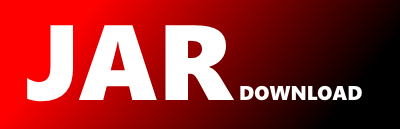
org.eclipse.osgi.container.ModuleRevision Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aspectjtools Show documentation
Show all versions of aspectjtools Show documentation
Tools from the AspectJ project
/*******************************************************************************
* Copyright (c) 2012, 2016 IBM Corporation and others.
*
* This program and the accompanying materials
* are made available under the terms of the Eclipse Public License 2.0
* which accompanies this distribution, and is available at
* https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.osgi.container;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import org.eclipse.osgi.container.ModuleRevisionBuilder.GenericInfo;
import org.eclipse.osgi.container.namespaces.EquinoxModuleDataNamespace;
import org.eclipse.osgi.internal.container.InternalUtils;
import org.osgi.framework.Bundle;
import org.osgi.framework.Version;
import org.osgi.framework.namespace.IdentityNamespace;
import org.osgi.framework.wiring.BundleCapability;
import org.osgi.framework.wiring.BundleRequirement;
import org.osgi.framework.wiring.BundleRevision;
import org.osgi.resource.Capability;
import org.osgi.resource.Requirement;
/**
* An implementation of {@link BundleRevision}.
* @since 3.10
*/
public final class ModuleRevision implements BundleRevision {
private final String symbolicName;
private final Version version;
private final int types;
private final List capabilities;
private final List requirements;
private final ModuleRevisions revisions;
private final Object revisionInfo;
private volatile Boolean lazyActivationPolicy = null;
ModuleRevision(String symbolicName, Version version, int types, List capabilityInfos, List requirementInfos, ModuleRevisions revisions, Object revisionInfo) {
this.symbolicName = symbolicName;
this.version = version;
this.types = types;
this.capabilities = createCapabilities(capabilityInfos);
this.requirements = createRequirements(requirementInfos);
this.revisions = revisions;
this.revisionInfo = revisionInfo;
}
private List createCapabilities(List capabilityInfos) {
if (capabilityInfos == null || capabilityInfos.isEmpty())
return Collections.emptyList();
List result = new ArrayList<>(capabilityInfos.size());
for (GenericInfo info : capabilityInfos) {
if (info.mutable) {
result.add(new ModuleCapability(info.namespace, copyUnmodifiableMap(info.directives), copyUnmodifiableMap(info.attributes), this));
} else {
result.add(new ModuleCapability(info.namespace, info.directives, info.attributes, this));
}
}
return result;
}
private static Map copyUnmodifiableMap(Map map) {
int size = map.size();
if (size == 0) {
return Collections.emptyMap();
}
if (size == 1) {
Map.Entry entry = map.entrySet().iterator().next();
return Collections.singletonMap(entry.getKey(), entry.getValue());
}
return Collections.unmodifiableMap(new HashMap<>(map));
}
private List createRequirements(List requirementInfos) {
if (requirementInfos == null || requirementInfos.isEmpty())
return Collections.emptyList();
List result = new ArrayList<>(requirementInfos.size());
for (GenericInfo info : requirementInfos) {
result.add(new ModuleRequirement(info.namespace, info.directives, info.attributes, this));
}
return result;
}
@Override
public Bundle getBundle() {
return revisions.getBundle();
}
@Override
public String getSymbolicName() {
return symbolicName;
}
@Override
public Version getVersion() {
return version;
}
@Override
public List getDeclaredCapabilities(String namespace) {
return InternalUtils.asListBundleCapability(getModuleCapabilities(namespace));
}
@Override
public List getDeclaredRequirements(String namespace) {
return InternalUtils.asListBundleRequirement(getModuleRequirements(namespace));
}
/**
* Returns the capabilities declared by this revision
* @param namespace The namespace of the declared capabilities to return or
* {@code null} to return the declared capabilities from all namespaces.
* @return An unmodifiable list containing the declared capabilities.
*/
public List getModuleCapabilities(String namespace) {
if (namespace == null)
return Collections.unmodifiableList(capabilities);
List result = new ArrayList<>();
for (ModuleCapability capability : capabilities) {
if (namespace.equals(capability.getNamespace())) {
result.add(capability);
}
}
return Collections.unmodifiableList(result);
}
/**
* Returns the requirements declared by this revision
* @param namespace The namespace of the declared requirements to return or
* {@code null} to return the declared requirements from all namespaces.
* @return An unmodifiable list containing the declared requirements.
*/
public List getModuleRequirements(String namespace) {
if (namespace == null)
return Collections.unmodifiableList(requirements);
List result = new ArrayList<>();
for (ModuleRequirement requirement : requirements) {
if (namespace.equals(requirement.getNamespace())) {
result.add(requirement);
}
}
return Collections.unmodifiableList(result);
}
@Override
public int getTypes() {
return types;
}
@Override
public ModuleWiring getWiring() {
return revisions.getContainer().getWiring(this);
}
@Override
public List getCapabilities(String namespace) {
return InternalUtils.asListCapability(getDeclaredCapabilities(namespace));
}
@Override
public List getRequirements(String namespace) {
return InternalUtils.asListRequirement(getDeclaredRequirements(namespace));
}
/**
* Returns the {@link ModuleRevisions revisions} for this revision.
* @return the {@link ModuleRevisions revisions} for this revision.
*/
public ModuleRevisions getRevisions() {
return revisions;
}
/**
* Returns the revision info for this revision. The revision info is
* assigned when a revision is created to install a module or update module
* @return the revision info for this revision, may be {@code null}.
*/
public Object getRevisionInfo() {
return revisionInfo;
}
/**
* A convenience method to quickly determine if this revision
* has declared the lazy activation policy.
* @return true if the lazy activation policy has been declared by this module; otherwise false is returned.
*/
public boolean hasLazyActivatePolicy() {
Boolean currentPolicy = lazyActivationPolicy;
if (currentPolicy != null) {
return currentPolicy.booleanValue();
}
boolean lazyPolicy = false;
List data = getCapabilities(EquinoxModuleDataNamespace.MODULE_DATA_NAMESPACE);
if (!data.isEmpty()) {
Capability moduleData = data.get(0);
lazyPolicy = EquinoxModuleDataNamespace.CAPABILITY_ACTIVATION_POLICY_LAZY.equals(moduleData.getAttributes().get(EquinoxModuleDataNamespace.CAPABILITY_ACTIVATION_POLICY));
}
lazyActivationPolicy = Boolean.valueOf(lazyPolicy);
return lazyPolicy;
}
boolean isCurrent() {
return !revisions.isUninstalled() && this.equals(revisions.getCurrentRevision());
}
@Override
public String toString() {
List identities = getModuleCapabilities(IdentityNamespace.IDENTITY_NAMESPACE);
if (identities.isEmpty())
return super.toString();
return identities.get(0).toString();
}
static String toString(Map map, boolean directives) {
return toString(map, directives, false);
}
static String toString(Map map, boolean directives, boolean stringsOnly) {
if (map.size() == 0)
return ""; //$NON-NLS-1$
String assignment = directives ? ":=" : "="; //$NON-NLS-1$ //$NON-NLS-2$
Set> set = map.entrySet();
StringBuilder sb = new StringBuilder();
for (Entry entry : set) {
sb.append("; "); //$NON-NLS-1$
String key = entry.getKey();
Object value = entry.getValue();
if (value instanceof List) {
@SuppressWarnings("unchecked")
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy