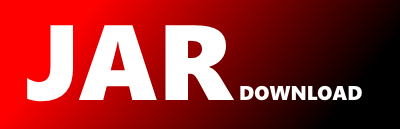
src-main.org.awakefw.file.servlet.KawanNotifier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file Show documentation
Show all versions of awake-file Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.file.servlet;
import java.io.BufferedReader;
import java.io.File;
import java.io.InputStreamReader;
import java.net.InetAddress;
import java.net.URL;
import java.net.URLConnection;
import java.util.HashSet;
import java.util.Set;
import javax.servlet.http.HttpServletRequest;
import org.apache.commons.io.IOUtils;
import org.awakefw.commons.api.server.AwakeCommonsConfigurator;
import org.awakefw.commons.api.server.util.Sha1;
import org.awakefw.commons.server.util.AwakeServerLogger;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.util.Tag;
/**
*
* Thread will notify our remote Kawan servers that a user has succeeded to
* login.
*
* This is done once during the JVM session per client user login, at first
* login. It is also done in a separated and secured thread: your Awake FILE
* Manager Servlet will not be slowed down by the notification and no Exceptions
* will be thrown. Notification contains only anonymous data that are not
* reversible and thus can not identify your server: hash value of your server ip
* address and login count. There are no notifications for localhost or
* 127.0.0.1 server name.
*
* Please note that the notification mechanism is important for us as software
* editor: it says if our software is used, and the average client users per
* installation. However, if you *really* don't want our remote Kawan servers to
* be notified by your server, just create the following file with any content:
* user.home/.awake/no_notify.txt
, where user.home
is
* the one of your Java EE Web server. Notification will be deactivated and at
* server startup the message
* "[AWAKE START] Notification to Kawan Servers: OFF"
* will be inserted in the log defined by
* {@link AwakeCommonsConfigurator#getLogger()}
* You can check the notification mechanism following source code in class
* org.awakefw.sql.servlet.KawanNotifier.java
.
*
* @author Nicolas de Pomereu
*/
public class KawanNotifier extends Thread {
private static boolean DEBUG = AwakeDebug.isSet(KawanNotifier.class);
/** All the usernames that have logged */
private static Set usernames = new HashSet();
/** the username that has logged */
private String username = null;
/** The product in use Awake SQL or Awake FILE + Version */
private String product = null;
/** The counter of users that successfully logged once */
private static int usernameCpt = 0;
/**
*
* @param username
* the username that has logged
* @param product
* the product in use
*/
public KawanNotifier(String username, String product) {
this.username = username;
this.product = product;
}
/**
* Notify the Host that a user has done a login - Done once in a server
* session.
* This is done in this secured thread: the Awake SQL Manager Servlet will
* not wait and all thrown Exceptions
are trapped
*/
public void run() {
BufferedReader bufferedReader = null;
try {
if (!usernames.contains(username)) {
usernames.add(username);
// Increment the number of users
usernameCpt++;
InetAddress inetAddress = InetAddress.getLocalHost();
String ip = inetAddress.toString();
// Make IP address completely anonymous
Sha1 sha1 = new Sha1();
ip = sha1.getHexHash(ip.getBytes());
String urlStr = "http://www.awake-sql.org/Notify?ip=" + ip
+ "&user=" + usernameCpt + "&product=" + product;
debug("urlStr: " + urlStr);
URL url = new URL(urlStr);
URLConnection urlConnection = url.openConnection();
bufferedReader = new BufferedReader(new InputStreamReader(
urlConnection.getInputStream()));
String inputLine;
while ((inputLine = bufferedReader.readLine()) != null) {
debug(inputLine);
}
}
} catch (Exception e) {
if (DEBUG) {
try {
AwakeServerLogger.log(Tag.AWAKE_EXCEPTION_RAISED
+ "Notify Exception: " + e.toString());
} catch (Exception e1) {
e1.printStackTrace(System.out);
}
}
} finally {
IOUtils.closeQuietly(bufferedReader);
}
}
/**
* Says it the username has already logged once in JVM session
* @param username
* the username that has logged
* @return thrue if the username has already logged once in JVM session
*/
public static boolean usernameAlreadyLogged(String username) {
return usernames.contains(username) ? true : false;
}
/**
* Says if the file user.home/.awake/no_notify.txt exists.
*
* @return true if user.home/.awake/no_notify.txt exists.
*/
public static boolean existsNoNotifyTxt() {
String userHome = System.getProperty("user.home");
if (!userHome.endsWith(File.separator)) {
userHome += File.separator;
}
File awakeDir = new File(userHome + ".awake");
awakeDir.mkdirs();
String NoNotifyTxt = awakeDir.toString() + File.separator
+ "no_notify.txt";
boolean noNotifyFileExists = new File(NoNotifyTxt).exists();
return noNotifyFileExists;
}
/**
* Says if web server is localhost (or 127.0.0.1)
*
* @return true if web server is localhost (or 127.0.0.1)
*/
public static boolean serverNameIsLocalhost() {
//RequestInfoStore requestInfoStore = new RequestInfoStore();
//String serverName = requestInfoStore.getServerName();
HttpServletRequest httpServletRequest = RequestInfoStore.getHttpServletRequest();
String serverName = httpServletRequest.getServerName();
if (serverName.toLowerCase().contains("localhost")
|| serverName.toLowerCase().contains("127.0.0.1")) {
debug("localhost serverName: " + serverName);
return true;
} else {
return false;
}
}
/**
* Method called by children Servlet for debug purpose Println is done only
* if class name name is in debug_list.ini
*/
public static void debug(String s) {
if (DEBUG) {
AwakeServerLogger.log(s);
System.out.println(s);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy