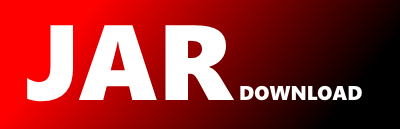
src-main.org.awakefw.commons.api.client.HttpProtocolParameters Maven / Gradle / Ivy
Show all versions of awake-file Show documentation
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.commons.api.client;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import org.awakefw.file.api.client.AwakeFileSession;
import org.awakefw.file.api.util.DefaultParms;
/**
*
* Allows to define some parameters for the Awake session:
*
* - Buffer size when uploading files. Defaults to 20480 (20 Kb).
* - Buffer size when downloading files. Defaults to 20480 (20 Kb).
* - Maximum authorized length for a string for upload or download (in order
* to avoid OutOfMemoryException on client and server side.) Defaults to 2097152
* (2 Mb).
* - Boolean to say if client sides allows HTTPS call with all SSL Certificates, including "invalid" or self-signed Certificates. defaults to
true
.
* - Password to use for encrypting all parameters request between and Host.
* - Boolean to say if Clob upload/download using character stream or ASCII
* stream must be html encoded. Defaults to
true
. (For Awake SQL
* only).
* - Upload chunk length to be used by {@link AwakeFileSession#upload(java.io.File, String)}. Defaults to 100 Mb. 0 means files are not chunked.
* - Boolean to say if client side is repeatable. Defaults to
false
.
* Note that client is always set as repeatable when using a proxy.
*
*
* Allows also to store http protocol parameters that will be passed to the
* underlying DefaultHttpClient
class of the Jakarta HttpClient 4.2 library.
*
* Use this class only if you want to change the default values of the
* HttpClient library and pass the created instance to
* AwakeFileSession
or AwakeConnection
.
*
* For example, the following change the default connection timeout to 10
* seconds and the default socket timeout to 60 seconds:
*
*
* String url = "https://www.acme.org/AwakeFileManager";
* String username = "myUsername";
* char [] password = {'m', 'y', 'P', 'a', 's', 's', 'w', 'o', 'r', 'd'};
*
* HttpProtocolParameters httpProtocolParameters = new HttpProtocolParameters();
*
* // Sets the timeout until a connection is established to 10 seconds
* httpProtocolParameters.setHttpClientParameter(
* "http.connection.timeout", new Integer(10 * 1000));
*
* // Sets the socket timeout (SO_TIMEOUT) to 60 seconds
* httpProtocolParameters.setHttpClientParameter("http.socket.timeout",
* new Integer(60 * 1000));
*
* // We will use no proxy
* HttpProxy httpProxy = null;
*
* AwakeFileSession awakeFileSession
* = new AwakeFileSession(url, username, password, httpProxy, httpProtocolParameters);
*
* // Etc.
*
*
*
* See HttpClient 4.2 Tutorial for more info on HTTP parameters.
*
* @author Nicolas de Pomereu
* @since 1.0
*/
public class HttpProtocolParameters {
/** The maximum size of a string read from input stream. Should be <= 2Mb */
private int maxLengthForString = DefaultParms.DEFAULT_MAX_LENGTH_FOR_STRING;
/** The buffer size when uploading a file */
private int uploadBufferSize = DefaultParms.DEFAULT_UPLOAD_BUFFER_SIZE;
/** Buffer size for download and copy */
private int downloadBufferSize = DefaultParms.DEFAULT_DOWNLOAD_BUFFER_SIZE;
/**
* Says if we want to html Encode the Clob when using chararacter or ASCII
* stream Default is true
*/
private boolean htmlEncodingOn = DefaultParms.DEFAULT_HTML_ENCODING_ON;
/** Says if we accept all SSL Certificates (example: self signed certificates) */
private boolean acceptAllSslCertificates = DefaultParms.ACCEPT_ALL_SSL_CERTIFICATES;
/**
* The password to use to encrypt all request parameter names and values.
* null means no encryption is done
*/
private char[] encryptionPassword = null;
/** The chunk length for {@link AwakeFileSession#upload(java.io.File, String)}. Defaults to 100 Mb. */
private long uploadChunkLength = 100 * DefaultParms.MB;
/** Says client side is always repeatable. Defaults to false */
private boolean repeatable = DefaultParms.DEFAULT_REPEATABLE;
/** Hash map of HTTP parameters that this collection contains */
private MapHttpProtocolParameters
* instance.
*
* @return a clean representation of the HttpProtocolParameters
* instance
*/
@Override
public String toString() {
return "HttpProtocolParameters [maxLengthForString="
+ this.maxLengthForString + ", uploadBufferSize="
+ this.uploadBufferSize + ", downloadBufferSize="
+ this.downloadBufferSize + ", encryptionPassword="
+ Arrays.toString(this.encryptionPassword)
+ ", htmlEncodingOn=" + this.htmlEncodingOn
+ ", httpClientParameters=" + this.httpClientParameters + "]";
}
}