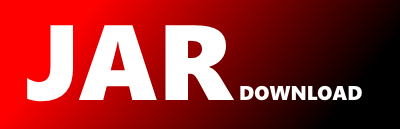
src-main.org.awakefw.file.api2.client.RemoteInputStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file Show documentation
Show all versions of awake-file Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.file.api2.client;
import java.io.BufferedReader;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.net.ConnectException;
import java.net.MalformedURLException;
import java.net.UnknownHostException;
import java.util.List;
import java.util.Vector;
import org.apache.http.message.BasicNameValuePair;
import org.awakefw.commons.api.client.HttpProtocolParameters;
import org.awakefw.commons.api.client.HttpProxy;
import org.awakefw.commons.api.client.InvalidLoginException;
import org.awakefw.commons.api.client.RemoteException;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.api.util.DefaultParms;
import org.awakefw.file.api.util.HtmlConverter;
import org.awakefw.file.http.HttpTransferOne;
import org.awakefw.file.util.AwakeClientLogger;
import org.awakefw.file.util.Tag;
import org.awakefw.file.util.TransferStatus;
import org.awakefw.file.util.parms.Action;
import org.awakefw.file.util.parms.Parameter;
/**
* @author Nicolas de Pomereu
*/
public class RemoteInputStream extends InputStream {
/** For debug info */
private static boolean DEBUG = AwakeDebug.isSet(RemoteInputStream.class);
/**
* The ServerCallerNew instance. May be reused for file transfers, per
* example
*/
private AwakeFileSessionNew awakeFileSession = null;
/** The remote file path */
private String remoteFilePath = null;
/** The entity remote stream */
private InputStream in = null;
/** The total number of bytes read */
private int totalRead = 0;
/** The beginning String */
private String beginString = "";
private boolean continueAnalasys = true;
/**
* Constructor
*
* @param awakeFileSession
* The Awake FILE session to use
* @param remoteFilePath
* the (relative) path of the file located on the remote host.
*
* @throws IllegalArgumentException
* if awakeFileSession or remoteFilePath is is null
* @throws AwakeIOException
*/
public RemoteInputStream(AwakeFileSessionNew awakeFileSession,
String remoteFilePath) throws AwakeIOException {
if (awakeFileSession == null) {
throw new IllegalArgumentException(
"awakeFileSession can not be null!");
}
if (remoteFilePath == null) {
throw new IllegalArgumentException(
"remoteFilePath can not be null!");
}
this.awakeFileSession = awakeFileSession;
this.remoteFilePath = remoteFilePath;
try {
this.buildHttpEntityInputStream();
} catch (Exception e) {
wrapExceptionAsAwakeIOException(e);
}
}
/**
* Build the InputStream from the Http Entity.
*
* @throws IOException
* @throws InterruptedException
* @throws SecurityException
* @throws UnknownHostException
* @throws IllegalArgumentException
* @throws ConnectException
* @throws Exception
* in any Exception occurs
*/
private void buildHttpEntityInputStream() throws Exception {
remoteFilePath = HtmlConverter.toHtml(remoteFilePath);
//remoteFilePath = StringUtil.toBase64(remoteFilePath);
String url = awakeFileSession.getUrl();
String username = awakeFileSession.getUsername();
String authenticationToken = awakeFileSession.getAuthenticationToken();
HttpProxy httpProxy = awakeFileSession.getHttpProxy();
HttpProtocolParameters httpProtocolParameters = awakeFileSession
.getHttpProtocolParameters();
HttpTransferOne httpTransfer = new HttpTransferOne(url, httpProxy,
httpProtocolParameters, null);
// Prepare the request parametersO
List requestParams = new Vector();
requestParams.add(new BasicNameValuePair(Parameter.ACTION,
Action.DOWNLOAD_FILE_ACTION));
requestParams.add(new BasicNameValuePair(Parameter.USERNAME, username));
requestParams.add(new BasicNameValuePair(Parameter.TOKEN,
authenticationToken));
requestParams.add(new BasicNameValuePair(Parameter.FILENAME,
remoteFilePath));
in = httpTransfer.getInputStreeam(requestParams);
if (in == null) {
throw new IOException(Tag.AWAKE_PRODUCT_FAIL
+ "The remote input stream is null!");
}
}
/**
*
* @param b
* @param off
* @param len
* @return
* @throws IOException
* @see java.io.InputStream#read(byte[], int, int)
*/
public int read(byte[] b, int off, int len) throws IOException {
int intRead = this.in.read(b, off, len);
totalRead += intRead;
if (continueAnalasys) {
try {
analyseInputStreamStart(b);
} catch (Exception e) {
wrapExceptionAsAwakeIOException(e);
}
}
return intRead;
}
/**
* @param b
* @throws IOException
*/
private void analyseInputStreamStart(byte[] b) throws Exception {
int len;
byte[] bytes = new byte[b.length];
for (int i = 0; i < b.length; i++) {
bytes[i] = b[i];
}
String string = new String(bytes);
beginString += string;
debug("beginString: " + beginString);
if (totalRead > TransferStatus.SEND_FAILED.length()) {
if (beginString.startsWith(TransferStatus.SEND_FAILED)) {
int uploadBufferSize = DefaultParms.DEFAULT_DOWNLOAD_BUFFER_SIZE;
// Get all content into a string and throw an analyzed exception
// Exception
byte[] buf = new byte[uploadBufferSize];
ByteArrayOutputStream baos = new ByteArrayOutputStream();
while ((len = this.in.read(buf)) > 0) {
baos.write(buf, 0, len);
}
close();
String content = beginString + new String(baos.toByteArray());
baos.close();
StringReader stringReader = new StringReader(content);
HttpTransferOne.throwTheRemoteException(new BufferedReader(
stringReader));
} else {
continueAnalasys = false;
}
}
}
/**
* @throws IOException
* @see java.io.InputStream#close()
*/
public void close() throws IOException {
this.in.close();
}
/**
* Throws back the trapped Exception throw by HttpTransfer as IOException
*
* @param e
* the Exception thrown by HttpTransfer call
*
* @throws AwakeIOException
* the wrapped thrown Exception:
*/
private void wrapExceptionAsAwakeIOException(Exception e)
throws AwakeIOException {
if (e instanceof UnknownHostException) {
UnknownHostException wrappedException = (UnknownHostException) e;
throw new AwakeIOException(wrappedException.getMessage(),
wrappedException);
} else if (e instanceof ConnectException) {
ConnectException wrappedException = (ConnectException) e;
throw new AwakeIOException(wrappedException.getMessage(),
wrappedException);
} else if (e instanceof MalformedURLException) {
MalformedURLException wrappedException = (MalformedURLException) e;
throw new AwakeIOException(wrappedException.getMessage(),
wrappedException);
} else if (e instanceof InvalidLoginException) {
throw new AwakeIOException(e);
} else if (e instanceof RemoteException) // Must be done before
// IOException
{
RemoteException castException = (RemoteException) e;
throw new AwakeIOException(castException.getMessage(),
castException.getCause(), // we must get the underlying sql
// cause
castException.getRemoteStackTrace());
} else if (e instanceof IOException) // IOException thrown by local code
{
IOException wrappedException = (IOException) e;
throw new AwakeIOException(wrappedException.getMessage(),
wrappedException);
} else {
throw new AwakeIOException(e);
}
}
/**
* @param b
* @return
* @throws IOException
* @see java.io.InputStream#read(byte[])
*/
public int read(byte[] b) throws IOException {
return this.read(b, 0, b.length);
}
/**
* @param n
* @return
* @throws IOException
* @see java.io.InputStream#skip(long)
*/
public long skip(long n) throws IOException {
return this.in.skip(n);
}
/**
* @return
* @throws IOException
* @see java.io.InputStream#available()
*/
public int available() throws IOException {
return this.in.available();
}
/**
* @param readlimit
* @see java.io.InputStream#mark(int)
*/
public void mark(int readlimit) {
this.in.mark(readlimit);
}
/**
* @throws IOException
* @see java.io.InputStream#reset()
*/
public void reset() throws IOException {
this.in.reset();
}
/**
* @return
* @see java.io.InputStream#markSupported()
*/
public boolean markSupported() {
return this.in.markSupported();
}
@Override
public int read() throws IOException {
// return this.in.read();
throw new IllegalStateException(
"read() method not implemented in this Awake FILE version.");
}
/**
* debug tool
*/
private void debug(String s) {
if (DEBUG) {
AwakeClientLogger.log(s);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy