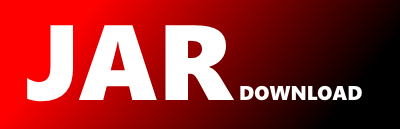
org.avaje.config.AppProperties Maven / Gradle / Ivy
The newest version!
package org.avaje.config;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
import java.util.Set;
import javax.servlet.ServletContext;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Provides access to properties loaded from the application.properties file.
*
* Allows for loading of external properties by specifying a load.properties
* entry in the properties file that references a file typically using an
* environment variable.
*
*/
public final class AppProperties {
private static final Logger log = LoggerFactory.getLogger(AppProperties.class);
private static PropertyMap globalMap;
private static boolean initialised;
/**
* Ensure the properties are initialised.
*/
public static synchronized void init(ServletContext sc) {
if (initialised) {
log.warn("properties already initialised");
} else {
initPropertyMap(sc);
initialised = true;
}
}
/**
* Return the subset of properties that start with the given prefix.
*/
public static Properties startingWith(String propertyPrefix) {
Properties subset = new Properties();
for (Entry entry : getPropertyMap().entrySet()) {
if (entry.getKey().startsWith(propertyPrefix)) {
subset.put(entry.getKey(), entry.getValue());
}
}
return subset;
}
/**
* Parse the string replacing any expressions like ${catalina.base}.
*
* This will evaluate expressions using first environment variables, than java
* system properties and lastly local properties - in that order.
*
*
* Expressions start with "${" and end with "}".
*
*/
public static String eval(String val) {
return getPropertyMap().eval(val);
}
/**
* Return as a traditional Properties object.
*/
public static Properties asProperties() {
return getPropertyMap().asProperties();
}
private static void initPropertyMap(ServletContext sc) {
String fileName = System.getenv("APP_PROPS");
if (fileName == null) {
fileName = System.getProperty("app.props");
if (fileName == null) {
fileName = "application.properties";
}
}
PropertyMap map = new PropertyMap();
if (sc == null) {
log.info("initialising without servletContext, setting webContextPath to empty string");
map.put("webContextPath", "");
} else {
String contextPath = sc.getContextPath();
map.put("contextPath", contextPath);
log.info("initialising with servletContext, contextPath:[{}]", contextPath);
if ("".equals(contextPath)) {
contextPath = "root";
}
contextPath = contextPath.replace("/", "");
log.info("setting webContextPath={}", contextPath);
map.put("webContextPath", contextPath);
}
globalMap = PropertyMapLoader.load(map, fileName);
if (globalMap == null) {
globalMap = new PropertyMap();
}
if (globalMap.getBoolean("loadIntoSystemProperties", false)) {
log.info("loading AppProperties into SystemProperties ...");
// Load each property into SystemProperties
Set> entrySet = globalMap.entrySet();
for (Entry entry : entrySet) {
System.setProperty(entry.getKey(), entry.getValue());
}
}
}
/**
* Return the property map loading it if required.
*/
private static synchronized PropertyMap getPropertyMap() {
if (globalMap == null) {
initPropertyMap(null);
}
return globalMap;
}
/**
* Return a String property.
*/
public static synchronized String get(String key) {
return getPropertyMap().get(key, null);
}
/**
* Return a String property with a default value.
*/
public static synchronized String get(String key, String defaultValue) {
return getPropertyMap().get(key, defaultValue);
}
/**
* Return a int property with a default value.
*/
public static synchronized int getInt(String key, int defaultValue) {
return getPropertyMap().getInt(key, defaultValue);
}
/**
* Return a boolean property with a default value.
*/
public static synchronized boolean getBoolean(String key, boolean defaultValue) {
return getPropertyMap().getBoolean(key, defaultValue);
}
/**
* Set a property return the previous value. This will evaluate any
* expressions in the value.
*/
public static synchronized String put(String key, String value) {
return getPropertyMap().putEval(key, value);
}
/**
* Set a Map of key value properties.
*/
public static synchronized void putAll(Map keyValueMap) {
for (Entry e : keyValueMap.entrySet()) {
getPropertyMap().putEval(e.getKey(), e.getValue());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy