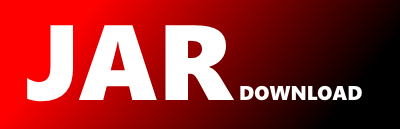
org.asynchttpclient.AsyncHttpClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of async-http-client Show documentation
Show all versions of async-http-client Show documentation
The Async Http Client (AHC) classes.
/*
* Copyright 2010 Ning, Inc.
*
* This program is licensed to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*
*/
package org.asynchttpclient;
import java.io.Closeable;
import java.util.concurrent.Future;
import java.util.function.Predicate;
/**
* This class support asynchronous and synchronous HTTP request.
*
* To execute synchronous HTTP request, you just need to do
*
* AsyncHttpClient c = new AsyncHttpClient();
* Future<Response> f = c.prepareGet(TARGET_URL).execute();
*
*
* The code above will block until the response is fully received. To execute asynchronous HTTP request, you
* create an {@link AsyncHandler} or its abstract implementation, {@link AsyncCompletionHandler}
*
*
* AsyncHttpClient c = new AsyncHttpClient();
* Future<Response> f = c.prepareGet(TARGET_URL).execute(new AsyncCompletionHandler<Response>() {
*
* @Override
* public Response onCompleted(Response response) throws IOException {
* // Do something
* return response;
* }
*
* @Override
* public void onThrowable(Throwable t) {
* }
* });
* Response response = f.get();
*
* // We are just interested to retrieve the status code.
* Future<Integer> f = c.prepareGet(TARGET_URL).execute(new AsyncCompletionHandler<Integer>() {
*
* @Override
* public Integer onCompleted(Response response) throws IOException {
* // Do something
* return response.getStatusCode();
* }
*
* @Override
* public void onThrowable(Throwable t) {
* }
* });
* Integer statusCode = f.get();
*
* The {@link AsyncCompletionHandler#onCompleted(Response)} will be invoked once the http response has been fully read, which include
* the http headers and the response body. Note that the entire response will be buffered in memory.
*
* You can also have more control about the how the response is asynchronously processed by using a {@link AsyncHandler}
*
* AsyncHttpClient c = new AsyncHttpClient();
* Future<String> f = c.prepareGet(TARGET_URL).execute(new AsyncHandler<String>() {
* private StringBuilder builder = new StringBuilder();
*
* @Override
* public STATE onStatusReceived(HttpResponseStatus s) throws Exception {
* // return STATE.CONTINUE or STATE.ABORT
* return STATE.CONTINUE
* }
*
* @Override
* public STATE onHeadersReceived(HttpResponseHeaders bodyPart) throws Exception {
* // return STATE.CONTINUE or STATE.ABORT
* return STATE.CONTINUE
*
* }
* @Override
*
* public STATE onBodyPartReceived(HttpResponseBodyPart bodyPart) throws Exception {
* builder.append(new String(bodyPart));
* // return STATE.CONTINUE or STATE.ABORT
* return STATE.CONTINUE
* }
*
* @Override
* public String onCompleted() throws Exception {
* // Will be invoked once the response has been fully read or a ResponseComplete exception
* // has been thrown.
* return builder.toString();
* }
*
* @Override
* public void onThrowable(Throwable t) {
* }
* });
*
* String bodyResponse = f.get();
*
* You can asynchronously process the response status,headers and body and decide when to
* stop the processing the response by returning a new {@link AsyncHandler.State#ABORT} at any moment.
*
* This class can also be used without the need of {@link AsyncHandler}.
*
*
* AsyncHttpClient c = new AsyncHttpClient();
* Future<Response> f = c.prepareGet(TARGET_URL).execute();
* Response r = f.get();
*
*
* Finally, you can configure the AsyncHttpClient using an {@link DefaultAsyncHttpClientConfig} instance.
*
*
* AsyncHttpClient c = new AsyncHttpClient(new AsyncHttpClientConfig.Builder().setRequestTimeoutInMs(...).build());
* Future<Response> f = c.prepareGet(TARGET_URL).execute();
* Response r = f.get();
*
*
* An instance of this class will cache every HTTP 1.1 connections and close them when the {@link DefaultAsyncHttpClientConfig#getReadTimeout()}
* expires. This object can hold many persistent connections to different host.
*/
public interface AsyncHttpClient extends Closeable {
/**
* Return true if closed
*
* @return true if closed
*/
boolean isClosed();
/**
* Set default signature calculator to use for requests build by this client instance
*
* @param signatureCalculator a signature calculator
* @return {@link RequestBuilder}
*/
AsyncHttpClient setSignatureCalculator(SignatureCalculator signatureCalculator);
/**
* Prepare an HTTP client request.
*
* @param method HTTP request method type. MUST BE in upper case
* @param url A well formed URL.
* @return {@link RequestBuilder}
*/
BoundRequestBuilder prepare(String method, String url);
/**
* Prepare an HTTP client GET request.
*
* @param url A well formed URL.
* @return {@link RequestBuilder}
*/
BoundRequestBuilder prepareGet(String url);
/**
* Prepare an HTTP client CONNECT request.
*
* @param url A well formed URL.
* @return {@link RequestBuilder}
*/
BoundRequestBuilder prepareConnect(String url);
/**
* Prepare an HTTP client OPTIONS request.
*
* @param url A well formed URL.
* @return {@link RequestBuilder}
*/
BoundRequestBuilder prepareOptions(String url);
/**
* Prepare an HTTP client HEAD request.
*
* @param url A well formed URL.
* @return {@link RequestBuilder}
*/
BoundRequestBuilder prepareHead(String url);
/**
* Prepare an HTTP client POST request.
*
* @param url A well formed URL.
* @return {@link RequestBuilder}
*/
BoundRequestBuilder preparePost(String url);
/**
* Prepare an HTTP client PUT request.
*
* @param url A well formed URL.
* @return {@link RequestBuilder}
*/
BoundRequestBuilder preparePut(String url);
/**
* Prepare an HTTP client DELETE request.
*
* @param url A well formed URL.
* @return {@link RequestBuilder}
*/
BoundRequestBuilder prepareDelete(String url);
/**
* Prepare an HTTP client PATCH request.
*
* @param url A well formed URL.
* @return {@link RequestBuilder}
*/
BoundRequestBuilder preparePatch(String url);
/**
* Prepare an HTTP client TRACE request.
*
* @param url A well formed URL.
* @return {@link RequestBuilder}
*/
BoundRequestBuilder prepareTrace(String url);
/**
* Construct a {@link RequestBuilder} using a {@link Request}
*
* @param request a {@link Request}
* @return {@link RequestBuilder}
*/
BoundRequestBuilder prepareRequest(Request request);
/**
* Construct a {@link RequestBuilder} using a {@link RequestBuilder}
*
* @param requestBuilder a {@link RequestBuilder}
* @return {@link RequestBuilder}
*/
BoundRequestBuilder prepareRequest(RequestBuilder requestBuilder);
/**
* Execute an HTTP request.
*
* @param request {@link Request}
* @param handler an instance of {@link AsyncHandler}
* @param Type of the value that will be returned by the associated {@link java.util.concurrent.Future}
* @return a {@link Future} of type T
*/
ListenableFuture executeRequest(Request request, AsyncHandler handler);
/**
* Execute an HTTP request.
*
* @param requestBuilder {@link RequestBuilder}
* @param handler an instance of {@link AsyncHandler}
* @param Type of the value that will be returned by the associated {@link java.util.concurrent.Future}
* @return a {@link Future} of type T
*/
ListenableFuture executeRequest(RequestBuilder requestBuilder, AsyncHandler handler);
/**
* Execute an HTTP request.
*
* @param request {@link Request}
* @return a {@link Future} of type Response
*/
ListenableFuture executeRequest(Request request);
/**
* Execute an HTTP request.
*
* @param requestBuilder {@link RequestBuilder}
* @return a {@link Future} of type Response
*/
ListenableFuture executeRequest(RequestBuilder requestBuilder);
/***
* Return details about pooled connections.
*
* @return a {@link ClientStats}
*/
ClientStats getClientStats();
/**
* Flush ChannelPool partitions based on a predicate
*
* @param predicate the predicate
*/
void flushChannelPoolPartitions(Predicate
© 2015 - 2024 Weber Informatics LLC | Privacy Policy