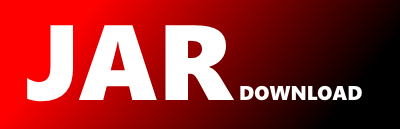
src-main.org.awakefw.file.http.HttpHostPartsExtractor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file Show documentation
Show all versions of awake-file Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
/**
*
*/
package org.awakefw.file.http;
import org.apache.commons.lang3.StringUtils;
/**
*
* Classes that allow to extract parts from a HTTP Host in the format
* "http://www.site.com:8080"
*
*
* @author Nicolas de Pomereu
*
*/
public class HttpHostPartsExtractor {
public static final int DEFAULT_PORT_HTTP = 80;
public static final int DEFAULT_PORT_HTTPS = 443;
/** A HTTP Host in the format "http://www.site.com:8080" */
private String httpHost = null;
/**
* Constructor
* @param httpHost A HTTP Host in the format "http://www.site.com:8080"
*/
public HttpHostPartsExtractor(String httpHost) {
if (httpHost == null) {
throw new IllegalArgumentException("httpHost can not be null!");
}
if (! httpHost.startsWith("http://") && httpHost.startsWith("https://")) {
throw new IllegalArgumentException("httpHost must start with \"http://\" or \"https://\". passed httpUrl is invalid: " + this.httpHost);
}
String hostname = StringUtils.substringAfter(httpHost, "://");
if (hostname.contains("/")) {
throw new IllegalArgumentException("httpHost can not contain / separator");
}
if (hostname.contains(":")) {
String portStr= StringUtils.substringAfter(hostname, ":");
if (! StringUtils.isNumeric(portStr)) {
throw new IllegalArgumentException("port is not numeric: " + httpHost);
}
}
this.httpHost = httpHost;
}
/**
* Returns the host name.
*
* @return the host name (IP or DNS name)
*/
public String getHostName() {
String hostname = StringUtils.substringAfter(httpHost, "://");
if (hostname.contains(":")) {
hostname = StringUtils.substringBefore(hostname, ":");
}
return hostname;
}
/**
* Returns the port.
*
* @return the host port, or 80
if not set for http scheme or
* or 443
if not set for https scheme.
*/
public int getPort() {
String hostname = StringUtils.substringAfter(httpHost, "://");
if (hostname.contains(":")) {
String portStr= StringUtils.substringAfter(hostname, ":");
int port = Integer.parseInt(portStr);
return port;
}
if (getSchemeName().equals("http")) {
return DEFAULT_PORT_HTTP;
}
else if (getSchemeName().equals("https")) {
return DEFAULT_PORT_HTTPS;
}
else {
throw new IllegalArgumentException("invalid scheme. Must be http or https. value is: " + getSchemeName());
}
}
/**
* Returns the scheme name ("http" or "https")
*
* @return "http" or "https"
*/
public String getSchemeName() {
String shemeName = StringUtils.substringBefore(httpHost, "://");
return shemeName;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy