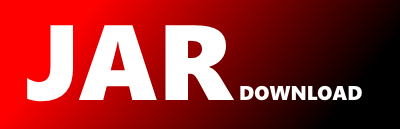
src-main.org.awakefw.file.util.AwakeFileUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-file Show documentation
Show all versions of awake-file Show documentation
Awake FILE is a secure Open Source framework that allows to program very easily file uploads/downloads and RPC through http. File transfers include
powerful features like file chunking and automatic recovery mechanism.
Security has been taken into account from the design: server side allows
to specify strong security rules in order to protect the files and to secure the RPC calls.
/*
* This file is part of Awake FILE.
* Awake FILE: Easy file upload & download over HTTP with Java.
* Copyright (C) 2014, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake FILE is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* Awake FILE is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.file.util;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.RandomAccessFile;
import java.util.Date;
import java.util.List;
import java.util.UUID;
import java.util.Vector;
import org.apache.commons.io.IOUtils;
/**
* Misc file utilities
*
* @author Nicolas de Pomereu
*
*/
public class AwakeFileUtil {
/** The DEBUG flag */
public static boolean DEBUG = false;
public static String CR_LF = System.getProperty("line.separator");
/** List of files */
//private List m_fileList = new Vector();
/**
* Constructor
*/
protected AwakeFileUtil() {
super();
}
/**
* Build a unique string
*
* @return a unique string
*/
public static synchronized String getUniqueId() {
// long uniqueLong = new Date().getTime();
//
// String unique = Long.toString(uniqueLong);
//
// double randomDouble = Math.random();
// String random = Double.toString(randomDouble);
// random = StringUtils.substringAfter(random, ".");
// random = StringUtils.substring(random, 0, 4);
//
// unique += "-" + random;
UUID idOne = UUID.randomUUID();
return idOne.toString();
}
/**
* Returns System.getProperty("user.home") for all devices except for Android which
* returns java.io.tmpdir/awake-user-home
*
* @return System.getProperty("user.home") for all devices except for Android which
* returns java.io.tmpdir/awake-user-home
*/
public static String getUserHome()
{
String userHome = System.getProperty("user.home");
if (! AwakeSystemUtil.isAndroid()) {
return userHome;
}
// We are on andro?d
userHome = System.getProperty("java.io.tmpdir");
if (!userHome.endsWith(File.separator)) {
userHome += File.separator;
}
userHome += "awake-user-home";
File userHomeFile = new File(userHome);
userHomeFile.mkdirs();
return userHome;
}
/**
* @return the Awake temp directory (create it if not exists)
*/
public static String getAwakeTempDir() {
// String dir = System.getProperty("java.io.tmpdir");
// if (!dir.endsWith(File.separator)) {
// dir += File.separator;
// }
// String tempDir = dir + ".awake" + File.separator;
String tempDir = getUserHome();
if (!tempDir.endsWith(File.separator)) {
tempDir += File.separator;
}
tempDir += ".awake" + File.separator + "tmp";
File tempDirFile = new File(tempDir);
tempDirFile.mkdirs();
return tempDir;
}
/**
* Extract the first line of a presumed text file
* @param file the presumed text file to extract the first line from
* @return the first line of the file
* @throws IOException
*/
public static String getFirstLineOfFile(File file) throws IOException {
if (file == null) {
throw new IllegalArgumentException(Tag.AWAKE_PRODUCT_FAIL + "receiveFile is null");
}
if (! file.exists()) {
throw new FileNotFoundException(Tag.AWAKE_PRODUCT_FAIL + "receiveFile does not exists: " + file);
}
BufferedReader bufferedReader = null;
try {
// Read content of first line.
bufferedReader = new BufferedReader(new InputStreamReader(
new FileInputStream(file)));
String firstLine = bufferedReader.readLine();
return firstLine;
} finally {
IOUtils.closeQuietly(bufferedReader);
}
}
// /**
// * Cleans the temp directory
// */
// public static void cleanTempDir()
// {
// try {
// String dir = getAwakeTempDir();
// File [] files = new File(dir).listFiles();
// if (files != null)
// {
// for (int i = 0; i < files.length; i++) {
// boolean deleted = files[i].delete();
// if (! deleted) {
// AwakeLogger.log("Could not delete in Awake Temp Dir file: " + files[i].getName());
// }
// }
// }
// } catch (Exception e) {
// AwakeLogger.log(e.toString());
// }
// }
/**
* Return true if the filename is a Linux possible Filename
*
* @param filename
* the filename to test
* @return true if the filename is a Linux Filename
*/
public static boolean isPossibleLinuxFilename(String filename) {
if (filename.indexOf("\\") != -1 || // Windows
filename.indexOf("/") != -1 || // Windows
filename.indexOf(":") != -1 || // Windows
filename.indexOf("*") != -1 || // Windows
filename.indexOf("?") != -1 || // Windows
filename.indexOf("\"") != -1 || // Windows
filename.indexOf("<") != -1 || // Windows
filename.indexOf(">") != -1 || // Windows
filename.indexOf("|") != -1 || // Windows
filename.indexOf("@") != -1 || // Linux
filename.indexOf(" ") != -1) // Linux
{
return false;
} else {
return true;
}
}
/**
* Return true if the fils exists, is readable and is not locked by another
* process
* New version that works because it uses RandomAccessFile and will throw an
* exception if another file has locked the file
*/
public static boolean isUnlockedForRead(File file) {
if (file.exists() && file.canRead()) {
if (file.isDirectory()) {
return true;
}
try {
RandomAccessFile raf = new RandomAccessFile(file, "r");
raf.close();
debug(new Date() + " " + file + " OK!");
return true;
} catch (Exception e) {
debug(new Date() + " " + file + " LOCKED! " + e.getMessage());
}
} else {
debug(new Date() + " " + file + " LOCKED! File exists(): "
+ file.exists() + " File canWrite: " + file.canWrite());
}
return false;
}
/**
* Return true if the fils exists and is readable and writable not locked by
* another process
* New version that works because it uses RandomAccessFile and will throw an
* exception if another file has locked the file
*/
public static boolean isUnlockedForWrite(File file) {
if (file.exists() && file.canWrite()) {
if (file.isDirectory()) {
return true;
}
try {
RandomAccessFile raf = new RandomAccessFile(file, "rw");
raf.close();
debug(new Date() + " " + file + " OK!");
return true;
} catch (Exception e) {
debug(new Date() + " " + file + " LOCKED! " + e.getMessage());
}
} else {
debug(new Date() + " " + file + " LOCKED! File exists(): "
+ file.exists() + " File canWrite: " + file.canWrite());
}
return false;
}
/**
* Recuse research of a files. Result is stored in m_fileList
*
* @param fileList
* the initial list
* @param recurse
* if true, search inside directories
*/
// private static void searchFiles(File[] fileList, boolean recurse) {
// if (fileList == null) {
// throw new IllegalArgumentException("File list can't be null!");
// }
//
// for (int i = 0; i < fileList.length; i++) {
// m_fileList.add(fileList[i]);
//
// if (fileList[i].isDirectory()) {
// if (recurse) {
// searchFiles(fileList[i].listFiles(), recurse);
// }
// }
// }
// }
/**
* Extract all files from a list of files, with recurse options
*
* @param fileList
* the initial list
* @param recurse
* if true, search inside directories
*
* @return the extracted all files
*/
// public static List listAllFiles(List fileList, boolean recurse) {
//
// if (fileList == null) {
// throw new IllegalArgumentException("File list can't be null!");
// }
//
// if (fileList.isEmpty()) {
// return new Vector();
// }
//
// File[] files = new File[fileList.size()];
//
// for (int i = 0; i < fileList.size(); i++) {
// files[i] = fileList.get(i);
// }
//
// searchFiles(files, recurse);
// return m_fileList;
// }
/**
*
* Extract the files that are not directories from a file list
*
* @param fileList
* the initial list (firt level only)
* @return the file list
*/
public static List extractFilesOnly(List fileList) {
if (fileList == null) {
throw new IllegalArgumentException("File list can't be null!");
}
if (fileList.isEmpty()) {
return new Vector();
}
List files = new Vector();
for (int i = 0; i < fileList.size(); i++) {
if (!fileList.get(i).isDirectory()) {
files.add(fileList.get(i));
}
}
return files;
}
/**
*
* Extract the directories only from a file list
*
* @param fileList
* the initial list (firt level only)
* @return the file list
*/
public static List extractDirectoriesOnly(List fileList) {
List directories = new Vector();
for (int i = 0; i < fileList.size(); i++) {
if (fileList.get(i).isDirectory()) {
directories.add(fileList.get(i));
}
}
return directories;
}
/**
* Put the content of a file as HTML into a String
* No carriage returns will be included in output String
*
* @param fileIn
* The HTML text file
* @return The content in text
* @throws IOException
*/
public static String getHtmlContent(File fileIn) throws IOException {
if (fileIn == null) {
throw new IllegalArgumentException("File name can't be null!");
}
BufferedReader br = null;
try {
String text = "";
br = new BufferedReader(new FileReader(fileIn));
String line = null;
while ((line = br.readLine()) != null) {
text += line;
}
return text;
} finally {
IOUtils.closeQuietly(br);
}
}
/**
* debug tool
*/
private static void debug(String s) {
if (DEBUG) {
AwakeClientLogger.log(s);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy