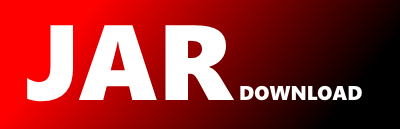
src-main.org.awakefw.commons.server.util.FileJoiner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file Show documentation
Show all versions of awake-file Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
package org.awakefw.commons.server.util;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.IOUtils;
import org.apache.commons.lang3.StringUtils;
/**
* Aas it says, class to join file that have been splitted by FileSplitter.
*
* @author Nicolas de Pomereu
*
*/
public class FileJoiner {
public static final int EOF = -1;
public static final int DEFAULT_BUFFER_SIZE = 1024 * 4;
public static final String DOT_LAST = ".LAST";
public static final String DOT_AYSNC_DOT_LAST = ".AYSNC.LAST";
/**
* Constructor
*/
public FileJoiner() {
}
/**
* Join the parts of the designated file that have been created by a previous split
*
* @param file
* the file to join from it's parts
* @throws IOException
*/
public void join(File file) throws IOException {
int countFile = 1;
int n = 0;
byte[] buffer = new byte[DEFAULT_BUFFER_SIZE];
OutputStream output = null;
InputStream input = null;
try {
output = new BufferedOutputStream(new FileOutputStream(file));
while (true) {
File filePart = new File(getFilePart(file, countFile));
if (filePart.exists()) {
try {
input = new BufferedInputStream(new FileInputStream(
filePart));
while (EOF != (n = input.read(buffer))) {
output.write(buffer, 0, n);
}
} finally {
IOUtils.closeQuietly(input);
}
countFile++;
} else {
break;
}
}
} finally {
IOUtils.closeQuietly(output);
}
}
/**
* Deletes all the parts files of the designated file
*
* @param file
* the file to delete the parts of
* @throws IOException
*/
public void deleteParts(File file) throws IOException {
int countFile = 1;
while (true) {
File filePart = new File(getFilePart(file, countFile));
if (filePart.exists()) {
FileUtils.deleteQuietly(filePart);
countFile++;
} else {
break;
}
}
}
/**
* Return the file part name from the file
*
* @param file
* the original file path
* @param countFile
* the number of the file
* @return the full file part in form /dir/filename.1.awake.sp, /dir/filename.2.awake.sp, etc...
*/
public String getFilePart(File file, int countFile) {
return file.toString() + "." + countFile + ".awake.chunk";
}
/**
* Return the file part name from the file
*
* @param filePath
* the original file path
* @param countFile
* the number of the file
* @return the full file part in form /dir/filename.1.awake.sp, /dir/filename.2.awake.sp, etc...
*/
public String getFilePart(String filename, int countFile) {
return filename + "." + countFile + ".awake.chunk";
}
/**
* Get the original file name from the file chunkc name
* @param filePart the file part name
* @return the file name with path
*/
public String getFilePathFromPart(String filePart) {
// Remove generic ext
String filePath = StringUtils.substringBeforeLast(filePart, ".awake.chunk");
// Remove file number .1 or .2, etc.
filePath = StringUtils.substringBeforeLast(filePath, ".");
return filePath;
}
/**
* Says is this file a is the fist chunk of a series of chunks
* @param filePart
* @return
*/
private boolean isFirstChunk(String filePart) {
if (filePart.endsWith(".1.awake.chunk")) {
return true;
}
else {
return false;
}
}
/**
* Delete all previous existing chunks starting at 2.
* @param filePart the chunk that may be a fist chunk
*/
public void deleteExistingChunks(String filePart) {
if (!isFirstChunk(filePart)) {
return;
}
// Delete all chunks
String rawFilename = StringUtils.substringBefore(filePart, ".1.awake.chunk");
int count = 2;
while (true) {
File chunk = new File(getFilePart(rawFilename, count));
if (chunk.exists()) {
chunk.delete();
}
else {
break;
}
count++;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy