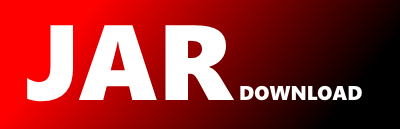
src-main.org.awakefw.sql.servlet.DatabaseMetaDataSpecial Maven / Gradle / Ivy
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.servlet;
import java.sql.DatabaseMetaData;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.List;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.util.AwakeClientLogger;
import org.awakefw.sql.json.IntArrayTransport;
import org.awakefw.sql.json.StringArrayTransport;
/**
*
* Dedicated executor for DatabaseMetaData.getTables() &
* DatabaseMetaData.getUDTs() that have special array String[] or int[]
* parameters.
*
* @author Nicolas de Pomereu
*/
public class DatabaseMetaDataSpecial {
/** Set to true to display/log debug info */
private static boolean DEBUG = AwakeDebug
.isSet(DatabaseMetaDataSpecial.class);
/** DatabaseMetaData instance */
private DatabaseMetaData databaseMetaData = null;
/* the DatabaseMetaData special method to execute */
private String methodName = null;
/* the parameters of the special method to execute */
private List listParamsValues = null;
/**
*
* @param databaseMetaData
* DatabaseMetaData instance
* @param methodName
* the DatabaseMetaData special method to execute
* @param listParamsValues
* the parameters of the special method to execute
*/
public DatabaseMetaDataSpecial(DatabaseMetaData databaseMetaData,
String methodName, List listParamsValues) {
if (databaseMetaData == null) {
throw new IllegalArgumentException(
"databaseMetaData can not be null!");
}
if (methodName == null) {
throw new IllegalArgumentException("methodName can not be null!");
}
if (listParamsValues == null) {
throw new IllegalArgumentException(
"listParamsValues can not be null!");
}
this.databaseMetaData = databaseMetaData;
this.methodName = methodName;
this.listParamsValues = listParamsValues;
}
/**
* Execute the special method
*
* @return the Result set of DatabaseMetaData.getTables or
* DatabaseMetaData.getUDTs
* @throws SQLException
*/
public ResultSet execute() throws SQLException {
ResultSet rs = null;
if (methodName.equals("getTables")) {
rs = executeGetTables();
} else if (methodName.equals("getUDTs")) {
rs = executeGetUDTs();
} else if (methodName.equals("getPrimaryKeys")) {
rs = executeGetPrimaryKeys();
} else {
throw new IllegalArgumentException(
"Invalid DatabaseMetaData method name. "
+ "Must be \"getTables\" or \"getUDTs\" or \"getPrimaryKeys\": " + methodName);
}
return rs;
}
/**
* Execute DatabaseMetaData.getTables
*
* @return the Result set
* @throws SQLException
*/
private ResultSet executeGetTables() throws SQLException {
// Method prototype:
// ResultSet getTables(String catalog, String schemaPattern,
// String tableNamePattern, String types[]) throws SQLException;
String catalog = listParamsValues.get(0);
String schemaPattern = listParamsValues.get(1);
String tableNamePattern = listParamsValues.get(2);
String jsonString = listParamsValues.get(3);
debug("catalog :" + catalog + ":");
debug("schemaPattern :" + schemaPattern + ":");
debug("tableNamePattern :" + tableNamePattern + ":");
debug("jsonString :" + jsonString + ":");
//String[] types = CallMetaDataTransport.fromJsonStringArray(jsonString);
String[] types = StringArrayTransport.fromJson(jsonString);
// Detect null transported values
if (catalog.equals("NULL"))
catalog = null;
if (schemaPattern.equals("NULL"))
schemaPattern = null;
if (tableNamePattern.equals("NULL"))
tableNamePattern = null;
if (types.length == 1 && types[0] == "NULL")
types = null;
ResultSet rsMetaTables = databaseMetaData.getTables(catalog,
schemaPattern, tableNamePattern, types);
return rsMetaTables;
}
/**
* Execute DatabaseMetaData.getUDTs
*
* @return the Result set
* @throws SQLException
*/
private ResultSet executeGetUDTs() throws SQLException {
// Method prototype:
// ResultSet getUDTs(String catalog, String schemaPattern,
// String typeNamePattern, int[] types)
// throws SQLException;
String catalog = listParamsValues.get(0);
String schemaPattern = listParamsValues.get(1);
String tableNamePattern = listParamsValues.get(2);
String jsonString = listParamsValues.get(3);
//int[] types = CallMetaDataTransport.fromJsonIntArray(jsonString);
int[] types = IntArrayTransport.fromJson(jsonString);
// Detect null transported values
if (catalog.equals("NULL"))
catalog = null;
if (schemaPattern.equals("NULL"))
schemaPattern = null;
if (tableNamePattern.equals("NULL"))
tableNamePattern = null;
if (types.length == 1 && types[0] == -999)
types = null;
ResultSet rs = databaseMetaData.getUDTs(catalog, schemaPattern,
tableNamePattern, types);
return rs;
}
/**
* Execute DatabaseMetaData.executeGetPrimaryKeys
*
* @return the Result set
* @throws SQLException
*/
private ResultSet executeGetPrimaryKeys() throws SQLException {
// Method prototype:
// ResultSet getUDTs(String catalog, String schemaPattern,
// String typeNamePattern, int[] types)
// throws SQLException;
String catalog = listParamsValues.get(0);
String schemaPattern = listParamsValues.get(1);
String tableNamePattern = listParamsValues.get(2);
//catalog = HtmlConverter.fromHtml(catalog);
//schemaPattern = HtmlConverter.fromHtml(schemaPattern);
//tableNamePattern = HtmlConverter.fromHtml(tableNamePattern);
// Detect null transported values
if (catalog.equals("NULL"))
catalog = null;
if (schemaPattern.equals("NULL"))
schemaPattern = null;
if (tableNamePattern.equals("NULL"))
tableNamePattern = null;
ResultSet rs = databaseMetaData.getPrimaryKeys(catalog, schemaPattern,
tableNamePattern);
return rs;
}
/**
* Method called by children Servlest for debug purpose Println is done only
* if class name name is in awake-debug.ini
*/
public static void debug(String s) {
if (DEBUG) {
AwakeClientLogger.log(s);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy