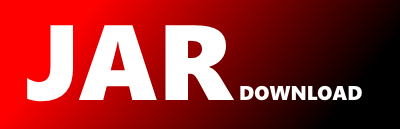
src-main.org.awakefw.file.http.BasicNameValuePairConvertor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file Show documentation
Show all versions of awake-file Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.file.http;
import java.io.IOException;
import java.util.List;
import java.util.Vector;
import org.apache.http.message.BasicNameValuePair;
import org.awakefw.commons.api.client.HttpProtocolParameters;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.api.util.HtmlConverter;
import org.awakefw.file.util.AwakeClientLogger;
import org.awakefw.file.util.convert.Pbe;
import org.awakefw.file.util.parms.Parameter;
import org.awakefw.file.version.AwakeFileVersionValues;
public class BasicNameValuePairConvertor {
/** Debug flag */
private static boolean DEBUG = AwakeDebug
.isSet(BasicNameValuePairConvertor.class);
/** The request params */
private List requestParams = null;
/** The http protocol parameters */
private HttpProtocolParameters httpProtocolParameters = null;
/**
* Constructor
*
* @param requestParams
* the request parameters list of BasicNameValuePair
*/
public BasicNameValuePairConvertor(List requestParams,
HttpProtocolParameters httpProtocolParameters) {
this.requestParams = requestParams;
this.httpProtocolParameters = httpProtocolParameters;
}
/**
* @return the convert & may be encrypted parameters
*/
public List convert() throws IOException {
List requestParamsConverted = new Vector();
for (BasicNameValuePair basicNameValuePair : requestParams) {
String paramName = basicNameValuePair.getName();
String paramValue = basicNameValuePair.getValue();
// Don't do it for STATEMENT_HOLDER ==> already has it's own Html
// conversion and encryption
if (! paramName.equals(Parameter.STATEMENT_HOLDER))
{
paramValue = HtmlConverter.toHtml(paramValue);
paramValue = encryptValue(paramName, paramValue);
}
debug("converted param name : " + paramName);
debug("converted param value: " + paramValue);
BasicNameValuePair basicNameValuePairEnc = new BasicNameValuePair(
paramName, paramValue);
requestParamsConverted.add(basicNameValuePairEnc);
}
// Add the Version
BasicNameValuePair basicNameValuePairEnc = new BasicNameValuePair(
Parameter.VERSION, AwakeFileVersionValues.VERSION);
requestParamsConverted.add(basicNameValuePairEnc);
return requestParamsConverted;
}
/**
* Encrypt the parameter value
*
* @param paramName
* the parameter name
* @param paramValue
* the parameter value
*
* @return the encrypted parameter value
*
* @throws IOException
* if any exception occurs
*/
private String encryptValue(String paramName, String paramValue)
throws IOException {
if (httpProtocolParameters == null) {
return paramValue;
}
char [] password = httpProtocolParameters.getEncryptionPassword();
if (password == null || password.length <= 1) {
return paramValue;
}
try {
paramValue = Pbe.AWAKE_ENCRYPTED
+ new Pbe().encryptToHexa(paramValue, password);
} catch (Exception e) {
String message = "Impossible to encrypt the value of the parameter "
+ paramName;
throw new IOException(message, e);
}
return paramValue;
}
/**
* Displays the given message if DEBUG is set.
*
* @param s
* the debug message
*/
private static void debug(String s) {
if (DEBUG) {
AwakeClientLogger.log(s);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy