
* @deprecated Please use {@linkplain #getStyle()} instead
*/
@Deprecated
String style();
String getStyle();
void setStyle(String style);
/**
* @return The list of child blocks of this block
* @deprecated Please use {@linkplain #getBlocks()} instead
*/
@Deprecated
List blocks();
/**
* @return The list of child blocks of this block
*/
List getBlocks();
/**
* Appends a new child block as the last block to this block.
* @param block The new child block added as last child to this block.
*/
void append(StructuralNode block);
/**
* @deprecated Please use {@linkplain #getContent()} instead
*/
@Deprecated
Object content();
Object getContent();
String convert();
List findBy(Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy