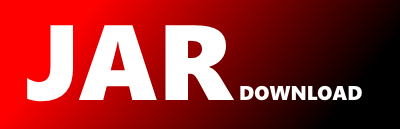
src-main.org.awakefw.file.http.InputStreamBodyForEngine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file Show documentation
Show all versions of awake-file Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.file.http;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import org.apache.http.entity.mime.content.InputStreamBody;
import org.awakefw.commons.api.client.AwakeProgressManager;
import org.awakefw.commons.api.client.HttpProtocolParameters;
import org.awakefw.file.api.util.DefaultParms;
import org.awakefw.file.util.AwakeClientLogger;
/**
* @author Nicolas de Pomereu
*
* this class externalize InputStreamBody only to provide a sendData()
* implementation. Each write on the output stream set the progress
* indicator for the file transfer engine.
*
* Note that the tempLen used for progress manager is httpTransferOne.tempLen
* because input stream may be reused dor subsquent calls.
*
* So, NEVER CLOSE THE inputStream.
*/
public class InputStreamBodyForEngine extends InputStreamBody {
/** The debug flag */
public boolean DEBUG = false;
/** The http protocol parameters */
private HttpProtocolParameters httpProtocolParameters;
/** The calling/owner thread */
private AwakeProgressManager awakeProgressManager = null;
/** The file we wan to upload */
private long filesLength = 0;
/**
* InputStreamBody Constructor.
* @param in
* the input stream to us post. May be reused in subsquents calls, do not close!
* @param mimeType
* the mime Type
* @param httpProtocolParameters
* the http protocol parameters
* @param awakeProgressManager
* the AwakeProgressManager instance
* @throws FileNotFoundException
* if the file is not a normal file or if it is not
* readable.
*/
public InputStreamBodyForEngine(InputStream in, String mimeType,
HttpProtocolParameters httpProtocolParameters,
AwakeProgressManager awakeProgressManager)
throws FileNotFoundException {
super(in, mimeType);
if (in == null) {
throw new IllegalArgumentException("in can not be null!");
}
this.httpProtocolParameters = httpProtocolParameters;
this.awakeProgressManager = awakeProgressManager;
}
/**
* @return true is there is an owner AND it's interrupted
*/
private boolean isAwakeProgressManagerInterrupted() {
if (awakeProgressManager == null) {
return false;
}
return awakeProgressManager.isCancelled();
}
/**
* Set the owner current value for progression bar
*
* @param current
*/
private void addOneToAwakeProgressManager() {
if (awakeProgressManager != null) {
int current = awakeProgressManager.getProgress();
current++;
if (current < HttpTransfer.MAXIMUM_PROGRESS_100) {
awakeProgressManager.setProgress(current);
}
}
}
/**
* Write the data in "source" to the specified stream.
*
* @param out
* The output stream.
* @throws IOException
* if an IO problem occurs.
* @see org.apache.commons.httpclient.methods.multipart.Part#sendData(OutputStream)
*/
@Override
public void writeTo(final OutputStream out) throws IOException {
debug("send data!");
debug("filesLength: " + filesLength);
// For Chunk Length comparison. If buffer read > chunk length ==> exit
long total = 0;
// For ProgressMonitor
int tempLen = 0;
long chunkLength = DefaultParms.DEFAULT_UPLOAD_CHUNK_LENGTH;
int uploadBufferSize = DefaultParms.DEFAULT_UPLOAD_BUFFER_SIZE;
if (httpProtocolParameters != null) {
chunkLength = httpProtocolParameters.getUploadChunkLength();
uploadBufferSize = httpProtocolParameters.getUploadBufferSize();
}
byte[] tmp = new byte[uploadBufferSize]; // Keep a huge
// size,
// otherwise
// it's very
// slow
// May be reused in subsquents calls, do not close!
InputStream instream = super.getInputStream();
int len;
if (awakeProgressManager != null) {
this.filesLength = awakeProgressManager.getLengthToTransfer();
}
while ((len = instream.read(tmp)) >= 0) {
tempLen += len;
total += len;
if (filesLength > 0
&& tempLen > filesLength
/ HttpTransfer.MAXIMUM_PROGRESS_100
) {
tempLen = 0;
try {
Thread.sleep(10); // TODO: adjust sleep time
// depending on fileIn length
} catch (InterruptedException e) {
e.printStackTrace();
}
addOneToAwakeProgressManager(); // For ProgressMonitor
// progress bar
// if (isAwakeProgressManagerInterrupted()) {
// throw new HttpTransferInterruptedException();
// }
if (isAwakeProgressManagerInterrupted()) {
debug("writeTo() INTERRUPTED!");
// throw new HttpTransferInterruptedException(Tag.AWAKE
// + "File upload interrupted by user.");
return;
}
}
// debug("len: " + len);
out.write(tmp, 0, len);
// Exit if chunk length is reached. We just send the chunck,
// InputStream
// will be reused
if (chunkLength > 0 && total > chunkLength) {
return;
}
}
}
/**
* debug tool
*/
private void debug(String s) {
if (DEBUG) {
AwakeClientLogger.log(s);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy