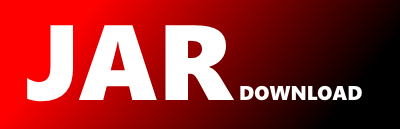
org.kawanfw.commons.api.server.util.ServerInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file-server Show documentation
Show all versions of awake-file-server Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
The newest version!
/*
* This file is part of Awake FILE.
* Awake file: Easy file upload & download over HTTP with Java.
* Copyright (C) 2015, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.kawanfw.commons.api.server.util;
import java.net.InetAddress;
import java.net.NetworkInterface;
import javax.servlet.http.HttpServletRequest;
import org.kawanfw.file.servlet.RequestInfoStore;
/**
*
* Gets some server info, including info extracted from HttpServletRequest
at server startup.
* Includes:
*
* - Computer Hostname.
* - Computer MAC Address.
* - Computer IP Address.
* - Server URL (without the port) in
http(s)://www.acme.org
format.
* - Server scheme.
* - Server port.
*
*
* @author Nicolas de Pomereu.
*/
public class ServerInfo {
public static final String UNKNOWN_HOSTNAME = "unknown_hostname";
public static final String UNKNOWN_IP_ADDRESS = "unknown_ip_address";
public static final String UNKNOWN_MAC_ADDRESS = "unknown_mac_address";
/** The hostname */
private static String hostName = null;
/** The MAC address */
private static String macAddress = null;
/** The IP address */
private static String ipAddress = null;
/**
* Protected
*/
protected ServerInfo() {
}
/**
* Returns the computer name (Hostname).
*
* @return the name or unknown_hostname
if the name cannot be found
*/
public static String getHostname() {
try {
if (hostName == null) {
final InetAddress addr = InetAddress.getLocalHost();
hostName = new String(addr.getHostName());
if (hostName == null) {
hostName = UNKNOWN_HOSTNAME;
}
}
} catch (final Exception e) {
hostName = UNKNOWN_HOSTNAME;
e.printStackTrace(System.out);
}
return hostName;
}
/**
* Returns the computer IP address in 192.168.1.146 format.
*
* @return the name or unknown_ip_address
if the IP address cannot
* be found
*/
public static String getIpAddress() {
try {
if (ipAddress == null) {
InetAddress ip = InetAddress.getLocalHost();
ipAddress = ip.getHostAddress();
}
} catch (Exception e) {
ipAddress = UNKNOWN_IP_ADDRESS;
e.printStackTrace(System.out);
}
return ipAddress;
}
/**
* Returns the computer MAC address in 5C-26-0A-88-4E-DA format.
*
* @return the name or unknown_mac_address
if the MAC address cannot
* be found
*/
public static String getMacAddress() {
try {
if (macAddress == null) {
InetAddress ip = InetAddress.getLocalHost();
NetworkInterface network = NetworkInterface
.getByInetAddress(ip);
if (network == null) {
macAddress = UNKNOWN_MAC_ADDRESS;
return macAddress;
}
byte[] mac = network.getHardwareAddress();
if (mac == null) {
macAddress = UNKNOWN_MAC_ADDRESS;
return macAddress;
}
StringBuilder sb = new StringBuilder();
for (int i = 0; i < mac.length; i++) {
sb.append(String.format("%02X%s", mac[i],
(i < mac.length - 1) ? "-" : ""));
}
macAddress = sb.toString();
}
} catch (Exception e) {
macAddress = UNKNOWN_MAC_ADDRESS;
e.printStackTrace(System.out);
}
return macAddress;
}
/**
* Server URL (without the port) in http(s)://www.acme.org
format.
*
* Info extracted from HttpServletRequest
at each request
*
* @return Server URL (without the port)
*/
public static String getServerUrl() {
HttpServletRequest httpServletRequest = RequestInfoStore.getHttpServletRequest();
String serverName = httpServletRequest.getServerName();
String scheme = httpServletRequest.getScheme();
if (scheme == null || serverName == null) {
return null;
}
String hostUrl = scheme + "://" + serverName;
return hostUrl;
}
/**
* Returns the name of the scheme used to make this request, for example,
* http, https, or ftp. Different schemes have different rules for
* constructing URLs, as noted in RFC 1738.
*
* Info extracted from HttpServletRequest
at each request
*
* @return a String containing the name of the scheme used to make this
* request
*/
public static String getScheme() {
HttpServletRequest httpServletRequest = RequestInfoStore.getHttpServletRequest();
return httpServletRequest.getScheme();
}
/**
* Returns the host name of the server to which the request was sent. It is
* the value of the part before ":" in the Host header value, if any, or the
* resolved server name, or the server IP address.
*
* Info extracted from HttpServletRequest
at each request
*
* @return a String containing the name of the server
*/
public static String getServerName() {
HttpServletRequest httpServletRequest = RequestInfoStore.getHttpServletRequest();
return httpServletRequest.getServerName();
}
/**
* Returns the port number to which the request was sent. It is the value of
* the part after ":" in the Host header value, if any, or the server port
* where the client connection was accepted on.
*
* Info extracted from HttpServletRequest
at each request
*
* @return an integer specifying the port number
*/
public static int getServerPort() {
HttpServletRequest httpServletRequest = RequestInfoStore.getHttpServletRequest();
return httpServletRequest.getServerPort();
}
public static void main(String[] args) throws Exception {
System.out.println("getHostname() : " + getHostname());
System.out.println("getIpAddres() : " + getIpAddress());
System.out.println("getMacAddress(): " + getMacAddress());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy