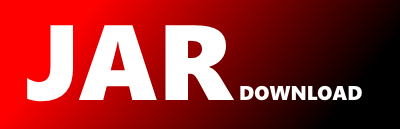
src-main.org.awakefw.commons.jdbc.abstracts.AbstractClob Maven / Gradle / Ivy
Show all versions of awake-file Show documentation
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.commons.jdbc.abstracts;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.Reader;
import java.io.Writer;
import java.sql.Clob;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
/**
* Clob Wrapper.
* Implements all the Connection methods. Usage is exactly the same as a Clob.
*/
public abstract class AbstractClob implements Clob {
/** SQL Clob container. */
private Clob clob;
/**
* Constructor
*
* @param clob
* actual SQL/JDBC Clob in use to wrap.
*/
public AbstractClob(Clob clob) {
this.clob = clob;
}
/**
* Retrieves the number of characters in the CLOB
value
* designated by this Clob
object.
*
* @return length of the CLOB
in characters
* @exception SQLException
* if there is an error accessing the length of the
* CLOB
value
* @since 1.2
*/
public long length() throws SQLException {
return this.clob.length();
}
/**
* Retrieves a copy of the specified substring in the CLOB
* value designated by this Clob
object. The substring begins
* at position pos
and has up to length
* consecutive characters.
*
* @param pos
* the first character of the substring to be extracted. The
* first character is at position 1.
* @param length
* the number of consecutive characters to be copied
* @return a String
that is the specified substring in the
* CLOB
value designated by this Clob
* object
* @exception SQLException
* if there is an error accessing the CLOB
value
* @since 1.2
*/
public String getSubString(long pos, int length) throws SQLException {
return this.clob.getSubString(pos, length);
}
/**
* Retrieves the CLOB
value designated by this
* Clob
object as a java.io.Reader
object (or as a
* stream of characters).
*
* @return a java.io.Reader
object containing the
* CLOB
data
* @exception SQLException
* if there is an error accessing the CLOB
value
* @see #setCharacterStream
* @since 1.2
*/
public Reader getCharacterStream() throws SQLException {
return this.clob.getCharacterStream();
}
/**
* Retrieves the CLOB
value designated by this
* Clob
object as an ascii stream.
*
* @return a java.io.InputStream
object containing the
* CLOB
data
* @exception SQLException
* if there is an error accessing the CLOB
value
* @see #setAsciiStream
* @since 1.2
*/
public InputStream getAsciiStream() throws SQLException {
return this.clob.getAsciiStream();
}
/**
* Retrieves the character position at which the specified substring
* searchstr
appears in the SQL CLOB
value
* represented by this Clob
object. The search begins at
* position start
.
*
* @param searchstr
* the substring for which to search
* @param start
* the position at which to begin searching; the first position
* is 1
* @return the position at which the substring appears or -1 if it is not
* present; the first position is 1
* @exception SQLException
* if there is an error accessing the CLOB
value
* @since 1.2
*/
public long position(String searchstr, long start) throws SQLException {
return this.clob.position(searchstr, start);
}
/**
* Retrieves the character position at which the specified Clob
* object searchstr
appears in this Clob
object.
* The search begins at position start
.
*
* @param searchstr
* the Clob
object for which to search
* @param start
* the position at which to begin searching; the first position
* is 1
* @return the position at which the Clob
object appears or -1
* if it is not present; the first position is 1
* @exception SQLException
* if there is an error accessing the CLOB
value
* @since 1.2
*/
public long position(Clob searchstr, long start) throws SQLException {
return this.clob.position(searchstr, start);
}
// ---------------------------- jdbc 3.0 -----------------------------------
/**
* Writes the given Java String
to the CLOB
value
* that this Clob
object designates at the position
* pos
.
*
* @param pos
* the position at which to start writing to the
* CLOB
value that this Clob
object
* represents
* @param str
* the string to be written to the CLOB
value that
* this Clob
designates
* @return the number of characters written
* @exception SQLException
* if there is an error accessing the CLOB
value
*
* @since 1.4
*/
public int setString(long pos, String str) throws SQLException {
return this.clob.setString(pos, str);
}
/**
* Writes len
characters of str
, starting at
* character offset
, to the CLOB
value that this
* Clob
represents.
*
* @param pos
* the position at which to start writing to this
* CLOB
object
* @param str
* the string to be written to the CLOB
value that
* this Clob
object represents
* @param offset
* the offset into str
to start reading the
* characters to be written
* @param len
* the number of characters to be written
* @return the number of characters written
* @exception SQLException
* if there is an error accessing the CLOB
value
*
* @since 1.4
*/
public int setString(long pos, String str, int offset, int len)
throws SQLException {
return this.clob.setString(pos, str, offset, len);
}
/**
* Retrieves a stream to be used to write Ascii characters to the
* CLOB
value that this Clob
object represents,
* starting at position pos
.
*
* @param pos
* the position at which to start writing to this
* CLOB
object
* @return the stream to which ASCII encoded characters can be written
* @exception SQLException
* if there is an error accessing the CLOB
value
* @see #getAsciiStream
*
* @since 1.4
*/
public OutputStream setAsciiStream(long pos) throws SQLException {
return this.clob.setAsciiStream(pos);
}
/**
* Retrieves a stream to be used to write a stream of Unicode characters to
* the CLOB
value that this Clob
object
* represents, at position pos
.
*
* @param pos
* the position at which to start writing to the
* CLOB
value
*
* @return a stream to which Unicode encoded characters can be written
* @exception SQLException
* if there is an error accessing the CLOB
value
* @see #getCharacterStream
*
* @since 1.4
*/
public Writer setCharacterStream(long pos) throws SQLException {
return this.clob.setCharacterStream(pos);
}
/**
* Truncates the CLOB
value that this Clob
* designates to have a length of len
characters.
*
* @param len
* the length, in bytes, to which the CLOB
value
* should be truncated
* @exception SQLException
* if there is an error accessing the CLOB
value
*
* @since 1.4
*/
public void truncate(long len) throws SQLException {
this.clob.truncate(len);
}
/**
* This method frees the Clob
object and releases the resources
* the resources that it holds. The object is invalid once the
* free
method is called.
*
* After free
has been called, any attempt to invoke a method
* other than free
will result in a SQLException
* being thrown. If free
is called multiple times, the
* subsequent calls to free
are treated as a no-op.
*
*
* @throws SQLException
* if an error occurs releasing the Clob's resources
*
* @exception SQLFeatureNotSupportedException
* if the JDBC driver does not support this method
* @since 1.6
*/
public void free() throws SQLException {
this.clob.free();
}
/**
* Returns a Reader
object that contains a partial
* Clob
value, starting with the character specified by pos,
* which is length characters in length.
*
* @param pos
* the offset to the first character of the partial value to be
* retrieved. The first character in the Clob is at position 1.
* @param length
* the length in characters of the partial value to be retrieved.
* @return Reader
through which the partial Clob
* value can be read.
* @throws SQLException
* if pos is less than 1 or if pos is greater than the number of
* characters in the Clob
or if pos + length is
* greater than the number of characters in the
* Clob
*
* @exception SQLFeatureNotSupportedException
* if the JDBC driver does not support this method
* @since 1.6
*/
public Reader getCharacterStream(long pos, long length) throws SQLException {
return this.clob.getCharacterStream(pos, length);
}
}