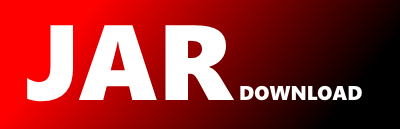
org.atmosphere.cdi.CDIObjectFactory Maven / Gradle / Ivy
/*
* Copyright 2015 Async-IO.org
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package org.atmosphere.cdi;
import org.atmosphere.cpr.AtmosphereConfig;
import org.atmosphere.cpr.AtmosphereObjectFactory;
import org.atmosphere.inject.AtmosphereConfigAware;
import org.atmosphere.inject.CDIProducer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.enterprise.context.spi.CreationalContext;
import javax.enterprise.inject.spi.Bean;
import javax.enterprise.inject.spi.BeanManager;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import java.util.Iterator;
import java.util.ServiceLoader;
/**
* CDI support for injecting object
*
* @author Jeanfrancois Arcand
*/
public class CDIObjectFactory implements AtmosphereObjectFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy