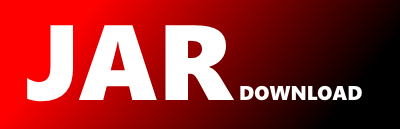
org.example.JunkApi Maven / Gradle / Ivy
package org.example;
import io.avaje.http.api.*;
import io.avaje.http.client.HttpCall;
import java.io.InputStream;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.nio.file.Path;
import java.util.List;
import java.util.UUID;
import java.util.concurrent.CompletableFuture;
import java.util.stream.Stream;
@Client
public interface JunkApi {
@Post
void asVoid();
@Post
HttpResponse asVoid2();
@Post
String asPlainString();
@Post
HttpResponse asString2();
// @Post byte[] asBytesErr();
@Post
HttpResponse asBytes2();
// @Post InputStream asInputStreamErr();
@Post
HttpResponse asInputStream2();
// @Post Stream asLinesErr();
@Post
HttpResponse> asLines2();
@Post
Repo bean();
@Post
List list();
@Post
Stream stream();
@Post
CompletableFuture cfBean();
@Post
CompletableFuture> cfList();
@Post
CompletableFuture> cfStream();
@Post
HttpCall callBean();
@Post
HttpCall> callList();
@Post
HttpCall> callStream();
// -------
// @Post CompletableFuture cfVoidErr();
@Post
CompletableFuture> cfVoid();
// @Post CompletableFuture cfStringErr();
@Post
CompletableFuture> cfString();
// @Post CompletableFuture cfBytesErr();
@Post
CompletableFuture> cfBytes();
// @Post CompletableFuture cfInputStreamErr2();
@Post
CompletableFuture> cfInputStream();
// @Post CompletableFuture> cfLinesErr();
@Post
CompletableFuture>> cfLines();
// @Post CompletableFuture cfVoidErr();
@Post
HttpCall> callVoid();
// @Post CompletableFuture cfStringErr();
@Post
HttpCall> callString();
// @Post HttpCall callBytesErr();
@Post
HttpCall> callBytes();
// @Post HttpCall callInputStreamErr();
@Post
HttpCall> callInputStream();
// @Post HttpCall> callLinesErr();
@Post
HttpCall>> callLines();
@Get
HttpResponse withHandGeneric(HttpResponse.BodyHandler handler);
@Get
HttpResponse withHandPath(HttpResponse.BodyHandler handler);
@Get
CompletableFuture> cfWithHandGeneric(HttpResponse.BodyHandler handler);
@Get
CompletableFuture> cfWithHandPath(HttpResponse.BodyHandler handler);
@Get
HttpCall> callWithHandGeneric(HttpResponse.BodyHandler handler);
@Get
HttpCall> callWithHandPath(HttpResponse.BodyHandler handler);
@Post("/{id}/foo/{name}")
HttpResponse postWithBody(String id, String name, HttpRequest.BodyPublisher body, String other);
@Get("/{id}")
HttpResponse getWithHandler(String id, HttpResponse.BodyHandler myHandler, String other);
@Get("/{id}")
HttpResponse getWithGeneralHandler(String id, HttpResponse.BodyHandler myHandler);
@Post("/{id}/foo/{name}")
HttpResponse reqBodyResHand2(HttpResponse.BodyHandler handler, HttpRequest.BodyPublisher body, String id, String name, String other);
@Form
@Post("foo/{email}")
void postFormWithPath(String email, String name, String other);
@Post("withBeanParam/{id}")
void postWithBeanParam(UUID id, @BeanParam CommonParams commonParams);
@Form @Post("withFormParam/{id}")
void postWithFormParam(UUID id, MyForm theForm, @BeanParam CommonParams commonParams);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy