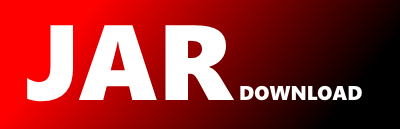
org.eclipse.osgi.internal.debug.FrameworkDebugOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aspectjtools Show documentation
Show all versions of aspectjtools Show documentation
Tools from the AspectJ project
/*******************************************************************************
* Copyright (c) 2003, 2016 IBM Corporation and others.
*
* This program and the accompanying materials
* are made available under the terms of the Eclipse Public License 2.0
* which accompanies this distribution, and is available at
* https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.osgi.internal.debug;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import org.eclipse.osgi.internal.framework.EquinoxConfiguration;
import org.eclipse.osgi.internal.location.LocationHelper;
import org.eclipse.osgi.service.debug.DebugOptions;
import org.eclipse.osgi.service.debug.DebugOptionsListener;
import org.eclipse.osgi.service.debug.DebugTrace;
import org.osgi.framework.BundleContext;
import org.osgi.framework.InvalidSyntaxException;
import org.osgi.framework.ServiceReference;
import org.osgi.util.tracker.ServiceTracker;
import org.osgi.util.tracker.ServiceTrackerCustomizer;
/**
* The DebugOptions implementation class that allows accessing the list of debug options specified
* for the application as well as creating {@link DebugTrace} objects for the purpose of having
* dynamic enablement of debug tracing.
*
* @since 3.1
*/
public class FrameworkDebugOptions implements DebugOptions, ServiceTrackerCustomizer {
private static final String OSGI_DEBUG = "osgi.debug"; //$NON-NLS-1$
private static final String OSGI_DEBUG_VERBOSE = "osgi.debug.verbose"; //$NON-NLS-1$
public static final String PROP_TRACEFILE = "osgi.tracefile"; //$NON-NLS-1$
/** The default name of the .options file if loading when the -debug command-line argument is used */
private static final String OPTIONS = ".options"; //$NON-NLS-1$
/** A lock object used to synchronize access to the trace file */
private final static Object writeLock = new Object();
/** monitor used to lock the options maps */
private final Object lock = new Object();
/** A current map of all the options with values set */
private Properties options = null;
/** A map of all the disabled options with values set at the time debug was disabled */
private Properties disabledOptions = null;
/** A cache of all of the bundles DebugTrace
in the format --> */
protected final Map debugTraceCache = new HashMap<>();
/** The File object to store messages. This value may be null. */
protected File outFile = null;
/** Is verbose debugging enabled? Changing this value causes a new tracing session to start. */
protected boolean verboseDebug = true;
/** A flag to determine if the message being written is done to a new file (i.e. should the header information be written) */
private boolean newSession = true;
private final EquinoxConfiguration environmentInfo;
private volatile BundleContext context;
private volatile ServiceTracker listenerTracker;
public FrameworkDebugOptions(EquinoxConfiguration environmentInfo) {
this.environmentInfo = environmentInfo;
// check if verbose debugging was set during initialization. This needs to be set even if debugging is disabled
this.verboseDebug = Boolean.valueOf(environmentInfo.getConfiguration(OSGI_DEBUG_VERBOSE, Boolean.TRUE.toString())).booleanValue();
// if no debug option was specified, don't even bother to try.
// Must ensure that the options slot is null as this is the signal to the
// platform that debugging is not enabled.
String debugOptionsFilename = environmentInfo.getConfiguration(OSGI_DEBUG);
if (debugOptionsFilename == null)
return;
options = new Properties();
URL optionsFile;
if (debugOptionsFilename.length() == 0) {
// default options location is user.dir (install location may be r/o so
// is not a good candidate for a trace options that need to be updatable by
// by the user)
String userDir = System.getProperty("user.dir").replace(File.separatorChar, '/'); //$NON-NLS-1$
if (!userDir.endsWith("/")) //$NON-NLS-1$
userDir += "/"; //$NON-NLS-1$
debugOptionsFilename = new File(userDir, OPTIONS).toString();
}
optionsFile = LocationHelper.buildURL(debugOptionsFilename, false);
if (optionsFile == null) {
System.out.println("Unable to construct URL for options file: " + debugOptionsFilename); //$NON-NLS-1$
return;
}
System.out.print("Debug options:\n " + optionsFile.toExternalForm()); //$NON-NLS-1$
try {
InputStream input = LocationHelper.getStream(optionsFile);
try {
options.load(input);
System.out.println(" loaded"); //$NON-NLS-1$
} finally {
input.close();
}
} catch (FileNotFoundException e) {
System.out.println(" not found"); //$NON-NLS-1$
} catch (IOException e) {
System.out.println(" did not parse"); //$NON-NLS-1$
e.printStackTrace(System.out);
}
// trim off all the blanks since properties files don't do that.
for (Object key : options.keySet()) {
options.put(key, ((String) options.get(key)).trim());
}
}
public void start(BundleContext bc) {
this.context = bc;
listenerTracker = new ServiceTracker<>(bc, DebugOptionsListener.class.getName(), this);
listenerTracker.open();
}
public void stop(BundleContext bc) {
listenerTracker.close();
listenerTracker = null;
this.context = null;
}
/**
* @see DebugOptions#getBooleanOption(String, boolean)
*/
@Override
public boolean getBooleanOption(String option, boolean defaultValue) {
String optionValue = getOption(option);
return optionValue != null ? optionValue.equalsIgnoreCase("true") : defaultValue; //$NON-NLS-1$
}
/**
* @see DebugOptions#getOption(String)
*/
@Override
public String getOption(String option) {
return getOption(option, null);
}
/**
* @see DebugOptions#getOption(String, String)
*/
@Override
public String getOption(String option, String defaultValue) {
synchronized (lock) {
if (options != null) {
return options.getProperty(option, defaultValue);
}
}
return defaultValue;
}
/**
* @see DebugOptions#getIntegerOption(String, int)
*/
@Override
public int getIntegerOption(String option, int defaultValue) {
String value = getOption(option);
try {
return value == null ? defaultValue : Integer.parseInt(value);
} catch (NumberFormatException e) {
return defaultValue;
}
}
@SuppressWarnings({"unchecked", "rawtypes"})
@Override
public Map getOptions() {
Map snapShot = new HashMap<>();
synchronized (lock) {
if (options != null)
snapShot.putAll((Map) options);
else if (disabledOptions != null)
snapShot.putAll((Map) disabledOptions);
}
return snapShot;
}
/*
* (non-Javadoc)
* @see org.eclipse.osgi.service.debug.DebugOptions#getAllOptions()
*/
String[] getAllOptions() {
String[] optionsArray = null;
synchronized (lock) {
if (options != null) {
optionsArray = new String[options.size()];
final Iterator> entrySetIterator = options.entrySet().iterator();
int i = 0;
while (entrySetIterator.hasNext()) {
Map.Entry
© 2015 - 2024 Weber Informatics LLC | Privacy Policy