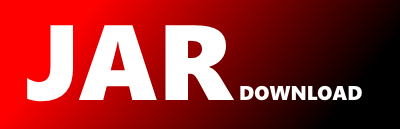
src-main.org.awakefw.file.servlet.ServerUserException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file Show documentation
Show all versions of awake-file Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.file.servlet;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.exception.ExceptionUtils;
import org.awakefw.commons.api.server.AwakeCommonsConfigurator;
import org.awakefw.commons.server.util.AwakeServerLogger;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.util.Tag;
/**
* @author Nicolas de Pomereu
*
* Universal Exception message when a use class (configurator) throws an
* Exception
*/
public class ServerUserException {
private static boolean DEBUG = AwakeDebug.isSet(ServerUserException.class);
/**
* Protected constructor
*/
protected ServerUserException() {
}
/**
* Generate the error message.
*
* @param awakeCommonsConfigurator
* the user Awake Commons Configurator
* @param method
* the method concerned
* @return the error message
*/
public static String getErrorMessage(
AwakeCommonsConfigurator awakeCommonsConfigurator, String method) {
String key = awakeCommonsConfigurator.getClass().getName();
String errorMessage = Tag.AWAKE_USER_CONFIG_FAIL + "The " + key + "."
+ method + "() method threw an Exception.";
return errorMessage;
}
/**
* Return Exception.getMessage() if the Exception is not thrown by a
* Configurator, else return Tag.AWAKE_USER_CONFIG_FAIL +
* Exception.getMessage()
*
* @param e
* the Exception thrown
* @return the wrapped/modifier exception
*/
public static String getMessage(Exception e) {
String UserConfigFailureMessage = "";
if (ServerUserException.isThrowingClassAwakeConfigurator(e)) {
UserConfigFailureMessage = Tag.AWAKE_USER_CONFIG_FAIL;
Class> theClass = ServerUserException
.extractThrowingClassFromException(e);
if (theClass != null) {
UserConfigFailureMessage += "in " + theClass.getName();
String methodName = ServerUserException
.extractThrowingMethodNameFromException(e);
UserConfigFailureMessage += "." + methodName + ": ";
}
}
return UserConfigFailureMessage + e.getMessage();
}
/**
* Says, for an Exception thrown, if the class that throws the Exception is
* an Awake Configurator
*
* @param e
* the Exception thrown
* @return thrue if the class that throws the Exception is an Awake
* Configurator
*/
private static boolean isThrowingClassAwakeConfigurator(Exception e) {
debug("e.toString(): " + e.toString());
Class> theClass = ServerUserException
.extractThrowingClassFromException(e);
if (theClass != null) {
debug("theClass: " + theClass.getName());
}
if (theClass == null) {
return false;
}
boolean isAwakeCommonsConfigurator = DoesClassImplementsAConfigurator(theClass);
if (isAwakeCommonsConfigurator) {
return true;
}
// maybe there is Default parent Configurator. Test if it's implement a
// Configurator
Class> cSuper = theClass.getSuperclass();
isAwakeCommonsConfigurator = DoesClassImplementsAConfigurator(cSuper);
return isAwakeCommonsConfigurator;
}
/**
* Says if a class is a Commons Configurator
*
* @param theClass
* @return
*/
private static boolean DoesClassImplementsAConfigurator(Class> theClass) {
boolean isAwakeCommonsConfigurator = false;
if (theClass != null) {
Class>[] interfaces = theClass.getInterfaces();
if (interfaces == null) {
return isAwakeCommonsConfigurator;
}
for (int i = 0; i < interfaces.length; i++) {
Class> theInterface = interfaces[i];
String interfaceName = theInterface.getName();
debug("interfaceName: " + interfaceName);
if (interfaceName
.equals("org.awakefw.commons.api.server.AwakeCommonsConfigurator")
|| interfaceName
.equals("org.awakefw.file.api.server.AwakeFileConfigurator")
|| interfaceName
.equals("org.awakefw.file.api.server.fileaction.AwakeFileActionManager")
|| interfaceName
.equals("org.awakefw.sql.api.server.AwakeSqlConfigurator")
) {
isAwakeCommonsConfigurator = true;
break;
}
}
}
return isAwakeCommonsConfigurator;
}
/**
* Return the class where the Exception has been thrown
*
* @param e
* the exception thrown
* @return the class that throws the Exception
*/
private static Class> extractThrowingClassFromException(Exception e) {
try {
String statckTrace = ExceptionUtils.getStackTrace(e);
String className = StringUtils.substringAfter(statckTrace, "at ");
className = StringUtils.substringBefore(className, "(");
className = StringUtils.substringBeforeLast(className, ".");
debug("className: " + ":" + className + ":");
Class> theClass = Class.forName(className);
return theClass;
} catch (ClassNotFoundException e1) {
e1.printStackTrace(System.out);
return null;
}
}
/**
* Return the class where the Exception has been thrown
*
* @param e
* the exception thrown
* @return the class that throws the Exception
*/
private static String extractThrowingMethodNameFromException(Exception e) {
String statckTrace = ExceptionUtils.getStackTrace(e);
String className = StringUtils.substringAfter(statckTrace, "at ");
className = StringUtils.substringBefore(className, "(");
String methodName = StringUtils.substringAfterLast(className, ".");
debug("className : " + ":" + className + ":");
debug("methodName: " + ":" + methodName + ":");
return methodName;
}
private static void debug(String s) {
if (DEBUG) {
AwakeServerLogger.log(s);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy