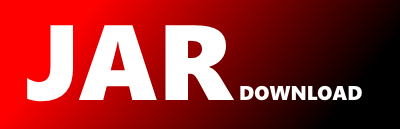
com.avaje.ebeaninternal.server.ldap.expression.LdJunctionExpression Maven / Gradle / Ivy
package com.avaje.ebeaninternal.server.ldap.expression;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.avaje.ebean.Expression;
import com.avaje.ebean.ExpressionFactory;
import com.avaje.ebean.ExpressionList;
import com.avaje.ebean.FutureIds;
import com.avaje.ebean.FutureList;
import com.avaje.ebean.FutureRowCount;
import com.avaje.ebean.Junction;
import com.avaje.ebean.OrderBy;
import com.avaje.ebean.PagingList;
import com.avaje.ebean.QueryIterator;
import com.avaje.ebean.QueryListener;
import com.avaje.ebean.QueryResultVisitor;
import com.avaje.ebean.event.BeanQueryRequest;
import com.avaje.ebeaninternal.api.ManyWhereJoins;
import com.avaje.ebeaninternal.api.SpiExpression;
import com.avaje.ebeaninternal.api.SpiExpressionRequest;
import com.avaje.ebeaninternal.api.SpiLuceneExpr;
import com.avaje.ebeaninternal.server.deploy.BeanDescriptor;
import com.avaje.ebeaninternal.server.query.LuceneResolvableRequest;
import com.avaje.ebeaninternal.util.DefaultExpressionList;
/**
* Junction implementation.
*/
abstract class LdJunctionExpression implements Junction, SpiExpression {
private static final long serialVersionUID = -7422204102750462677L;
static class Conjunction extends LdJunctionExpression {
private static final long serialVersionUID = -645619859900030679L;
Conjunction(com.avaje.ebean.Query query, ExpressionList parent){
super("&",query, parent);
}
Conjunction(ExpressionFactory exprFactory) {
super("&", exprFactory);
}
}
static class Disjunction extends LdJunctionExpression {
private static final long serialVersionUID = -8464470066692221414L;
Disjunction(com.avaje.ebean.Query query, ExpressionList parent){
super("|",query, parent);
}
Disjunction(ExpressionFactory exprFactory) {
super("|", exprFactory);
}
}
private final DefaultExpressionList exprList;
private final String joinType;
LdJunctionExpression(String joinType, com.avaje.ebean.Query query, ExpressionList parent) {
this.joinType = joinType;
this.exprList = new DefaultExpressionList(query, parent);
}
LdJunctionExpression(String joinType, ExpressionFactory exprFactory) {
this.joinType = joinType;
this.exprList = new DefaultExpressionList(null, exprFactory, null);
}
public boolean isLuceneResolvable(LuceneResolvableRequest req) {
return false;
}
public SpiLuceneExpr createLuceneExpr(SpiExpressionRequest request) {
return null;
}
public void containsMany(BeanDescriptor> desc, ManyWhereJoins manyWhereJoin) {
List list = exprList.internalList();
for (int i = 0; i < list.size(); i++) {
list.get(i).containsMany(desc, manyWhereJoin);
}
}
public Junction add(Expression item){
SpiExpression i = (SpiExpression)item;
exprList.add(i);
return this;
}
public void addBindValues(SpiExpressionRequest request) {
List list = exprList.internalList();
for (int i = 0; i < list.size(); i++) {
SpiExpression item = list.get(i);
item.addBindValues(request);
}
}
public void addSql(SpiExpressionRequest request) {
List list = exprList.internalList();
if (!list.isEmpty()){
request.append("(");
request.append(joinType);
for (int i = 0; i < list.size(); i++) {
SpiExpression item = list.get(i);
item.addSql(request);
}
request.append(") ");
}
}
/**
* Based on Junction type and all the expression contained.
*/
public int queryAutoFetchHash() {
int hc = LdJunctionExpression.class.getName().hashCode();
hc = hc * 31 + joinType.hashCode();
List list = exprList.internalList();
for (int i = 0; i < list.size(); i++) {
hc = hc * 31 + list.get(i).queryAutoFetchHash();
}
return hc;
}
public int queryPlanHash(BeanQueryRequest> request) {
int hc = LdJunctionExpression.class.getName().hashCode();
hc = hc * 31 + joinType.hashCode();
List list = exprList.internalList();
for (int i = 0; i < list.size(); i++) {
hc = hc * 31 + list.get(i).queryPlanHash(request);
}
return hc;
}
public int queryBindHash() {
int hc = LdJunctionExpression.class.getName().hashCode();
List list = exprList.internalList();
for (int i = 0; i < list.size(); i++) {
hc = hc * 31 + list.get(i).queryBindHash();
}
return hc;
}
public ExpressionList endJunction() {
return exprList.endJunction();
}
public ExpressionList allEq(Map propertyMap) {
return exprList.allEq(propertyMap);
}
public ExpressionList and(Expression expOne, Expression expTwo) {
return exprList.and(expOne, expTwo);
}
public ExpressionList between(String propertyName, Object value1, Object value2) {
return exprList.between(propertyName, value1, value2);
}
public ExpressionList betweenProperties(String lowProperty, String highProperty, Object value) {
return exprList.betweenProperties(lowProperty, highProperty, value);
}
public Junction conjunction() {
return exprList.conjunction();
}
public ExpressionList contains(String propertyName, String value) {
return exprList.contains(propertyName, value);
}
public Junction disjunction() {
return exprList.disjunction();
}
public ExpressionList endsWith(String propertyName, String value) {
return exprList.endsWith(propertyName, value);
}
public ExpressionList eq(String propertyName, Object value) {
return exprList.eq(propertyName, value);
}
public ExpressionList exampleLike(Object example) {
return exprList.exampleLike(example);
}
public ExpressionList filterMany(String prop) {
throw new RuntimeException("filterMany not allowed on Junction expression list");
}
public FutureIds findFutureIds() {
return exprList.findFutureIds();
}
public FutureList findFutureList() {
return exprList.findFutureList();
}
public FutureRowCount findFutureRowCount() {
return exprList.findFutureRowCount();
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy