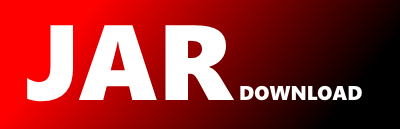
src-main.org.awakefw.file.servlet.AwakeFileManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file Show documentation
Show all versions of awake-file Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.file.servlet;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.io.StringReader;
import java.util.List;
import java.util.Set;
import java.util.logging.Logger;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.exception.ExceptionUtils;
import org.awakefw.commons.api.server.AwakeCommonsConfigurator;
import org.awakefw.commons.api.server.DefaultAwakeCommonsConfigurator;
import org.awakefw.commons.server.util.AwakeServerLogger;
import org.awakefw.file.api.server.AwakeFileConfigurator;
import org.awakefw.file.api.server.DefaultAwakeFileConfigurator;
import org.awakefw.file.api.server.fileaction.AwakeFileActionManager;
import org.awakefw.file.api.server.fileaction.DefaultAwakeFileActionManager;
import org.awakefw.file.servlet.convert.HttpServletRequestConvertor;
import org.awakefw.file.util.Tag;
import org.awakefw.file.util.TransferStatus;
import org.awakefw.file.version.AwakeFileVersion;
/**
* The main Awake FILE Manager Servlet
*
* @author Nicolas de Pomereu
*/
@SuppressWarnings("serial")
public class AwakeFileManager extends HttpServlet {
public static String CR_LF = System.getProperty("line.separator");
public static final String AWAKE_FILE_ACTION_MANAGER_CLASS_NAME = "awakeFileActionManagerClassName";
public static final String AWAKE_FILE_CONFIGURATOR_CLASS_NAME = "awakeFileConfiguratorClassName";
public static final String AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME = "awakeCommonsConfiguratorClassName";
private AwakeCommonsConfigurator awakeCommonsConfigurator = null;
private AwakeFileConfigurator awakeFileConfigurator = null;
private AwakeFileActionManager awakeFileActionManager = null;
/** The init error message trapped */
private String initErrrorMesage = null;
/** The Exception thrown at init */
private Exception exception = null;
/** Says that the AwakeServerLogger is ok and we can log the exceptions */
private boolean awakeServerLoggerOk = false;
/**
* Init.
*/
public void init(ServletConfig config) throws ServletException {
super.init(config);
// Variable use to store the current name when loading, used to
// detail
// the exception in the catch clauses
String classNameToLoad = null;
String awakeCommonsConfiguratorClassName = config
.getInitParameter(AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME);
String awakeFileConfiguratorClassName = config
.getInitParameter(AWAKE_FILE_CONFIGURATOR_CLASS_NAME);
String awakeFileActionManagerClassName = config
.getInitParameter(AWAKE_FILE_ACTION_MANAGER_CLASS_NAME);
try {
// Check spelling with first letter capitalized
if (awakeCommonsConfiguratorClassName == null
|| awakeCommonsConfiguratorClassName.isEmpty()) {
String capitalized = StringUtils
.capitalize(AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME);
awakeCommonsConfiguratorClassName = config
.getInitParameter(capitalized);
}
if (awakeFileConfiguratorClassName == null
|| awakeFileConfiguratorClassName.isEmpty()) {
String capitalized = StringUtils
.capitalize(AWAKE_FILE_CONFIGURATOR_CLASS_NAME);
awakeFileConfiguratorClassName = config
.getInitParameter(capitalized);
}
if (awakeFileActionManagerClassName == null
|| awakeFileActionManagerClassName.isEmpty()) {
String capitalized = StringUtils
.capitalize(AWAKE_FILE_ACTION_MANAGER_CLASS_NAME);
awakeFileActionManagerClassName = config
.getInitParameter(capitalized);
}
// System.out.println(Tag.AWAKE_START +
// "AwakeFileManager start...");
// Call the specific Awake FILE Configurator class to use
classNameToLoad = awakeCommonsConfiguratorClassName;
if (awakeCommonsConfiguratorClassName != null
&& !awakeCommonsConfiguratorClassName.isEmpty()) {
Class> c = Class.forName(awakeCommonsConfiguratorClassName);
awakeCommonsConfigurator = (AwakeCommonsConfigurator) c
.newInstance();
} else {
awakeCommonsConfigurator = new DefaultAwakeCommonsConfigurator();
}
// Immediately create the AwakeLogger
Logger logger = null;
try {
logger = awakeCommonsConfigurator.getLogger();
AwakeServerLogger.createLogger(logger);
AwakeServerLogger.log("Starting Awake FILE...");
awakeServerLoggerOk = true;
} catch (Exception e) {
initErrrorMesage = Tag.AWAKE_USER_CONFIG_FAIL
+ "Impossible to create the Logger: " + logger;
exception = e;
}
classNameToLoad = awakeFileConfiguratorClassName;
if (awakeFileConfiguratorClassName != null
&& !awakeFileConfiguratorClassName.isEmpty()) {
Class> c = Class.forName(awakeFileConfiguratorClassName);
awakeFileConfigurator = (AwakeFileConfigurator) c.newInstance();
} else {
awakeFileConfigurator = new DefaultAwakeFileConfigurator();
}
classNameToLoad = awakeFileActionManagerClassName;
if (awakeFileActionManagerClassName != null
&& !awakeFileActionManagerClassName.isEmpty()) {
Class> c = Class.forName(awakeFileActionManagerClassName);
awakeFileActionManager = (AwakeFileActionManager) c
.newInstance();
} else {
awakeFileActionManager = new DefaultAwakeFileActionManager();
}
} catch (ClassNotFoundException e) {
initErrrorMesage = Tag.AWAKE_USER_CONFIG_FAIL
+ "Impossible to load (ClassNotFoundException) Awake Configurator class: "
+ classNameToLoad;
exception = e;
} catch (InstantiationException e) {
initErrrorMesage = Tag.AWAKE_USER_CONFIG_FAIL
+ "Impossible to load (InstantiationException) Awake Configurator class: "
+ classNameToLoad;
exception = e;
} catch (IllegalAccessException e) {
initErrrorMesage = Tag.AWAKE_USER_CONFIG_FAIL
+ "Impossible to load (IllegalAccessException) Awake FILE Configurator class: "
+ classNameToLoad;
exception = e;
} catch (Exception e) {
initErrrorMesage = Tag.AWAKE_PRODUCT_FAIL + "Please contact support [email protected]";
exception = e;
}
if (awakeCommonsConfigurator == null) {
awakeCommonsConfiguratorClassName = AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME;
} else {
awakeCommonsConfiguratorClassName = awakeCommonsConfigurator
.getClass().getName();
}
if (awakeFileConfigurator == null) {
awakeFileConfiguratorClassName = AWAKE_FILE_CONFIGURATOR_CLASS_NAME;
} else {
awakeFileConfiguratorClassName = awakeFileConfigurator.getClass()
.getName();
}
if (awakeFileActionManager == null) {
awakeFileActionManagerClassName = AWAKE_FILE_ACTION_MANAGER_CLASS_NAME;
} else {
awakeFileActionManagerClassName = awakeFileActionManager.getClass()
.getName();
}
System.out.println();
System.out.println(Tag.AWAKE_START
+ org.awakefw.file.version.AwakeFileVersion.getVersion());
System.out.println(Tag.AWAKE_START + this.getServletName()
+ " Servlet:");
System.out.println(Tag.AWAKE_START
+ "- Init Parameter awakeCommonsConfiguratorClassName: " + CR_LF + Tag.AWAKE_START + " "
+ awakeCommonsConfiguratorClassName);
System.out.println(Tag.AWAKE_START
+ "- Init Parameter awakeFileConfiguratorClassName: " + CR_LF + Tag.AWAKE_START + " "
+ awakeFileConfiguratorClassName);
System.out.println(Tag.AWAKE_START
+ "- Init Parameter awakeFileActionManagerClassName: " + CR_LF + Tag.AWAKE_START + " "
+ awakeFileActionManagerClassName);
if (exception == null) {
System.out.println(Tag.AWAKE_START + "Awake FILE Configurator Status: OK.");
}
else {
System.out.println(Tag.AWAKE_START + "Awake FILE Configurator Status: KO.");
System.out.println(initErrrorMesage);
System.out.println( ExceptionUtils.getStackTrace(exception));
}
if (awakeServerLoggerOk) {
if (KawanNotifier.existsNoNotifyTxt()) {
AwakeServerLogger.log(Tag.AWAKE_START
+ "Notification to Kawan Servers: OFF");
}
} else {
if (KawanNotifier.existsNoNotifyTxt()) {
System.out.println(Tag.AWAKE_START
+ "Notification to Kawan Servers: OFF");
}
}
}
/**
* Test the configurators main methods to see if they throw Exceptions
*/
private void testConfiguratorMethods() {
if (exception == null) {
// Test that the login method does not throw an Exception
@SuppressWarnings("unused")
boolean isOk = false;
try {
isOk = awakeCommonsConfigurator.login("dummy",
"dummy".toCharArray());
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(awakeCommonsConfigurator, "login");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
File serverRootFile = awakeFileConfigurator.getServerRoot();
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "getServerRoot");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
// String tokenRecomputed = awakeCommonsConfigurator
// .computeAuthToken("dummy");
String tokenRecomputed = AwakeCommonsConfiguratorCall.computeAuthToken(awakeCommonsConfigurator, "dummy");
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "getServerRoot");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
//boolean forceHttps = awakeCommonsConfigurator.forceSecureHttp();
boolean forceHttps = AwakeCommonsConfiguratorCall.forceSecureHttp(awakeCommonsConfigurator);
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "forceSecureHttp");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
//Set usernameSet = awakeCommonsConfigurator.getBannedUsernames();
Set usernameSet = AwakeCommonsConfiguratorCall.getBannedUsernames(awakeCommonsConfigurator);
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "getBannedUsernames");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
List ipsBlacklist = AwakeCommonsConfiguratorCall.getIPsBlacklist(awakeCommonsConfigurator);
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "getIPsBlacklist");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
List ipsWhitelist = AwakeCommonsConfiguratorCall.getIPsWhitelist(awakeCommonsConfigurator);
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "getIPsWhitelist");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
boolean doUseOneRootPerUsername = awakeFileConfigurator
.useOneRootPerUsername();
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "useOneRootPerUsername");
exception = e;
}
}
}
/**
* Post request.
*/
@Override
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws IOException {
// If init fail, say it cleanly client, instead of bad 500 Servlet Error
if (exception != null) {
OutputStream out = response.getOutputStream();
writeLine(out, TransferStatus.SEND_FAILED);
writeLine(out, exception.getClass().getName()); // Exception class name
writeLine(out, initErrrorMesage + " Reason: " + exception.getMessage()); // Exception message
writeLine(out, ExceptionUtils.getStackTrace(exception)); // stack trace
return;
}
// Store the host info in RequestInfoStore
RequestInfoStore.init(request);
// Configure a repository (to ensure a secure temp location is used)
ServletContext servletContext = this.getServletConfig()
.getServletContext();
File servletContextTempDir = (File) servletContext
.getAttribute("javax.servlet.context.tempdir");
// Wrap the HttpServletRequest with HttpServletRequestEncrypted for
// parameters decryption
HttpServletRequestConvertor requestEncrypted = new HttpServletRequestConvertor(
request, awakeCommonsConfigurator);
// System.out.println(Tag.AWAKE_START + "AwakeFileManager started!");
ServerAwakeFileDispatch dispatch = new ServerAwakeFileDispatch();
dispatch.executeRequest(requestEncrypted, response,
servletContextTempDir, awakeCommonsConfigurator,
awakeFileConfigurator, awakeFileActionManager);
}
/**
* Write a line of string on the servlet output stream. Will add the
* necessary CR_LF
*
* @param out
* the servlet output stream
* @param s
* the string to write
* @throws IOException
*/
private void writeLine(OutputStream out, String s) throws IOException {
out.write((s + CR_LF).getBytes());
}
/**
* Get request.
*/
@Override
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
String status = ""
+ "OK & Running.";
// Test all methods
testConfiguratorMethods();
if (exception != null) {
String stackTrace = ExceptionUtils.getStackTrace(exception);
BufferedReader bufferedReader = new BufferedReader(
new StringReader(stackTrace));
StringBuffer sb = new StringBuffer();
String line = null;
while ((line = bufferedReader.readLine()) != null) {
// All subsequent lines contain the result
sb.append(line);
sb.append("
");
}
status = ""
+ initErrrorMesage + "
"
+ sb.toString();
}
String awakeCommonsConfiguratorClassName = null;
if (awakeCommonsConfigurator == null) {
awakeCommonsConfiguratorClassName = AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME;
} else {
awakeCommonsConfiguratorClassName = awakeCommonsConfigurator
.getClass().getName();
}
String awakeFileConfiguratorClassName = null;
if (awakeFileConfigurator == null) {
awakeFileConfiguratorClassName = AWAKE_FILE_CONFIGURATOR_CLASS_NAME;
} else {
awakeFileConfiguratorClassName = awakeFileConfigurator.getClass()
.getName();
}
String awakeFileActionManagerClassName = null;
if (awakeFileActionManager == null) {
awakeFileActionManagerClassName = AWAKE_FILE_ACTION_MANAGER_CLASS_NAME;
} else {
awakeFileActionManagerClassName = awakeFileActionManager.getClass()
.getName();
}
out.println("");
out.println("");
out.println("" + AwakeFileVersion.getVersion() + "");
out.println("
");
out.println("
");
out.println(this.getServletName() + " Servlet Configuration");
out.println("");
out.println("
");
out.println("");
out.println("");
out.println(" Configurator Parameter ");
out.println(" Configurator Value ");
out.println(" ");
out.println("");
out.println(" " + AWAKE_COMMONS_CONFIGURATOR_CLASS_NAME + " ");
out.println(" " + awakeCommonsConfiguratorClassName + " ");
out.println(" ");
out.println("");
out.println(" " + AWAKE_FILE_CONFIGURATOR_CLASS_NAME + " ");
out.println(" " + awakeFileConfiguratorClassName + " ");
out.println(" ");
out.println("");
out.println(" " + AWAKE_FILE_ACTION_MANAGER_CLASS_NAME + " ");
out.println(" " + awakeFileActionManagerClassName + " ");
out.println(" ");
out.println("
");
out.println("
");
out.println("");
out.println("");
out.println(" Awake FILE Configuration Status ");
out.println(" ");
out.println("");
out.println(" " + status + " ");
out.println(" ");
out.println("
");
out.println("");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy