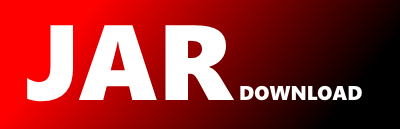
src-main.org.awakefw.sql.jdbc.http.JdbcHttpConnectionInfoTransfer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.jdbc.http;
import java.sql.Array;
import java.sql.SQLClientInfoException;
import java.sql.SQLException;
import java.sql.Types;
import java.util.List;
import java.util.Properties;
import java.util.Vector;
import org.apache.http.message.BasicNameValuePair;
import org.awakefw.commons.api.client.InvalidLoginException;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.api.util.HtmlConverter;
import org.awakefw.file.http.HttpTransfer;
import org.awakefw.file.http.HttpTransferOne;
import org.awakefw.file.util.AwakeClientLogger;
import org.awakefw.file.util.parms.Parameter;
import org.awakefw.file.util.parms.ReturnCode;
import org.awakefw.sql.api.client.InvalidatedSessionException;
import org.awakefw.sql.jdbc.ConnectionHttp;
import org.awakefw.sql.json.ObjectArrayTransport;
import org.awakefw.sql.json.PropertiesTransportGson;
import org.awakefw.sql.transport.ArrayHttp;
import org.awakefw.sql.util.ConnectionParms;
import org.awakefw.sql.util.SqlAction;
import org.awakefw.sql.util.SqlReturnCode;
/**
*
* For all Connection Info requests...
*
*/
public class JdbcHttpConnectionInfoTransfer {
/** Set to true to display/log debug info */
private static boolean DEBUG = AwakeDebug
.isSet(JdbcHttpConnectionInfoTransfer.class);
/** The Http Connection */
private ConnectionHttp connectionHttp = null;
/** The Authentication Token */
private String authenticationToken = null;
/**
* Protected constructor, not callable
*/
protected JdbcHttpConnectionInfoTransfer() {
}
/**
* Constructor
*
* @param connectionHttp
* the http Connection
* @param authenticationToken
* the Http Connection Authentication Token
*/
public JdbcHttpConnectionInfoTransfer(ConnectionHttp connectionHttp,
String authenticationToken) {
this.connectionHttp = connectionHttp;
this.authenticationToken = authenticationToken;
}
/*
* isValid(int timeout) setClientInfo(String name, String value)
* setClientInfo(Properties properties) getClientInfo(String name)
* getClientInfo()
*/
/**
* Returns true if the connection has not been closed and is still valid
*
* @return true if the connection has not been closed and is still valid
*/
public boolean isValid(int timeout) throws SQLException {
String isValidStr = null;
isValidStr = setConnectionInfoAction(SqlAction.ACTION_SQL_IS_VALID,
ConnectionParms.TIMEOUT, timeout + "");
boolean isValid = Boolean.parseBoolean(isValidStr);
return isValid;
}
/**
* setClientInfo
*/
public void setClientInfo(String name, String value)
throws SQLClientInfoException {
name = HtmlConverter.toHtml(name);
value = HtmlConverter.toHtml(value);
try {
setConnectionInfoActionTwoParms(
SqlAction.ACTION_SQL_SET_CLIENT_INFO_NAME,
ConnectionParms.NAME, name, ConnectionParms.VALUE, value);
} catch (SQLException e) {
throw new SQLClientInfoException(e.getMessage(), null, e);
}
}
/**
* setClientInfo
*/
public void setClientInfo(Properties prop) throws SQLClientInfoException {
String jsonString = PropertiesTransportGson.toJson(prop);
jsonString = HtmlConverter.toHtml(jsonString);
try {
setConnectionInfoAction(SqlAction.ACTION_SQL_SET_CLIENT_INFO_PROP,
ConnectionParms.PROPERTIES, jsonString);
} catch (SQLException e) {
throw new SQLClientInfoException(e.getMessage(), null, e);
}
}
/**
* getClientInfo
*/
public String getClientInfo(String name) throws SQLException {
String clientInfo = setConnectionInfoAction(
SqlAction.ACTION_SQL_GET_CLIENT_INFO_NAME,
ConnectionParms.NAME, name);
clientInfo = HtmlConverter.fromHtml(clientInfo);
return clientInfo;
}
/**
* getClientInfo
*/
public Properties getClientInfo() throws SQLException {
String jsonString = setConnectionInfoAction(
SqlAction.ACTION_SQL_GET_CLIENT_INFO,
ConnectionParms.NO_PARM, "NO_PARM");
jsonString = HtmlConverter.fromHtml(jsonString);
Properties prop = PropertiesTransportGson.fromJson(jsonString);
return prop;
}
/**
* send an action to execute a server Connection.createArrayOf()
* @param typeName
* @param elements
* @return
* @throws SQLException
*/
public Array createArrayOf(String typeName, Object[] elements) throws SQLException {
String jsonString = ObjectArrayTransport.toJson(elements);
jsonString = HtmlConverter.toHtml(jsonString);
String ArrayId = setConnectionInfoActionTwoParms(SqlAction.ACTION_SQL_CREATE_ARRAY_OF, ConnectionParms.TYPENAME, typeName, ConnectionParms.ELEMENTS, jsonString);
ArrayHttp arrayHttp = new ArrayHttp(ArrayId, typeName, Types.VARCHAR, elements);
return arrayHttp;
}
/**
* Sets a Connection info action
*
* @param action
* the action
* @param parm
* the parm name
* @param value
* the parm value
*
* @throws SQLException
*/
private String setConnectionInfoAction(String action, String parm,
String value) throws SQLException {
// Launch the Servlet
HttpTransfer httpTransfer = new HttpTransferOne(
connectionHttp.getUrl(), connectionHttp.getHttpProxy(),
connectionHttp.getHttpProtocolParameters(),
connectionHttp.getAwakeProgressManager());
List requestParams = new Vector();
requestParams.add(new BasicNameValuePair(Parameter.ACTION, action));
requestParams.add(new BasicNameValuePair(Parameter.USERNAME,
connectionHttp.getUsername()));
requestParams.add(new BasicNameValuePair(Parameter.TOKEN,
this.authenticationToken));
requestParams.add(new BasicNameValuePair(
ConnectionParms.CONNECTION_ID, connectionHttp
.getConnectionId()));
requestParams.add(new BasicNameValuePair(parm, value));
try {
httpTransfer.send(requestParams);
} catch (Exception e) {
JdbcHttpTransferUtil.wrapExceptionAsSQLException(e);
}
String receive = httpTransfer.recv();
receive = receive.trim();
debug("httpTransfer.recv(): " + receive + ":");
if (receive.startsWith(ReturnCode.INVALID_LOGIN_OR_PASSWORD)) {
throw new SQLException(new InvalidLoginException());
}
if (receive.startsWith(SqlReturnCode.SESSION_INVALIDATED)) {
throw new SQLException(new InvalidatedSessionException());
}
return receive;
}
/**
* Sets a Connection info action with two parameters
*
* @param action
* the action
* @param parm1
* the parm name
* @param value1
* the parm value
*
* @throws SQLException
*/
private String setConnectionInfoActionTwoParms(String action, String parm1,
String value1, String parm2, String value2) throws SQLException {
// Launch the Servlet
HttpTransfer httpTransfer = new HttpTransferOne(
connectionHttp.getUrl(), connectionHttp.getHttpProxy(),
connectionHttp.getHttpProtocolParameters(),
connectionHttp.getAwakeProgressManager());
List requestParams = new Vector();
requestParams.add(new BasicNameValuePair(Parameter.ACTION, action));
requestParams.add(new BasicNameValuePair(Parameter.USERNAME,
connectionHttp.getUsername()));
requestParams.add(new BasicNameValuePair(Parameter.TOKEN,
this.authenticationToken));
requestParams.add(new BasicNameValuePair(
ConnectionParms.CONNECTION_ID, connectionHttp
.getConnectionId()));
requestParams.add(new BasicNameValuePair(parm1, value1));
requestParams.add(new BasicNameValuePair(parm2, value2));
try {
httpTransfer.send(requestParams);
} catch (Exception e) {
JdbcHttpTransferUtil.wrapExceptionAsSQLException(e);
}
String receive = httpTransfer.recv();
receive = receive.trim();
debug("httpTransfer.recv(): " + receive + ":");
if (receive.startsWith(ReturnCode.INVALID_LOGIN_OR_PASSWORD)) {
throw new SQLException(new InvalidLoginException());
}
if (receive.startsWith(SqlReturnCode.SESSION_INVALIDATED)) {
throw new SQLException(new InvalidatedSessionException());
}
return receive;
}
// One case with two parameters
/**
* Debug tool
*
* @param s
*/
// @SuppressWarnings("unused")
private void debug(String s) {
if (DEBUG) {
AwakeClientLogger.log(s);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy