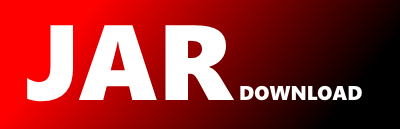
src-main.org.awakefw.file.api.client.AwakeUrl Maven / Gradle / Ivy
Show all versions of awake-file Show documentation
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.file.api.client;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.net.URL;
import java.net.UnknownHostException;
import org.awakefw.commons.api.client.AwakeProgressManager;
import org.awakefw.commons.api.client.HttpProtocolParameters;
import org.awakefw.commons.api.client.HttpProxy;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.http.HttpTransfer;
import org.awakefw.file.http.HttpTransferOne;
import org.awakefw.file.util.AwakeClientLogger;
import org.awakefw.file.version.AwakeFileVersion;
/**
* Main class for executing URL downloads.
*
* All long operations that need to be run on a separated thread may be followed
* in Swing using a Java Progress Bar or Progress Monitor: just implement the
* {@link org.awakefw.commons.api.client.AwakeProgressManager} interface.
*
*
* @see org.awakefw.commons.api.client.HttpProxy
* @see org.awakefw.commons.api.client.AwakeProgressManager
*
* @since 1.0
* @author Nicolas de Pomereu
*
*/
public class AwakeUrl {
/** For debug info */
private static boolean DEBUG = AwakeDebug.isSet(AwakeUrl.class);
/** The Http Proxy instance */
private HttpProxy httpProxy = null;
/** The http transfer instance */
private HttpTransfer httpTransfer = null;
/**
* Constructor that allows to define a proxy and protocol parameters.
*
*
* @param httpProxy
* the http proxy to use
* @param httpProtocolParameters
* the http parameters to use
*
*/
public AwakeUrl(HttpProxy httpProxy,
HttpProtocolParameters httpProtocolParameters)
throws IllegalArgumentException {
this.httpProxy = httpProxy;
httpTransfer = new HttpTransferOne(this.httpProxy,
httpProtocolParameters, null);
}
/**
* Constructor that allows to define a proxy.
*
*
* @param httpProxy
* the http proxy to use
*
*/
public AwakeUrl(HttpProxy httpProxy) {
this(httpProxy, null);
}
/**
* Constructor.
*/
public AwakeUrl() {
this(null, null);
}
/**
* Allows to set an Awake Progress Manager instance.
*
* @param awakeProgressManager
* the Awake Progress Manager instance
*/
public void setAwakeProgressManager(
AwakeProgressManager awakeProgressManager) {
httpTransfer.setAwakeProgressManager(awakeProgressManager);
}
/**
* Returns the http status code of the last executed download. Will allow to
* check, for example, if a proxy is required to access the URL.
*
* @return the http status code of the last executed download
*/
public int getHttpStatusCode() {
if (httpTransfer != null) {
return httpTransfer.getHttpStatusCode();
} else {
return 0;
}
}
/**
* Creates a File from an URL.
*
* @param url
* the URL
* @param file
* the file to create from the download.
*
* @throws IllegalArgumentException
* if url or file is null
* @throws UnknownHostException
* if Host url (http://www.acme.org) does not exists or no
* Internet Connection.
* @throws FileNotFoundException
* if it is impossible to connect to the URL.
* @throws InterruptedException
* if the download is interrupted by user through an
* AwakeProgressManager
* @throws IOException
* For all other IO / Network / System Error
*
*/
public void download(URL url, File file) throws IllegalArgumentException,
UnknownHostException, FileNotFoundException, InterruptedException,
IOException {
if (url == null) {
throw new IllegalArgumentException("url can not be null!");
}
if (file == null) {
throw new IllegalArgumentException("file can not be null!");
}
httpTransfer.downloadUrl(url, file);
}
/**
* Creates a String from an URL.
*
* @param url
* the URL
*
* @return the content of the url
*
* @throws UnknownHostException
* if Host url (http://www.acme.org) does not exists or no
* Internet Connection.
* @throws FileNotFoundException
* if it is impossible to connect to the URL.
* @throws IOException
* For all other IO / Network / System Error
*
*/
public String download(URL url) throws UnknownHostException, IOException {
if (url == null) {
throw new IllegalArgumentException("url can not be null!");
}
String content = httpTransfer.getUrlContent(url);
return content;
}
/**
* Returns the Awake FILE Version.
*
* @return the Awake FILE Version
*/
public String getVersion() {
return AwakeFileVersion.getVersion();
}
/**
* debug tool
*/
@SuppressWarnings("unused")
private void debug(String s) {
if (DEBUG) {
AwakeClientLogger.log(s);
}
}
}