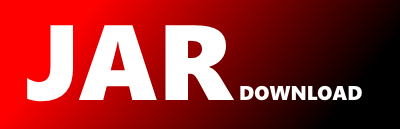
src-main.org.awakefw.commons.api.client.AwakeProgressManager Maven / Gradle / Ivy
Show all versions of awake-file Show documentation
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.commons.api.client;
import org.awakefw.file.api.client.AwakeFileSession;
/**
*
* Interface that defines methods to monitor in Swing the upload & download of
* files.
*
* An implemented instance must be passed to the {@link AwakeFileSession}
* instance using the setAwakeProgressManager
method.
*
* It is then possible to use your own methods to follow the progress or to
* cancel the task.
* See {@link DefaultAwakeProgressManager} for a default and basic
* implementation that should suit (almost) all your needs.
*
* See the source code of AwakeFileProgressMonitorDemo.java
* that demonstrates the use of an AwakeProgressManager
to upload
* files on a remote Awake Server.
*
* @see DefaultAwakeProgressManager
*
* @author Nicolas de Pomereu
* @since 1.0
*/
public interface AwakeProgressManager {
/**
* Allows to define the total length in bytes of the file(s) to transfer.
* Method will be called by Awake just before the transfer operation.
*
*
* @return the number for bytes to transfer
*/
public long getLengthToTransfer();
/**
* Sets the total length of files in bytes to transfer. Should also
* reinitialize the instance values for a new usage.
*
* @param lengthToTransfer
* the total length in bytes of the file(s) to transfer
*/
public void setLengthToTransfer(long lengthToTransfer);
/**
* Returns the progress (as percentage) of the current monitored operation.
* Method is needed and will be called by an external observer (Timer,
* SwingWorker, ...) to retrieve the progress value between 0 and 100.
*
* @return the progress value as percentage value
*/
public int getProgress();
/**
* Sets the progress in percentage of the transfer operation.
*
* The method is repeatedly called by Awake during transfer operation each
* 1% of transfer done.
*
* @param progress
* The value must be between 0 and 100
*/
public void setProgress(int progress);
/**
* Method repeatedly called by Awake when uploading/downloading files.
*
* Returns true if this task was canceled before it completed
* normally.
*
*
* @return true if this task was canceled before it completed
*/
boolean isCancelled();
/**
* Cancels the current monitored operation.
*/
public void cancel();
}