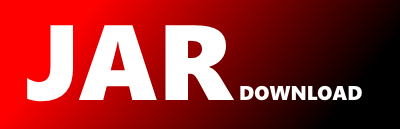
src-main.org.awakefw.commons.jdbc.abstracts.AbstractBlob Maven / Gradle / Ivy
Show all versions of awake-file Show documentation
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.commons.jdbc.abstracts;
import java.io.InputStream;
import java.io.OutputStream;
import java.sql.Blob;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
/**
* Blob Wrapper.
* Implements all the Connection methods. Usage is exactly the same as a Blob.
*/
public abstract class AbstractBlob implements Blob {
/** SQL Blob container. */
private Blob blob;
/**
* Constructor
*
* @param blob
* actual SQL/JDBC Blob in use to wrap.
*/
public AbstractBlob(Blob blob) {
this.blob = blob;
}
/**
* Returns the number of bytes in the BLOB
value designated by
* this Blob
object.
*
* @return length of the BLOB
in bytes
* @exception SQLException
* if there is an error accessing the length of the
* BLOB
* @since 1.2
*/
public long length() throws SQLException {
return this.blob.length();
}
/**
* Retrieves all or part of the BLOB
value that this
* Blob
object represents, as an array of bytes. This
* byte
array contains up to length
consecutive
* bytes starting at position pos
.
*
* @param pos
* the ordinal position of the first byte in the
* BLOB
value to be extracted; the first byte is at
* position 1
* @param length
* the number of consecutive bytes to be copied
* @return a byte array containing up to length
consecutive
* bytes from the BLOB
value designated by this
* Blob
object, starting with the byte at position
* pos
* @exception SQLException
* if there is an error accessing the BLOB
value
* @see #setBytes
* @since 1.2
*/
public byte[] getBytes(long pos, int length) throws SQLException {
return this.blob.getBytes(pos, length);
}
/**
* Retrieves the BLOB
value designated by this
* Blob
instance as a stream.
*
* @return a stream containing the BLOB
data
* @exception SQLException
* if there is an error accessing the BLOB
value
* @see #setBinaryStream
* @since 1.2
*/
public InputStream getBinaryStream() throws SQLException {
return this.blob.getBinaryStream();
}
/**
* Retrieves the byte position at which the specified byte array
* pattern
begins within the BLOB
value that this
* Blob
object represents. The search for pattern
* begins at position start
.
*
* @param pattern
* the byte array for which to search
* @param start
* the position at which to begin searching; the first position
* is 1
* @return the position at which the pattern appears, else -1
* @exception SQLException
* if there is an error accessing the BLOB
* @since 1.2
*/
public long position(byte[] pattern, long start) throws SQLException {
return this.blob.position(pattern, start);
}
/**
* Retrieves the byte position in the BLOB
value designated by
* this Blob
object at which pattern
begins. The
* search begins at position start
.
*
* @param pattern
* the Blob
object designating the BLOB
* value for which to search
* @param start
* the position in the BLOB
value at which to begin
* searching; the first position is 1
* @return the position at which the pattern begins, else -1
* @exception SQLException
* if there is an error accessing the BLOB
value
* @since 1.2
*/
public long position(Blob pattern, long start) throws SQLException {
return this.blob.position(pattern, start);
}
//
//
// -------------------------- JDBC 3.0 -----------------------------------
//
//
/**
* Writes the given array of bytes to the BLOB
value that this
* Blob
object represents, starting at position
* pos
, and returns the number of bytes written.
*
* @param pos
* the position in the BLOB
object at which to start
* writing
* @param bytes
* the array of bytes to be written to the BLOB
* value that this Blob
object represents
* @return the number of bytes written
* @exception SQLException
* if there is an error accessing the BLOB
value
* @see #getBytes
* @since 1.4
*/
public int setBytes(long pos, byte[] bytes) throws SQLException {
return this.blob.setBytes(pos, bytes);
}
/**
* Writes all or part of the given byte
array to the
* BLOB
value that this Blob
object represents and
* returns the number of bytes written. Writing starts at position
* pos
in the BLOB
value; len
bytes
* from the given byte array are written.
*
* @param pos
* the position in the BLOB
object at which to start
* writing
* @param bytes
* the array of bytes to be written to this BLOB
* object
* @param offset
* the offset into the array bytes
at which to start
* reading the bytes to be set
* @param len
* the number of bytes to be written to the BLOB
* value from the array of bytes bytes
* @return the number of bytes written
* @exception SQLException
* if there is an error accessing the BLOB
value
* @see #getBytes
* @since 1.4
*/
public int setBytes(long pos, byte[] bytes, int offset, int len)
throws SQLException {
return this.blob.setBytes(pos, bytes, offset, len);
}
/**
* Retrieves a stream that can be used to write to the BLOB
* value that this Blob
object represents. The stream begins at
* position pos
.
*
* @param pos
* the position in the BLOB
value at which to start
* writing
* @return a java.io.OutputStream
object to which data can be
* written
* @exception SQLException
* if there is an error accessing the BLOB
value
* @see #getBinaryStream
* @since 1.4
*/
public OutputStream setBinaryStream(long pos) throws SQLException {
return this.blob.setBinaryStream(pos);
}
/**
* Truncates the BLOB
value that this Blob
object
* represents to be len
bytes in length.
*
* @param len
* the length, in bytes, to which the BLOB
value
* that this Blob
object represents should be
* truncated
* @exception SQLException
* if there is an error accessing the BLOB
value
* @since 1.4
*/
public void truncate(long len) throws SQLException {
this.blob.truncate(len);
}
/**
* This method frees the Blob
object and releases the resources
* that it holds. The object is invalid once the free
method is
* called.
*
* After free
has been called, any attempt to invoke a method
* other than free
will result in a SQLException
* being thrown. If free
is called multiple times, the
* subsequent calls to free
are treated as a no-op.
*
*
* @throws SQLException
* if an error occurs releasing the Blob's resources
* @exception SQLFeatureNotSupportedException
* if the JDBC driver does not support this method
* @since 1.6
*/
public void free() throws SQLException {
this.blob.free();
}
/**
* Returns an InputStream
object that contains a partial
* Blob
value, starting with the byte specified by pos, which
* is length bytes in length.
*
* @param pos
* the offset to the first byte of the partial value to be
* retrieved. The first byte in the Blob
is at
* position 1
* @param length
* the length in bytes of the partial value to be retrieved
* @return InputStream
through which the partial
* Blob
value can be read.
* @throws SQLException
* if pos is less than 1 or if pos is greater than the number of
* bytes in the Blob
or if pos + length is greater
* than the number of bytes in the Blob
*
* @exception SQLFeatureNotSupportedException
* if the JDBC driver does not support this method
* @since 1.6
*/
public InputStream getBinaryStream(long pos, long length)
throws SQLException {
return this.blob.getBinaryStream(pos, length);
}
}
// EOF