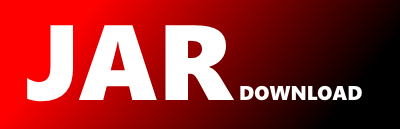
src-main.org.awakefw.sql.jdbc.ResultSetMetaDataHttp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.jdbc;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import org.awakefw.commons.jdbc.abstracts.AbstractResultSetMetaData;
import org.awakefw.file.util.Tag;
import org.awakefw.sql.json.no_obfuscation.ResultSetMetaDataHolder;
/**
* ResultSetMetaData Wrapper.
* Implements all the ResultSetMetaData methods. Usage is exactly the same as a
* ResultSetMetaData.
*
*/
public class ResultSetMetaDataHttp extends AbstractResultSetMetaData implements ResultSetMetaData {
/** the holder that contain all ResultSetMetaData infos */
private ResultSetMetaDataHolder resultSetMetaDataHolder = null;
/**
* Constructor
*
* @param resultSetMetaDataHolder
* the holder that contain all ResultSetMetaData info
*/
public ResultSetMetaDataHttp(ResultSetMetaDataHolder resultSetMetaDataHolder) {
if (resultSetMetaDataHolder == null) {
throw new IllegalArgumentException(Tag.AWAKE_PRODUCT_FAIL +
"resultSetMetaDataHolder can not be null!");
}
this.resultSetMetaDataHolder = resultSetMetaDataHolder;
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getColumnCount()
*/
@Override
public int getColumnCount() throws SQLException {
return this.resultSetMetaDataHolder.getColumnCount();
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#isAutoIncrement(int)
*/
@Override
public boolean isAutoIncrement(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getAutoIncrement().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getAutoIncrement().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#isCaseSensitive(int)
*/
@Override
public boolean isCaseSensitive(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getCaseSensitive().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getCaseSensitive().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#isSearchable(int)
*/
@Override
public boolean isSearchable(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getSearchable().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getSearchable().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#isCurrency(int)
*/
@Override
public boolean isCurrency(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getCurrency().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getCurrency().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#isNullable(int)
*/
@Override
public int isNullable(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getNullable().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getNullable().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#isSigned(int)
*/
@Override
public boolean isSigned(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getSigned().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getSigned().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getColumnDisplaySize(int)
*/
@Override
public int getColumnDisplaySize(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getColumnDisplaySize().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getColumnDisplaySize().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getColumnLabel(int)
*/
@Override
public String getColumnLabel(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getColumnLabel().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getColumnLabel().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getColumnName(int)
*/
@Override
public String getColumnName(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getColumnName().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getColumnName().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getSchemaName(int)
*/
@Override
public String getSchemaName(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getSchemaName().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getSchemaName().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getPrecision(int)
*/
@Override
public int getPrecision(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getPrecision().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getPrecision().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getScale(int)
*/
@Override
public int getScale(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getScale().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getScale().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getTableName(int)
*/
@Override
public String getTableName(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getTableName().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getTableName().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getCatalogName(int)
*/
@Override
public String getCatalogName(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getCatalogName().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getCatalogName().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getColumnType(int)
*/
@Override
public int getColumnType(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getColumnType().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getColumnType().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getColumnTypeName(int)
*/
@Override
public String getColumnTypeName(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getColumnTypeName().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getColumnTypeName().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#isReadOnly(int)
*/
@Override
public boolean isReadOnly(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getReadOnly().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getReadOnly().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#isWritable(int)
*/
@Override
public boolean isWritable(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getWritable().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getWritable().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#isDefinitelyWritable(int)
*/
@Override
public boolean isDefinitelyWritable(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getDefinitelyWritable().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getDefinitelyWritable().size());
}
}
/*
* (non-Javadoc)
*
* @see java.sql.ResultSetMetaData#getColumnClassName(int)
*/
@Override
public String getColumnClassName(int column) throws SQLException {
try {
return resultSetMetaDataHolder.getColumnClassName().get(column - 1);
} catch (IndexOutOfBoundsException e) {
throw new SQLException("Column index is out of bounds: "
+ column + ". Number of columns: " + resultSetMetaDataHolder.getColumnClassName().size());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy