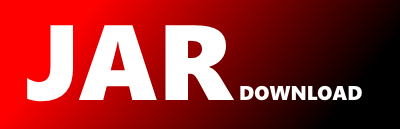
src-main.org.awakefw.file.http.engine.UrlDownloaderEngine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file Show documentation
Show all versions of awake-file Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.file.http.engine;
import java.io.File;
import java.net.MalformedURLException;
import java.net.URL;
import org.awakefw.commons.api.client.AwakeProgressManager;
import org.awakefw.file.api.client.AwakeFileSession;
import org.awakefw.file.api.client.AwakeUrl;
import org.awakefw.file.http.HttpTransfer;
import org.awakefw.file.util.AwakeClientLogger;
/**
* A Downloader Engine allows to download a file from the server.
*/
public class UrlDownloaderEngine extends Thread {
public static final String ERR_HTTP_SOCKET_EXCEPTION = "err_http_socket_exception";
public static final String ERR_HTTP_CONNECT_EXCEPTION = "err_http_connect_exception";
public static final String ERR_HTTP_UNKNOWN_SERVICE_EXCEPTION = "err_http_unknown_service_exception";
public static final String ERR_HTTP_PROTOCOL_EXCEPTION = "err_http_protocol_exception";
public static final String ERR_HTTP_IO_EXCEPTION = "err_http_io_exception";
public static final int RC_ERROR = -1;
public static final int RC_OK = 1;
/** The debug flag */
public boolean DEBUG = false;
/** The return code */
private int returnCode = RC_ERROR;
/** The Exception thrown if something *realy* bad happened */
private Exception exception = null;
/** The file to install */
private File file = null;
/** The URL of the file to download */
private URL url = null;
/** The ServerCallerNew instance (already logged user) */
private AwakeFileSession awakeFileSession;
/** The progress manager instance, to follow the transfer */
private AwakeProgressManager awakeProgressManager;
private int totalfileSize;
/**
* Constructor
*
* @param url
* the url of the file on the host
* @param file
* the file to create from a download
* @param filesLength
* The size of the file in bytes
*/
public UrlDownloaderEngine(AwakeFileSession awakeFileSession,
AwakeProgressManager awakeProgressManager, URL url, File file,
int filesLength) throws MalformedURLException {
if (awakeFileSession == null) {
throw new IllegalArgumentException(
"awakeFileSession can not be null!");
}
if (awakeProgressManager == null) {
throw new IllegalArgumentException(
"awakeProgressManager can not be null!");
}
if (file == null) {
throw new IllegalArgumentException("files can not be null!");
}
this.awakeFileSession = awakeFileSession;
this.awakeProgressManager = awakeProgressManager;
this.url = url;
this.file = file;
this.totalfileSize = filesLength;
}
public void run() {
try {
debug("InstallerEngine Begin");
awakeProgressManager.setLengthToTransfer(totalfileSize);
// Do the download
// awakeFileSession.downloadUrl(url, file);
AwakeUrl awakeUrl = new AwakeUrl(awakeFileSession.getHttpProxy(),
awakeFileSession.getHttpProtocolParameters());
awakeUrl.setAwakeProgressManager(awakeProgressManager);
awakeUrl.download(url, file);
returnCode = RC_OK;
awakeProgressManager.setProgress(HttpTransfer.MAXIMUM_PROGRESS_100);
debug("InstallerEngine End");
} catch (InterruptedException e) {
e.printStackTrace();
// This is a normal/regular interruption asked by user.
debug("FileDownloaderEngine Normal InterruptedException thrown by user Cancel!");
awakeProgressManager.setProgress(HttpTransfer.MAXIMUM_PROGRESS_100);
} catch (Exception e) {
e.printStackTrace();
debug("FileDownloaderEngine Exception thrown: " + e);
exception = e;
awakeProgressManager.setProgress(HttpTransfer.MAXIMUM_PROGRESS_100);
}
}
public File getFile() {
return file;
}
public int getReturnCode() {
return returnCode;
}
public Exception getException() {
return exception;
}
/**
* debug tool
*/
private void debug(String s) {
if (DEBUG) {
AwakeClientLogger.log(s);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy