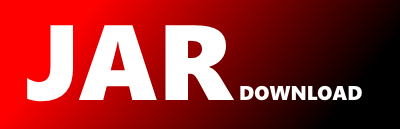
src-main.org.awakefw.file.api.util.AwakeDebug Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file Show documentation
Show all versions of awake-file Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.file.api.util;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.LineNumberReader;
import java.util.HashSet;
import java.util.Set;
import org.apache.commons.io.IOUtils;
import org.apache.commons.lang3.StringUtils;
import org.awakefw.file.util.AwakeFileUtil;
/**
* @author Nicolas de Pomereu
*
* Allow to debug files contained in user.home/.awake/awake-debug.ini.
*
*/
public class AwakeDebug {
/** The file that contain the classes to debug in user.home */
private static final String AWAKE_DEBUG_INI = "awake-debug.ini";
/** Stores the classes to debug */
private static Set CLASSES_TO_DEBUG = new HashSet();
/**
* Protected constructor
*/
protected AwakeDebug() {
}
/**
* Says if a class must be in debug mode
*
* @param clazz
* the class to analyze if debug must be on
* @return true if the class must be on debug mode, else false
*/
public static boolean isSet(Class> clazz) {
load();
String className = clazz.getName();
String rawClassName = StringUtils.substringAfterLast(className, ".");
if (CLASSES_TO_DEBUG.contains(className)
|| CLASSES_TO_DEBUG.contains(rawClassName)) {
return true;
} else {
return false;
}
}
/**
* Load the classes to debug from the file
*
* @throws IOException
*/
private static void load() {
if (!CLASSES_TO_DEBUG.isEmpty()) {
return;
}
String userHome = AwakeFileUtil.getUserHome();
if (!userHome.endsWith(File.separator)) {
userHome += File.separator;
}
File awakeDir = new File (userHome + ".awake");
awakeDir.mkdirs();
String file = awakeDir + File.separator + AWAKE_DEBUG_INI;
// Nothing to load if file not set
if (!new File(file).exists()) {
CLASSES_TO_DEBUG.add("empty");
return;
}
LineNumberReader lineNumberReader = null;
try {
lineNumberReader = new LineNumberReader(new FileReader(file));
String line = null;
while ((line = lineNumberReader.readLine()) != null) {
line = line.trim();
if (line.startsWith("//") || line.startsWith("#")
|| line.isEmpty()) {
continue;
}
CLASSES_TO_DEBUG.add(line);
}
} catch (FileNotFoundException e) {
throw new IllegalArgumentException(
"Wrapped IOException. Impossible to load debug file: "
+ file, e);
} catch (IOException e) {
throw new IllegalArgumentException(
"Wrapped IOException. Error reading debug file: " + file, e);
} finally {
IOUtils.closeQuietly(lineNumberReader);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy