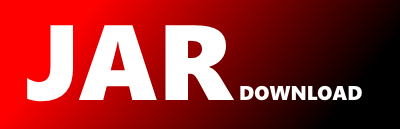
src-main.org.awakefw.sql.servlet.sql.ServerStatementRawExecute Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.servlet.sql;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.Map;
import java.util.Vector;
import java.util.logging.Level;
import javax.servlet.http.HttpServletRequest;
import org.apache.commons.io.IOUtils;
import org.awakefw.commons.api.server.AwakeCommonsConfigurator;
import org.awakefw.commons.server.util.AwakeServerLogger;
import org.awakefw.file.api.server.AwakeFileConfigurator;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.util.AwakeFileUtil;
import org.awakefw.file.util.Tag;
import org.awakefw.file.util.TransferStatus;
import org.awakefw.sql.api.server.AwakeSqlConfigurator;
import org.awakefw.sql.json.StatementHolder;
import org.awakefw.sql.servlet.AwakeSqlConfiguratorCall;
/**
* @author KawanSoft S.A.S
*
* Allows to execute the Statement or Prepared Statement on the Server
* as raw execute()
*/
public class ServerStatementRawExecute {
private static boolean DEBUG = AwakeDebug
.isSet(ServerStatementRawExecute.class);
public static String CR_LF = System.getProperty("line.separator");
private AwakeCommonsConfigurator awakeCommonsConfigurator = null;
/** The File Configurator (for Blobs/Clobs) */
private AwakeFileConfigurator awakeFileConfigurator = null;
private AwakeSqlConfigurator awakeSqlConfigurator;
private Connection connection;
private String username;
private StatementHolder statementHolder;
/** The http request */
private HttpServletRequest request;
/**
* Default Constructor
*
* @param request
* the http request
* @param awakeCommonsConfigurator
* the commons file configurator (for encryption)
* @param awakeFileConfigurator
* the FileConfigurator (for blobs/clobs)
* @param awakeSqlConfigurator
* the SqlConfigurator (for security check)
* @param connection
* the Jdbc connection
* @param username
* the client username
* @param sqlOrderAndParmsStore
* the Sql order and parms
*/
public ServerStatementRawExecute(HttpServletRequest request,
AwakeCommonsConfigurator awakeCommonsConfigurator,
AwakeFileConfigurator awakeFileConfigurator,
AwakeSqlConfigurator awakeSqlConfigurator, Connection connection,
String username, StatementHolder statementHolder)
throws SQLException {
if (awakeCommonsConfigurator == null) {
throw new IllegalArgumentException(Tag.AWAKE_PRODUCT_FAIL
+ "awakeCommonsConfigurator can not be null!");
}
if (awakeSqlConfigurator == null) {
throw new IllegalArgumentException(Tag.AWAKE_PRODUCT_FAIL
+ "awakeSqlConfigurator can not be null!");
}
if (connection == null) {
throw new IllegalArgumentException(Tag.AWAKE_PRODUCT_FAIL
+ "SQL Connection can not be null!");
}
if (username == null) {
throw new IllegalArgumentException(Tag.AWAKE_PRODUCT_FAIL
+ "username can not be null!");
}
if (statementHolder == null) {
throw new IllegalArgumentException(Tag.AWAKE_PRODUCT_FAIL
+ "statementHolder can not be null!");
}
this.awakeCommonsConfigurator = awakeCommonsConfigurator;
this.awakeFileConfigurator = awakeFileConfigurator;
this.awakeSqlConfigurator = awakeSqlConfigurator;
this.connection = connection;
this.username = username;
this.statementHolder = statementHolder;
this.request = request;
}
/**
* Execute the query/update for the statement and return the result (or
* result set) as as string
*
* @throws SQLException
*
* @return the temp file containing the result set output, null if no result
* set is produced
*/
public File execute(File tempFileForResultSet) throws SQLException,
IOException {
// File tempFileForResultSet = createTempFileForResultSet();
BufferedWriter br = null;
try {
br = new BufferedWriter(new FileWriter(tempFileForResultSet));
// Execute it
if (statementHolder.isPreparedStatement()) {
executePrepStatement(br);
} else {
executeStatement(br);
}
IOUtils.closeQuietly(br);
return tempFileForResultSet;
} finally {
IOUtils.closeQuietly(br);
}
}
/**
* Execute the passed SQL PreparedStatement as execute() sand return:
* - The result set as a List of Maps for SELECT statements.
* - The return code for other statements
*
* @param sqlOrder
* the qsql order
* @param sqlParms
* the sql parameters
* @param br
* the writer where to write to result set output
*
*
* @throws SQLException
*/
private void executePrepStatement(BufferedWriter br) throws SQLException,
IOException {
String sqlOrder = statementHolder.getSqlOrder();
PreparedStatement preparedStatement = null;
boolean usesAutoGeneratedKeys = false;
if (statementHolder.getAutoGeneratedKeys() != -1) {
preparedStatement = connection.prepareStatement(sqlOrder,
statementHolder.getAutoGeneratedKeys());
usesAutoGeneratedKeys = true;
} else if (statementHolder.getColumnIndexesAutogenerateKeys().length != 0) {
preparedStatement = connection.prepareStatement(sqlOrder,
statementHolder.getColumnIndexesAutogenerateKeys());
usesAutoGeneratedKeys = true;
} else if (statementHolder.getColumnNamesAutogenerateKeys().length != 0) {
preparedStatement = connection.prepareStatement(sqlOrder,
statementHolder.getColumnNamesAutogenerateKeys());
usesAutoGeneratedKeys = true;
} else {
preparedStatement = connection.prepareStatement(sqlOrder);
}
Map parameterTypes = null;
Map parameterStringValues = null;
// Class to set all the statement parameters
ServerPreparedStatementParameters serverPreparedStatementParameters = null;
try {
ServerSqlUtil.setStatementProperties(preparedStatement,
statementHolder);
parameterTypes = statementHolder.getParameterTypes();
parameterStringValues = statementHolder.getParameterStringValues();
if (!AwakeSqlConfiguratorCall.allowExecute(awakeSqlConfigurator,
username, connection)) {
String ipAddress = request.getRemoteAddr();
AwakeSqlConfiguratorCall.runIfStatementRefused(
awakeSqlConfigurator, username, connection, ipAddress,
sqlOrder, new Vector
© 2015 - 2025 Weber Informatics LLC | Privacy Policy