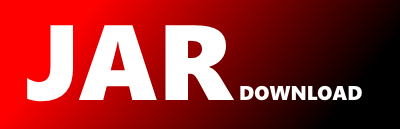
src-main.org.awakefw.sql.servlet.ConfiguratorMethodsTester Maven / Gradle / Ivy
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.servlet;
import java.io.File;
import java.util.List;
import java.util.Set;
import org.awakefw.commons.api.server.AwakeCommonsConfigurator;
import org.awakefw.commons.server.util.AwakeServerLogger;
import org.awakefw.file.api.server.AwakeFileConfigurator;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.servlet.AwakeCommonsConfiguratorCall;
import org.awakefw.file.servlet.ServerUserException;
/**
* Tests that all configurators methods are correct. set properties if not, with
* Exception & associated message.
*
* @author Nicolas de Pomereu
*
*/
public class ConfiguratorMethodsTester {
private static boolean DEBUG = AwakeDebug.isSet(ConfiguratorMethodsTester.class);
private AwakeCommonsConfigurator awakeCommonsConfigurator = null;
private AwakeFileConfigurator awakeFileConfigurator = null;
/** The Exception */
private Exception exception = null;
/** The init error message trapped */
private String initErrrorMesage = null;
/**
* Constructor
* @param awakeCommonsConfigurator
* @param awakeFileConfigurator
*/
public ConfiguratorMethodsTester(
AwakeCommonsConfigurator awakeCommonsConfigurator,
AwakeFileConfigurator awakeFileConfigurator) {
this.awakeCommonsConfigurator = awakeCommonsConfigurator;
this.awakeFileConfigurator = awakeFileConfigurator;
}
/**
*
* @return the Exception thrown
*/
public Exception getException() {
return exception;
}
/**
*
* @return the the label of the exception thrown
*/
public String getInitErrrorMesage() {
return initErrrorMesage;
}
/**
* Test the configurators main methods to see if they throw Exceptions
*/
public void testMethods() {
// Fist thing to do: Stores in static memory the user settings for this
// transaction
// This method will also test the Connection is created, otw a
// detailed Exception is thrown
debug("initErrrorMesage: " + initErrrorMesage);
debug("Before awakeCommonsConfigurator.login.");
if (exception == null) {
// Test that the login method does not throw an Exception
@SuppressWarnings("unused")
boolean isOk = false;
try {
debug("In awakeCommonsConfigurator.login.");
isOk = awakeCommonsConfigurator.login("dummy",
"dummy".toCharArray());
debug("After new awakeCommonsConfigurator.login.");
} catch (Exception e) {
debug("Exception thrown: " + e.toString());
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "login");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
File serverRootFile = awakeFileConfigurator.getServerRoot();
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "getServerRoot");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
boolean oneRooPerUserName = awakeFileConfigurator
.useOneRootPerUsername();
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "useOneRootPerUsername");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
String tokenRecomputed = AwakeCommonsConfiguratorCall
.computeAuthToken(awakeCommonsConfigurator, "dummy");
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "getServerRoot");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
boolean forceHttps = AwakeCommonsConfiguratorCall
.forceSecureHttp(awakeCommonsConfigurator);
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "forceSecureHttp");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
Set usernameSet = AwakeCommonsConfiguratorCall
.getBannedUsernames(awakeCommonsConfigurator);
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "getBannedUsernames");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
List usernameSet = AwakeCommonsConfiguratorCall
.getBannedIPs(awakeCommonsConfigurator);
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "getBannedIPs");
exception = e;
}
}
if (exception == null) {
try {
@SuppressWarnings("unused")
char[] encryptionPassword = AwakeCommonsConfiguratorCall
.getEncryptionPassword(awakeCommonsConfigurator);
} catch (Exception e) {
initErrrorMesage = ServerUserException.getErrorMessage(
awakeCommonsConfigurator, "getEncryptionPassword");
exception = e;
}
}
}
/**
* debug
*/
public static void debug(String s) {
if (DEBUG) {
try {
AwakeServerLogger.log(s);
} catch (Exception e1) {
System.out.println(s);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy