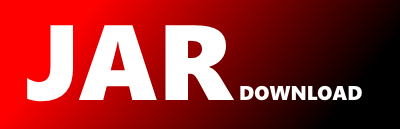
org.asynchttpclient.netty.channel.ConnectionSemaphore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of async-http-client Show documentation
Show all versions of async-http-client Show documentation
The Async Http Client (AHC) classes.
/*
* Copyright (c) 2017 AsyncHttpClient Project. All rights reserved.
*
* This program is licensed to you under the Apache License Version 2.0,
* and you may not use this file except in compliance with the Apache License Version 2.0.
* You may obtain a copy of the Apache License Version 2.0 at
* http://www.apache.org/licenses/LICENSE-2.0.
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the Apache License Version 2.0 is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the Apache License Version 2.0 for the specific language governing permissions and limitations there under.
*/
package org.asynchttpclient.netty.channel;
import org.asynchttpclient.AsyncHttpClientConfig;
import org.asynchttpclient.exception.TooManyConnectionsException;
import org.asynchttpclient.exception.TooManyConnectionsPerHostException;
import java.io.IOException;
import java.util.concurrent.ConcurrentHashMap;
import static org.asynchttpclient.util.ThrowableUtil.unknownStackTrace;
/**
* Max connections and max-per-host connections limiter.
*
* @author Stepan Koltsov
*/
public class ConnectionSemaphore {
private final NonBlockingSemaphoreLike freeChannels;
private final int maxConnectionsPerHost;
private final ConcurrentHashMap
© 2015 - 2024 Weber Informatics LLC | Privacy Policy