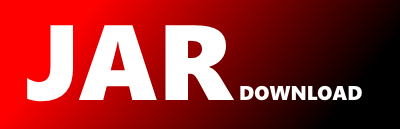
src-main.org.awakefw.sql.jdbc.CallableStatementHttp Maven / Gradle / Ivy
Show all versions of awake-sql Show documentation
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.jdbc;
/**
* Creates and handle a Callable Statement Http.
*
* @author Alexandre Becquereau
*/
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileOutputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.Reader;
import java.io.Writer;
import java.math.BigDecimal;
import java.net.URL;
import java.sql.Array;
import java.sql.Blob;
import java.sql.CallableStatement;
import java.sql.Clob;
import java.sql.Date;
import java.sql.NClob;
import java.sql.Ref;
import java.sql.ResultSet;
import java.sql.RowId;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
import java.sql.SQLXML;
import java.sql.Time;
import java.sql.Timestamp;
import java.util.Calendar;
import java.util.Map;
import org.apache.commons.io.IOUtils;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.api.util.HtmlConverter;
import org.awakefw.file.util.AwakeFileUtil;
import org.awakefw.file.util.KeepTempFilePolicyParms;
import org.awakefw.file.util.Tag;
import org.awakefw.sql.jdbc.http.JdbcHttpCallableStatementTransfer;
import org.awakefw.sql.jdbc.util.AwakeAsciiStream;
import org.awakefw.sql.jdbc.util.AwakeInputStream;
import org.awakefw.sql.jdbc.util.AwakeReader;
import org.awakefw.sql.jdbc.util.CallableResultFileSplitter;
import org.awakefw.sql.jdbc.util.CallableUtil;
import org.awakefw.sql.jdbc.util.ParametersUtilCallable;
import org.awakefw.sql.json.no_obfuscation.CallableStatementHolder;
import org.awakefw.sql.transport.TransportConverter;
import org.awakefw.sql.util.FileNameFromBlobBuilder;
import org.awakefw.sql.util.SqlActionCallable;
public class CallableStatementHttp extends PreparedStatementHttp implements
CallableStatement {
/** Debug flag */
private static boolean DEBUG = AwakeDebug
.isSet(CallableStatementHttp.class);
/** Streams & blobs/clobs are not supported in this version */
private static boolean STREAMS_SUPPORTED = false;
/**
* The holder that contains the sql order and the list if (parameter type,
* parameter value ) for prepared statements
**/
private CallableStatementHolder callableStatementHolder = null;
/**
* The update count returned by PreparedStatementHttp.getUpdateCount() after
* an execute()
*/
// private int updateCount = 0;
/** The statement to execute */
private String sql = null;
public CallableStatementHttp(ConnectionHttp connectionHttp, String sql,
int resultSetType, int resultSetConcurrency,
int resultSetHoldability) throws SQLException {
super(connectionHttp, sql, resultSetType, resultSetConcurrency,
resultSetHoldability);
this.sql = sql.trim();
callableStatementHolder = new CallableStatementHolder(sql,
resultSetType, resultSetConcurrency, resultSetHoldability);
callableStatementHolder.setHtmlEncodingOn(connectionHttp
.isHtmlEncodingOn());
}
@Override
public boolean execute() throws SQLException {
testIfClosed();
if (connectionHttp.isStatelessMode()) {
if (!connectionHttp.getAutoCommit()) {
throw new IllegalStateException(Tag.AWAKE
+ "execute can\'t be executed when auto commit is off.");
}
}
lastExecuteIsRaw = true;
// Check that all parameters values are set
ParametersUtilCallable.checkParameters(callableStatementHolder);
callableStatementHolder.setExecuteUpdate(false);
callableStatementHolder.setFetchSize(fetchSize);
callableStatementHolder.setMaxRows(maxRows);
callableStatementHolder.setQueryTimeout(queryTimeout);
callableStatementHolder.setEscapeProcessing(escapeProcessingInt);
// Reset the fields values
rsFromExecute = null;
// Send order to Server to SQL Executor
JdbcHttpCallableStatementTransfer jdbcHttpCallableStatementTransfer = new JdbcHttpCallableStatementTransfer(
connectionHttp, connectionHttp.getAuthenticationToken());
File receiveFile = jdbcHttpCallableStatementTransfer
.getFileFromExecuteOnServer(callableStatementHolder, SqlActionCallable.ACTION_SQL_CALLABLE_EXECUTE_RAW);
debug("getFileFromExecuteOnServer() : " + CR_LF + receiveFile);
CallableResultFileSplitter callableResultFileSplitter = new CallableResultFileSplitter(
receiveFile);
File callableStatementHolderFile = callableResultFileSplitter.getCallableStatementHolderFile();
this.callableStatementHolder = CallableUtil.fromRsFile(
callableStatementHolderFile,
connectionHttp);
if (!KeepTempFilePolicyParms.KEEP_TEMP_FILE && !DEBUG) {
callableStatementHolderFile.delete();
}
File rsFile = callableResultFileSplitter.getResultSetFile();
boolean fileResultSet = super.isFileResultSet(rsFile);
if (fileResultSet) {
rsFromExecute = new ResultSetHttp(connectionHttp, null, this,
rsFile);
return true;
}
else {
if (!KeepTempFilePolicyParms.KEEP_TEMP_FILE && !DEBUG) {
rsFile.delete();
}
}
return false;
}
/**
* Executes the SQL query in this CallableStatement
object and
* returns the ResultSet
object generated by the query.
*
* @return a ResultSet
object that contains the data produced
* by the query; never null
* @exception SQLException
* if a database access error occurs or the SQL statement
* does not return a ResultSet
object
*/
@Override
public ResultSet executeQuery() throws SQLException {
testIfClosed();
if (connectionHttp.isStatelessMode()) {
if (!connectionHttp.getAutoCommit()) {
throw new IllegalStateException(
Tag.AWAKE
+ "executeQuery() can\'t be executed when auto commit is off.");
}
}
// Check that all parameters values are set
ParametersUtilCallable.checkParameters(callableStatementHolder);
callableStatementHolder.setExecuteUpdate(false);
callableStatementHolder.setFetchSize(fetchSize);
callableStatementHolder.setMaxRows(maxRows);
callableStatementHolder.setQueryTimeout(queryTimeout);
callableStatementHolder.setEscapeProcessing(escapeProcessingInt);
// Send unique order to Server to SQL Executor
JdbcHttpCallableStatementTransfer jdbcHttpCallableStatementTransfer = new JdbcHttpCallableStatementTransfer(
connectionHttp, connectionHttp.getAuthenticationToken());
File receiveFile = jdbcHttpCallableStatementTransfer
.getFileFromExecuteOnServer(callableStatementHolder, SqlActionCallable.ACTION_SQL_CALLABLE_EXECUTE_QUERY);
debug("getFileFromExecuteOnServer() : " + CR_LF + receiveFile);
CallableResultFileSplitter callableResultFileSplitter = new CallableResultFileSplitter(
receiveFile);
File callableStatementHolderFile = callableResultFileSplitter.getCallableStatementHolderFile();
this.callableStatementHolder = CallableUtil.fromRsFile(
callableStatementHolderFile,
connectionHttp);
if (!KeepTempFilePolicyParms.KEEP_TEMP_FILE && !DEBUG) {
callableStatementHolderFile.delete();
}
File rsFile = callableResultFileSplitter.getResultSetFile();
ResultSet rs = new ResultSetHttp(connectionHttp, null, this, rsFile);
return rs;
}
/*
* (non-Javadoc)
*
* @see org.awakefw.sql.jdbc.PreparedStatementHttp#executeUpdate()
*/
@Override
public synchronized int executeUpdate() throws SQLException {
throw new SQLException(Tag.AWAKE + "executeUpdate is not supported for CallableStatement.");
}
@Override
public BigDecimal getBigDecimal(int parameterIndex) throws SQLException {
testIfClosed();
return (BigDecimal) callableStatementHolder
.getParameter(parameterIndex);
}
@Override
public BigDecimal getBigDecimal(String parameterName) throws SQLException {
testIfClosed();
return (BigDecimal) callableStatementHolder.getParameter(parameterName);
}
@Override
public BigDecimal getBigDecimal(int parameterIndex, int scale)
throws SQLException {
testIfClosed();
if (scale < 0) {
throw new SQLException("invalid scale: "+ scale + ". Scale must be >= 0" );
}
BigDecimal bd = (BigDecimal) callableStatementHolder
.getParameter(parameterIndex);
if (bd != null) {
bd = bd.setScale(scale, BigDecimal.ROUND_DOWN);
}
return bd;
}
@Override
public String getNString(int parameterIndex) throws SQLException {
testIfClosed();
return (String) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public Blob getBlob(int parameterIndex) throws SQLException {
testIfClosed();
return (Blob) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public Blob getBlob(String parameterName) throws SQLException {
testIfClosed();
return (Blob) callableStatementHolder.getParameter(parameterName);
}
@Override
public boolean getBoolean(int parameterIndex) throws SQLException {
testIfClosed();
return (Boolean) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public boolean getBoolean(String parameterName) throws SQLException {
testIfClosed();
return (Boolean) callableStatementHolder.getParameter(parameterName);
}
@Override
public byte getByte(int parameterIndex) throws SQLException {
testIfClosed();
return (Byte) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public byte getByte(String parameterName) throws SQLException {
testIfClosed();
return (Byte) callableStatementHolder.getParameter(parameterName);
}
@Override
public Clob getClob(int parameterIndex) throws SQLException {
testIfClosed();
return (Clob) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public Clob getClob(String parameterName) throws SQLException {
testIfClosed();
return (Clob) callableStatementHolder.getParameter(parameterName);
}
@Override
public Date getDate(int parameterIndex) throws SQLException {
testIfClosed();
return (Date) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public Date getDate(String parameterName) throws SQLException {
testIfClosed();
return (Date) callableStatementHolder.getParameter(parameterName);
}
@Override
public double getDouble(int parameterIndex) throws SQLException {
testIfClosed();
return (Double) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public double getDouble(String parameterName) throws SQLException {
testIfClosed();
return (Double) callableStatementHolder.getParameter(parameterName);
}
@Override
public float getFloat(int parameterIndex) throws SQLException {
testIfClosed();
return (Float) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public float getFloat(String parameterName) throws SQLException {
testIfClosed();
return (Float) callableStatementHolder.getParameter(parameterName);
}
@Override
public int getInt(int parameterIndex) throws SQLException {
testIfClosed();
return (Integer) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public int getInt(String parameterName) throws SQLException {
testIfClosed();
return (Integer) callableStatementHolder.getParameter(parameterName);
}
@Override
public long getLong(int parameterIndex) throws SQLException {
testIfClosed();
return (Long) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public long getLong(String parameterName) throws SQLException {
testIfClosed();
return (Long) callableStatementHolder.getParameter(parameterName);
}
@Override
public Object getObject(int parameterIndex) throws SQLException {
testIfClosed();
return callableStatementHolder.getParameter(parameterIndex);
}
@Override
public Object getObject(String parameterName) throws SQLException {
testIfClosed();
return callableStatementHolder.getParameter(parameterName);
}
@Override
public short getShort(int parameterIndex) throws SQLException {
testIfClosed();
return (Short) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public short getShort(String parameterName) throws SQLException {
testIfClosed();
return (Short) callableStatementHolder.getParameter(parameterName);
}
@Override
public String getString(int parameterIndex) throws SQLException {
testIfClosed();
return (String) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public String getString(String parameterName) throws SQLException {
testIfClosed();
return (String) callableStatementHolder.getParameter(parameterName);
}
@Override
public Timestamp getTimestamp(int parameterIndex) throws SQLException {
testIfClosed();
return (Timestamp) callableStatementHolder.getParameter(parameterIndex);
}
@Override
public Timestamp getTimestamp(String parameterName) throws SQLException {
testIfClosed();
return (Timestamp) callableStatementHolder.getParameter(parameterName);
}
@Override
public String getNString(String parameterName) throws SQLException {
testIfClosed();
return (String) callableStatementHolder.getParameter(parameterName);
}
@Override
public void registerOutParameter(int parameterIndex, int sqlType)
throws SQLException {
testIfClosed();
callableStatementHolder.setOutParameter(parameterIndex, sqlType);
}
/**
* Sets the designated parameter to SQL NULL
.
*
*
* Note: You must specify the parameter's SQL type.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param sqlType
* the SQL type code defined in java.sql.Types
* @exception SQLException
* if a database access error occurs
*/
public void setNull(int parameterIndex, int sqlType) throws SQLException {
testIfClosed();
callableStatementHolder.setNullParameter(parameterIndex, sqlType);
}
/**
* Sets the designated parameter to the given Java boolean
* value. The driver converts this to an SQL BIT
value when it
* sends it to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
@Override
public void setBoolean(int parameterIndex, boolean x) throws SQLException {
testIfClosed();
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
* Sets the designated parameter to the given Java short
value.
* The driver converts this to an SQL SMALLINT
value when it
* sends it to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
@Override
public void setShort(int parameterIndex, short x) throws SQLException {
testIfClosed();
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
* Sets the designated parameter to the given Java int
value.
* The driver converts this to an SQL INTEGER
value when it
* sends it to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
@Override
public void setInt(int parameterIndex, int x) throws SQLException {
testIfClosed();
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
* Sets the designated parameter to the given Java long
value.
* The driver converts this to an SQL BIGINT
value when it
* sends it to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
@Override
public void setLong(int parameterIndex, long x) throws SQLException {
testIfClosed();
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
* Sets the designated parameter to the given Java float
value.
* The driver converts this to an SQL FLOAT
value when it sends
* it to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
@Override
public void setFloat(int parameterIndex, float x) throws SQLException {
testIfClosed();
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
* Sets the designated parameter to the given Java double
* value. The driver converts this to an SQL DOUBLE
value when
* it sends it to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
@Override
public void setDouble(int parameterIndex, double x) throws SQLException {
testIfClosed();
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
* Sets the designated parameter to the given
* java.math.BigDecimal
value. The driver converts this to an
* SQL NUMERIC
value when it sends it to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if parameterIndex does not correspond to a parameter
* marker in the SQL statement; if a database access error
* occurs or this method is called on a closed
* PreparedStatement
*/
@Override
public void setBigDecimal(int parameterIndex, BigDecimal x)
throws SQLException {
testIfClosed();
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
* Sets the designated parameter to the given Java String
* value. The driver converts this to an SQL VARCHAR
or
* LONGVARCHAR
value (depending on the argument's size relative
* to the driver's limits on VARCHAR
values) when it sends it
* to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
@Override
public void setString(int parameterIndex, String x) throws SQLException {
testIfClosed();
if (x != null && x.length() > connectionHttp.getMaxLengthForString()) {
throw new SQLException(
"String is too big for upload with Awake SQL: "
+ x.length()
+ " bytes. Maximum length authorized is: "
+ connectionHttp.getMaxLengthForString());
}
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
* Sets the designated parameter to the given Java byte
value.
* The driver converts this to an SQL TINYINT
value when it
* sends it to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
@Override
public void setByte(int parameterIndex, byte x) throws SQLException {
testIfClosed();
byte[] theByte = new byte[1];
theByte[0] = x;
String encodedString = TransportConverter.toTransportFormat(theByte);
// parameterValues.put(parameterIndex, hexString);
callableStatementHolder.setParameter(parameterIndex, encodedString);
}
/**
* Sets the designated parameter to the given Java array of bytes. The
* driver converts this to an SQL VARBINARY
or
* LONGVARBINARY
(depending on the argument's size relative to
* the driver's limits on VARBINARY
values) when it sends it to
* the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
@Override
public void setBytes(int parameterIndex, byte[] x) throws SQLException {
testIfClosed();
String encodedString = TransportConverter.toTransportFormat(x);
// parameterValues.put(parameterIndex, hexString);
callableStatementHolder.setParameter(parameterIndex, encodedString);
}
/**
* Sets the designated parameter to the given java.sql.Date
* value. The driver converts this to an SQL DATE
value when it
* sends it to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
@Override
public void setDate(int parameterIndex, java.sql.Date x)
throws SQLException {
testIfClosed();
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
* Sets the designated parameter to the given java.sql.Time
* value. The driver converts this to an SQL TIME
value when it
* sends it to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
public void setTime(int parameterIndex, java.sql.Time x)
throws SQLException {
testIfClosed();
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
* Sets the designated parameter to the given
* java.sql.Timestamp
value. The driver converts this to an SQL
* TIMESTAMP
value when it sends it to the database.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the parameter value
* @exception SQLException
* if a database access error occurs
*/
@Override
public void setTimestamp(int parameterIndex, java.sql.Timestamp x)
throws SQLException {
testIfClosed();
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
*
* Sets the value of the designated parameter using the given object. The
* second parameter must be of type Object
; therefore, the
* java.lang
equivalent objects should be used for built-in
* types.
*
*
* The JDBC specification specifies a standard mapping from Java
* Object
types to SQL types. The given argument will be
* converted to the corresponding SQL type before being sent to the
* database.
*
*
* Note that this method may be used to pass datatabase- specific abstract
* data types, by using a driver-specific Java type.
*
* If the object is of a class implementing the interface
* SQLData
, the JDBC driver should call the method
* SQLData.writeSQL
to write it to the SQL data stream. If, on
* the other hand, the object is of a class implementing Ref
,
* Blob
, Clob
, Struct
, or
* Array
, the driver should pass it to the database as a value
* of the corresponding SQL type.
*
* This method throws an exception if there is an ambiguity, for example, if
* the object is of a class implementing more than one of the interfaces
* named above.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the object containing the input parameter value
* @exception SQLException
* if a database access error occurs or the type of the given
* object is ambiguous
*/
@Override
public void setObject(int parameterIndex, Object x) throws SQLException {
testIfClosed();
if (x != null
&& x.toString().length() > connectionHttp
.getMaxLengthForString()) {
throw new SQLException(
"Object is too big for upload with Awake SQL: "
+ x.toString().length()
+ " bytes. Maximum length authorized is: "
+ connectionHttp.getMaxLengthForString());
}
callableStatementHolder.setParameter(parameterIndex, x);
}
/**
* Sets the designated parameter to the given input stream. When a very
* large binary value is input to a LONGVARBINARY
parameter, it
* may be more practical to send it via a java.io.InputStream
* object. The data will be read from the stream as needed until end-of-file
* is reached.
*
*
* Note: This stream object can either be a standard Java stream
* object or your own subclass that implements the standard interface.
*
* Note: Consult your JDBC driver documentation to determine if it
* might be more efficient to use a version of setBinaryStream
* which takes a length parameter.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the java input stream which contains the binary parameter
* value
* @exception SQLException
* if parameterIndex does not correspond to a parameter
* marker in the SQL statement; if a database access error
* occurs or this method is called on a closed
* PreparedStatement
* @throws SQLFeatureNotSupportedException
* if the JDBC driver does not support this method
* @since 1.6
*/
@Override
public void setBinaryStream(int parameterIndex, java.io.InputStream x)
throws SQLException {
testIfClosed();
if (!STREAMS_SUPPORTED) {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
// Create the file from the input Stream, naming it on the table name
FileNameFromBlobBuilder fileNameFromBlobBuilder = new FileNameFromBlobBuilder(
sql, parameterIndex, false);
String rawRemoteFileName = fileNameFromBlobBuilder.getFileName();
String dir = AwakeFileUtil.getAwakeTempDir();
File blobFile = new File(dir + rawRemoteFileName);
debug("rawRemoteFileName: " + rawRemoteFileName);
debug("blobFile : " + blobFile);
OutputStream out = null;
try {
out = new BufferedOutputStream(new FileOutputStream(blobFile));
IOUtils.copy(x, out);
} catch (IOException e) {
throw new SQLException(e.getMessage());
} finally {
IOUtils.closeQuietly(x);
IOUtils.closeQuietly(out);
}
connectionHttp.addLocalFile(blobFile);
connectionHttp.addRemoteFile(rawRemoteFileName);
// Ok. File is successfully uploaded!
// Set the parameter using the file name
InputStream inputStream = new AwakeInputStream(rawRemoteFileName);
// parameterValues.put(parameterIndex, inputStream);
callableStatementHolder.setParameter(parameterIndex, inputStream);
}
/**
* Sets the designated parameter to the given input stream, which will have
* the specified number of bytes. When a very large binary value is input to
* a LONGVARBINARY
parameter, it may be more practical to send
* it via a java.io.InputStream
object. The data will be read
* from the stream as needed until end-of-file is reached.
*
*
* Note: This stream object can either be a standard Java stream
* object or your own subclass that implements the standard interface.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the java input stream which contains the binary parameter
* value
* @param length
* the number of bytes in the stream
* @exception SQLException
* if parameterIndex does not correspond to a parameter
* marker in the SQL statement; if a database access error
* occurs or this method is called on a closed
* PreparedStatement
*/
@Override
public void setBinaryStream(int parameterIndex, java.io.InputStream x,
int length) throws SQLException {
testIfClosed();
this.setBinaryStream(parameterIndex, x);
}
/**
* Sets the designated parameter to the given input stream, which will have
* the specified number of bytes. When a very large binary value is input to
* a LONGVARBINARY
parameter, it may be more practical to send
* it via a java.io.InputStream
object. The data will be read
* from the stream as needed until end-of-file is reached.
*
*
* Note: This stream object can either be a standard Java stream
* object or your own subclass that implements the standard interface.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param x
* the java input stream which contains the binary parameter
* value
* @param length
* the number of bytes in the stream
* @exception SQLException
* if parameterIndex does not correspond to a parameter
* marker in the SQL statement; if a database access error
* occurs or this method is called on a closed
* PreparedStatement
* @since 1.6
*/
@Override
public void setBinaryStream(int parameterIndex, java.io.InputStream x,
long length) throws SQLException {
testIfClosed();
this.setBinaryStream(parameterIndex, x);
}
@Override
public void setAsciiStream(int parameterIndex, InputStream x, int length)
throws SQLException {
setAsciiStream(parameterIndex, x);
}
@Override
public void setAsciiStream(int parameterIndex, InputStream x, long length)
throws SQLException {
setAsciiStream(parameterIndex, x);
}
@Override
public void setAsciiStream(int parameterIndex, InputStream x)
throws SQLException {
testIfClosed();
if (!STREAMS_SUPPORTED) {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
// Create the file from the input Stream, naming it on the table name
FileNameFromBlobBuilder fileNameFromBlobBuilder = new FileNameFromBlobBuilder(
sql, parameterIndex, true);
String rawRemoteFileName = fileNameFromBlobBuilder.getFileName();
String dir = AwakeFileUtil.getAwakeTempDir();
File clobFile = new File(dir + rawRemoteFileName);
debug("rawRemoteFileName: " + rawRemoteFileName);
debug("blobFile : " + clobFile);
Writer writer = null;
try {
BufferedReader bufferedReader = new BufferedReader(
new InputStreamReader(x));
writer = new BufferedWriter(new FileWriter(clobFile));
String line = null;
while ((line = bufferedReader.readLine()) != null) {
if (connectionHttp.isHtmlEncodingOn()) {
line = HtmlConverter.toHtml(line);
}
writer.write(line + CR_LF);
}
} catch (IOException e) {
throw new SQLException(e.getMessage());
} finally {
IOUtils.closeQuietly(x);
IOUtils.closeQuietly(writer);
}
connectionHttp.addLocalFile(clobFile);
connectionHttp.addRemoteFile(rawRemoteFileName);
// Ok. File is successfully uploaded!
// Set the parameter using the file name
AwakeAsciiStream awakeAsciiStream = new AwakeAsciiStream(
rawRemoteFileName);
// parameterValues.put(parameterIndex, inputStream);
callableStatementHolder.setParameter(parameterIndex, awakeAsciiStream);
}
/**
* Sets the designated parameter to the given Reader
object.
* When a very large UNICODE value is input to a LONGVARCHAR
* parameter, it may be more practical to send it via a
* java.io.Reader
object. The data will be read from the stream
* as needed until end-of-file is reached. The JDBC driver will do any
* necessary conversion from UNICODE to the database char format.
*
*
* Note: This stream object can either be a standard Java stream
* object or your own subclass that implements the standard interface.
*
* Note: Consult your JDBC driver documentation to determine if it
* might be more efficient to use a version of
* setCharacterStream
which takes a length parameter.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param reader
* the java.io.Reader
object that contains the
* Unicode data
* @exception SQLException
* if parameterIndex does not correspond to a parameter
* marker in the SQL statement; if a database access error
* occurs or this method is called on a closed
* PreparedStatement
* @throws SQLFeatureNotSupportedException
* if the JDBC driver does not support this method
* @since 1.6
*/
@Override
public void setCharacterStream(int parameterIndex, java.io.Reader reader)
throws SQLException {
testIfClosed();
if (!STREAMS_SUPPORTED) {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
// Create the file from the input Stream, naming it on the table name
FileNameFromBlobBuilder fileNameFromBlobBuilder = new FileNameFromBlobBuilder(
sql, parameterIndex, true);
String rawRemoteFileName = fileNameFromBlobBuilder.getFileName();
String dir = AwakeFileUtil.getAwakeTempDir();
File clobFile = new File(dir + rawRemoteFileName);
debug("rawFileName: " + rawRemoteFileName);
debug("clobFile : " + clobFile);
BufferedReader bufferedReader = null;
Writer writer = null;
try {
bufferedReader = new BufferedReader(reader);
writer = new BufferedWriter(new FileWriter(clobFile));
String line = null;
while ((line = bufferedReader.readLine()) != null) {
if (connectionHttp.isHtmlEncodingOn()) {
line = HtmlConverter.toHtml(line);
}
writer.write(line + CR_LF);
}
} catch (IOException e) {
throw new SQLException(e.getMessage());
} finally {
IOUtils.closeQuietly(reader);
IOUtils.closeQuietly(writer);
}
connectionHttp.addLocalFile(clobFile);
connectionHttp.addRemoteFile(rawRemoteFileName);
// Ok. File is successfully uploaded!
// Set the parameter using the file name
Reader awakeReader = new AwakeReader(rawRemoteFileName);
// parameterValues.put(parameterIndex, awakeReader);
callableStatementHolder.setParameter(parameterIndex, awakeReader);
}
/**
* Sets the designated parameter to the given Reader
object,
* which is the given number of characters long. When a very large UNICODE
* value is input to a LONGVARCHAR
parameter, it may be more
* practical to send it via a java.io.Reader
object. The data
* will be read from the stream as needed until end-of-file is reached. The
* JDBC driver will do any necessary conversion from UNICODE to the database
* char format.
*
*
* Note: This stream object can either be a standard Java stream
* object or your own subclass that implements the standard interface.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param reader
* the java.io.Reader
object that contains the
* Unicode data
* @param length
* the number of characters in the stream
* @exception SQLException
* if parameterIndex does not correspond to a parameter
* marker in the SQL statement; if a database access error
* occurs or this method is called on a closed
* PreparedStatement
* @since 1.2
*/
@Override
public void setCharacterStream(int parameterIndex, java.io.Reader reader,
int length) throws SQLException {
testIfClosed();
this.setCharacterStream(parameterIndex, reader);
}
/**
* Sets the designated parameter to the given Reader
object,
* which is the given number of characters long. When a very large UNICODE
* value is input to a LONGVARCHAR
parameter, it may be more
* practical to send it via a java.io.Reader
object. The data
* will be read from the stream as needed until end-of-file is reached. The
* JDBC driver will do any necessary conversion from UNICODE to the database
* char format.
*
*
* Note: This stream object can either be a standard Java stream
* object or your own subclass that implements the standard interface.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, ...
* @param reader
* the java.io.Reader
object that contains the
* Unicode data
* @param length
* the number of characters in the stream
* @exception SQLException
* if parameterIndex does not correspond to a parameter
* marker in the SQL statement; if a database access error
* occurs or this method is called on a closed
* PreparedStatement
* @since 1.6
*/
@Override
public void setCharacterStream(int parameterIndex, java.io.Reader reader,
long length) throws SQLException {
testIfClosed();
this.setCharacterStream(parameterIndex, reader);
}
/**
* Clears the current parameter values immediately.
*
* In general, parameter values remain in force for repeated use of a
* statement. Setting a parameter value automatically clears its previous
* value. However, in some cases it is useful to immediately release the
* resources used by the current parameter values; this can be done by
* calling the method clearParameters
.
*
* @exception SQLException
* if a database access error occurs
*/
@Override
public void clearParameters() throws SQLException {
callableStatementHolder.clearParameters();
}
//
// Not (yet) implemented methods
//
@Override
public Array getArray(int parameterIndex) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Array getArray(String parameterName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public byte[] getBytes(int parameterIndex) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public byte[] getBytes(String parameterName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Reader getCharacterStream(int parameterIndex) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Reader getCharacterStream(String parameterName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Date getDate(int parameterIndex, Calendar cal) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Date getDate(String parameterName, Calendar cal) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Reader getNCharacterStream(int parameterIndex) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Reader getNCharacterStream(String parameterName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public NClob getNClob(int parameterIndex) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public NClob getNClob(String parameterName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Object getObject(int parameterIndex, Map> map)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Object getObject(String parameterName, Map> map)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Ref getRef(int parameterIndex) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Ref getRef(String parameterName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public RowId getRowId(int parameterIndex) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public RowId getRowId(String parameterName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public SQLXML getSQLXML(int parameterIndex) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public SQLXML getSQLXML(String parameterName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Time getTime(int parameterIndex) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Time getTime(String parameterName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Time getTime(int parameterIndex, Calendar cal) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Time getTime(String parameterName, Calendar cal) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Timestamp getTimestamp(int parameterIndex, Calendar cal)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public Timestamp getTimestamp(String parameterName, Calendar cal)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public URL getURL(int parameterIndex) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public URL getURL(String parameterName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setNString(String parameterName, String value)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setAsciiStream(String parameterName, InputStream x)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setAsciiStream(String parameterName, InputStream x, int length)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setAsciiStream(String parameterName, InputStream x, long length)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setBigDecimal(String parameterName, BigDecimal x)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setBinaryStream(String parameterName, InputStream x)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setBinaryStream(String parameterName, InputStream x, int length)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setBinaryStream(String parameterName, InputStream x, long length)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setBlob(String parameterName, Blob x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setBlob(String parameterName, InputStream inputStream)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setBlob(String parameterName, InputStream inputStream,
long length) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setBoolean(String parameterName, boolean x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setByte(String parameterName, byte x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setBytes(String parameterName, byte[] x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setCharacterStream(String parameterName, Reader reader)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setCharacterStream(String parameterName, Reader reader,
int length) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setCharacterStream(String parameterName, Reader reader,
long length) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setClob(String parameterName, Clob x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setClob(String parameterName, Reader reader)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setClob(String parameterName, Reader reader, long length)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setDate(String parameterName, Date x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setDate(String parameterName, Date x, Calendar cal)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setDouble(String parameterName, double x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setFloat(String parameterName, float x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setInt(String parameterName, int x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setLong(String parameterName, long x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setNCharacterStream(String parameterName, Reader value)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setNCharacterStream(String parameterName, Reader value,
long length) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setNClob(String parameterName, NClob value) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setNClob(String parameterName, Reader reader)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setNClob(String parameterName, Reader reader, long length)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setNull(String parameterName, int sqlType) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setNull(String parameterName, int sqlType, String typeName)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setObject(String parameterName, Object x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setObject(String parameterName, Object x, int targetSqlType)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setObject(String parameterName, Object x, int targetSqlType,
int scale) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setRowId(String parameterName, RowId x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setSQLXML(String parameterName, SQLXML xmlObject)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setShort(String parameterName, short x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setString(String parameterName, String x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setTime(String parameterName, Time x) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setTime(String parameterName, Time x, Calendar cal)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setTimestamp(String parameterName, Timestamp x)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setTimestamp(String parameterName, Timestamp x, Calendar cal)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void setURL(String parameterName, URL val) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public boolean wasNull() throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void registerOutParameter(String parameterName, int sqlType)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void registerOutParameter(int parameterIndex, int sqlType, int scale)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void registerOutParameter(int parameterIndex, int sqlType,
String typeName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void registerOutParameter(String parameterName, int sqlType,
int scale) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
@Override
public void registerOutParameter(String parameterName, int sqlType,
String typeName) throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
//
// Java 7 methods. For Java 6 / Java 7 Compatibility
//
/**
*
* Returns an object representing the value of OUT parameter
* {@code parameterIndex} and will convert from the SQL type of the
* parameter to the requested Java data type, if the conversion is
* supported. If the conversion is not supported or null is specified for
* the type, a SQLException
is thrown.
*
* At a minimum, an implementation must support the conversions defined in
* Appendix B, Table B-3 and conversion of appropriate user defined SQL
* types to a Java type which implements {@code SQLData}, or {@code Struct}.
* Additional conversions may be supported and are vendor defined.
*
* @param parameterIndex
* the first parameter is 1, the second is 2, and so on
* @param type
* Class representing the Java data type to convert the
* designated parameter to.
* @return an instance of {@code type} holding the OUT parameter value
* @throws SQLException
* if conversion is not supported, type is null or another error
* occurs. The getCause() method of the exception may provide a
* more detailed exception, for example, if a conversion error
* occurs
* @throws SQLFeatureNotSupportedException
* if the JDBC driver does not support this method
* @since 1.7
*/
public T getObject(int parameterIndex, Class type)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
/**
*
* Returns an object representing the value of OUT parameter
* {@code parameterName} and will convert from the SQL type of the parameter
* to the requested Java data type, if the conversion is supported. If the
* conversion is not supported or null is specified for the type, a
* SQLException
is thrown.
*
* At a minimum, an implementation must support the conversions defined in
* Appendix B, Table B-3 and conversion of appropriate user defined SQL
* types to a Java type which implements {@code SQLData}, or {@code Struct}.
* Additional conversions may be supported and are vendor defined.
*
* @param parameterName
* the name of the parameter
* @param type
* Class representing the Java data type to convert the
* designated parameter to.
* @return an instance of {@code type} holding the OUT parameter value
* @throws SQLException
* if conversion is not supported, type is null or another error
* occurs. The getCause() method of the exception may provide a
* more detailed exception, for example, if a conversion error
* occurs
* @throws SQLFeatureNotSupportedException
* if the JDBC driver does not support this method
* @since 1.7
*/
public T getObject(String pZarameterName, Class type)
throws SQLException {
throw new SQLFeatureNotSupportedException(ConnectionHttp.AWAKE_NOT_SUPPORTED_METHOD);
}
private static void debug(String s) {
if (DEBUG)
System.out.println(s);
}
}