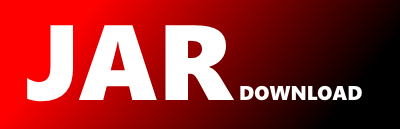
com.avaje.ebeaninternal.server.deploy.parse.DeployInheritInfo Maven / Gradle / Ivy
/**
* Copyright (C) 2006 Robin Bygrave
*
* This file is part of Ebean.
*
* Ebean is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; either version 2.1 of the License, or
* (at your option) any later version.
*
* Ebean is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Ebean; if not, write to the Free Software Foundation, Inc.,
* 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*/
package com.avaje.ebeaninternal.server.deploy.parse;
import java.sql.Types;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import com.avaje.ebeaninternal.server.deploy.InheritInfo;
/**
* Represents a node in the Inheritance tree.
* Holds information regarding Super Subclass support.
*/
public class DeployInheritInfo {
/**
* the default discriminator column according to the JPA 1.0 spec.
*/
private static final String JPA_DEFAULT_DISCRIM_COLUMN = "dtype";
private int discriminatorLength;
private int discriminatorType;
private String discriminatorStringValue;
private Object discriminatorObjectValue;
private String discriminatorColumn;
private String discriminatorWhere;
private Class> type;
private Class> parent;
private ArrayList children = new ArrayList();
/**
* Create for a given type.
*/
public DeployInheritInfo(Class> type){
this.type = type;
}
/**
* return the type.
*/
public Class> getType() {
return type;
}
/**
* Return the type of the root object.
*/
public Class> getParent() {
return parent;
}
/**
* Set the type of the root object.
*/
public void setParent(Class> parent) {
this.parent = parent;
}
/**
* Return true if this is abstract node.
*/
public boolean isAbstract() {
return (discriminatorObjectValue == null);
}
/**
* Return true if this is the root node.
*/
public boolean isRoot(){
return parent == null;
}
/**
* Return the child nodes.
*/
public Iterator children() {
return children.iterator();
}
/**
* Add a child node.
*/
public void addChild(DeployInheritInfo childInfo){
children.add(childInfo);
}
/**
* Return the derived where for the discriminator.
*/
public String getDiscriminatorWhere() {
return discriminatorWhere;
}
/**
* Set the derived where for the discriminator.
*/
public void setDiscriminatorWhere(String discriminatorWhere) {
this.discriminatorWhere = discriminatorWhere;
}
/**
* Return the column name of the discriminator.
*/
public String getDiscriminatorColumn(InheritInfo parent) {
if (discriminatorColumn == null){
if (parent == null){
discriminatorColumn = JPA_DEFAULT_DISCRIM_COLUMN;
} else {
discriminatorColumn = parent.getDiscriminatorColumn();
}
}
return discriminatorColumn;
}
/**
* Set the column name of the discriminator.
*/
public void setDiscriminatorColumn(String discriminatorColumn) {
this.discriminatorColumn = discriminatorColumn;
}
public int getDiscriminatorLength(InheritInfo parent) {
if (discriminatorLength == 0){
if (parent == null){
discriminatorLength = 10;
} else {
discriminatorLength = parent.getDiscriminatorLength();
}
}
return discriminatorLength;
}
/**
* Return the sql type of the discriminator value.
*/
public int getDiscriminatorType(InheritInfo parent) {
if (discriminatorType == 0){
if (parent == null){
discriminatorType = Types.VARCHAR;
} else {
discriminatorType = parent.getDiscriminatorType();
}
}
return discriminatorType;
}
/**
* Set the sql type of the discriminator.
*/
public void setDiscriminatorType(int discriminatorType) {
this.discriminatorType = discriminatorType;
}
/**
* Return the length of the discriminator column.
*/
public int getDiscriminatorLength() {
return discriminatorLength;
}
/**
* Set the length of the discriminator column.
*/
public void setDiscriminatorLength(int discriminatorLength) {
this.discriminatorLength = discriminatorLength;
}
/**
* Return the discriminator value for this node.
*/
public Object getDiscriminatorObjectValue() {
return discriminatorObjectValue;
}
public String getDiscriminatorStringValue() {
return discriminatorStringValue;
}
/**
* Set the discriminator value for this node.
*/
public void setDiscriminatorValue(String value) {
if (value != null){
value = value.trim();
if (value.length() == 0){
value = null;
} else {
discriminatorStringValue = value;
// convert the value if desired
if (discriminatorType == Types.INTEGER){
this.discriminatorObjectValue = Integer.valueOf(value.toString());
} else {
this.discriminatorObjectValue = value;
}
}
}
}
public String getWhere() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy