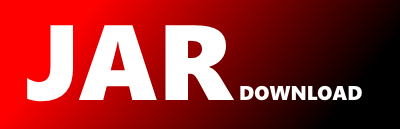
src-main.org.awakefw.sql.jdbc.http.JdbcHttpMetaDataTransfer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.jdbc.http;
import java.io.File;
import java.sql.SQLException;
import java.util.List;
import java.util.Vector;
import org.apache.http.message.BasicNameValuePair;
import org.awakefw.commons.api.client.InvalidLoginException;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.http.HttpTransfer;
import org.awakefw.file.http.HttpTransferOne;
import org.awakefw.file.json.ListOfStringTransport;
import org.awakefw.file.util.AwakeClientLogger;
import org.awakefw.file.util.AwakeFileUtil;
import org.awakefw.file.util.KeepTempFilePolicyParms;
import org.awakefw.file.util.Tag;
import org.awakefw.file.util.parms.Parameter;
import org.awakefw.file.util.parms.ReturnCode;
import org.awakefw.sql.api.client.InvalidatedSessionException;
import org.awakefw.sql.jdbc.ConnectionHttp;
import org.awakefw.sql.json.IntArrayTransport;
import org.awakefw.sql.json.StringArrayTransport;
import org.awakefw.sql.util.ConnectionParms;
import org.awakefw.sql.util.SqlAction;
import org.awakefw.sql.util.SqlReturnCode;
/**
*
* Class that execute all http transfer to the server, and gets back the results
*
*/
public class JdbcHttpMetaDataTransfer {
/** Set to true to display/log debug info */
private static boolean DEBUG = AwakeDebug
.isSet(JdbcHttpMetaDataTransfer.class);
/** The Http Connection */
private ConnectionHttp connectionHttp = null;
/** The Authentication Token */
private String authenticationToken = null;
/**
* Protected constructor, not callable
*/
protected JdbcHttpMetaDataTransfer() {
}
/**
* Constructor
*
* @param connectionHttp
* the http Connection
* @param authenticationToken
* the Http Connection Authentication Token
*/
public JdbcHttpMetaDataTransfer(ConnectionHttp connectionHttp,
String authenticationToken) {
this.connectionHttp = connectionHttp;
this.authenticationToken = authenticationToken;
}
/**
* Calls a remote DatabaseMetaData.method and (eventually) pass some
* parameters to it.
*
* @param methodName
* the raw DatabaseMetaData.method name to call in the format
* myMethod
(no package and class info)
* @param params
* the array of parameters passed to the method
* @return the result of the Java call as File
*
* @throws IllegalArgumentException
* if methodName is null
* @throws InvalidLoginException
* if the username is refused by the remote host
* @throws SQLException
* if the any SQL Exception occurs on host
*
*/
public File getFileFromCallMetaDataFunction(String methodName,
Object... params) throws SQLException {
// Class and method name can not be null
if (methodName == null) {
throw new IllegalArgumentException("methodName can not be null!");
}
// Build the params types
List paramsTypes = new Vector();
// Build the params values
List paramsValues = new Vector();
debug("");
for (int i = 0; i < params.length; i++) {
JdbcHttpMetaDataTransfer.detectNullForSpecialMethods(methodName, i,
params);
if (params[i] == null) {
debug(Tag.AWAKE
+ "null values are not supported for method: "
+ methodName
+ " param: "
+ (i + 1)
+ ". Param is *supposed* to be String and value forced to \"NULL\".");
params[i] = new String("NULL");
}
String classType = params[i].getClass().getName();
// NO! can alter class name if value is obsfucated
// classType = StringUtils.substringAfterLast(classType, ".");
paramsTypes.add(classType);
String value = null;
// For DatabaseMetaData.getTables() & DatabaseMetaData.getUDTs()
if (classType.equals("[Ljava.lang.String;")
|| classType.equals("[I")) {
// Gson gson = new Gson();
// value = gson.toJson(params[i]);
//value = CallMetaDataTransport.toJson(params[i], classType);
if (classType.equals("[Ljava.lang.String;")) {
String[] stringArray = (String[]) params[i];
value = StringArrayTransport.toJson(stringArray);
} else { // classType.equals("[I")
int[] intArray = (int[]) params[i];
value = IntArrayTransport.toJson(intArray);
}
debug("Array value:" + value);
} else {
value = params[i].toString();
}
debug("");
debug("classType: " + classType);
debug("value : " + value);
//value = HtmlConverter.toHtml(value);
paramsValues.add(value);
}
// ListHolder listHolderTypes = new ListHolder();
// listHolderTypes.setList(paramsTypes);
String jsonParamTypes = ListOfStringTransport.toJson(paramsTypes);
// ListHolder listHolderValues = new ListHolder();
// listHolderValues.setList(paramsValues);
String jsonParamValues = ListOfStringTransport.toJson(paramsValues);
debug("methodName : " + methodName);
debug("jsonParamTypes : " + jsonParamTypes);
debug("jsonParamValues: " + jsonParamValues);
HttpTransfer httpTransfer = null;
httpTransfer = new HttpTransferOne(connectionHttp.getUrl(),
connectionHttp.getHttpProxy(),
connectionHttp.getHttpProtocolParameters(),
connectionHttp.getAwakeProgressManager());
String username = connectionHttp.getUsername();
// Prepare the request parameters
List requestParams = new Vector();
requestParams.add(new BasicNameValuePair(Parameter.ACTION,
SqlAction.ACTION_SQL_GET_METADATA));
requestParams.add(new BasicNameValuePair(Parameter.USERNAME, username));
requestParams.add(new BasicNameValuePair(Parameter.TOKEN,
authenticationToken));
requestParams.add(new BasicNameValuePair(Parameter.METHOD_NAME,
methodName));
requestParams.add(new BasicNameValuePair(Parameter.PARAMS_TYPES,
jsonParamTypes));
requestParams.add(new BasicNameValuePair(Parameter.PARAMS_VALUES,
jsonParamValues));
requestParams.add(new BasicNameValuePair(ConnectionParms.CONNECTION_ID,
connectionHttp.getConnectionId() ));
httpTransfer.setReceiveInFile(true); // To say we get the result into a
File receiveFile = null;
try {
httpTransfer.send(requestParams);
receiveFile = httpTransfer.getReceiveFile();
String receive = AwakeFileUtil.getFirstLineOfFile(receiveFile);
debug("httpTransfer.getReceiveFile(): " + receiveFile + ":");
if (receive.startsWith(ReturnCode.INVALID_LOGIN_OR_PASSWORD)) {
if (!DEBUG && !KeepTempFilePolicyParms.KEEP_TEMP_FILE) {
receiveFile.delete();
}
throw new InvalidLoginException();
}
if (receive.startsWith(SqlReturnCode.SESSION_INVALIDATED)) {
throw new SQLException(new InvalidatedSessionException());
}
} catch (Exception e) {
JdbcHttpTransferUtil.wrapExceptionAsSQLException(e);
}
// We are done: return the received string
return receiveFile;
}
/**
* Debug tool
*
* @param s
*/
// @SuppressWarnings("unused")
private void debug(String s) {
if (DEBUG) {
AwakeClientLogger.log(s);
}
}
/**
* Detect null values for two special methods, and replace them by
* functionnal nulls
*
* @param methodName
* the method name : getTables or getUDTs
* @param i
* the index of the param in the method
* @param params
* the params aray
*/
private static void detectNullForSpecialMethods(String methodName, int i,
Object... params) {
// Modify null parameters
if (methodName.equals("getTables") || methodName.equals("getUDTs")) {
// The 3 first parameters are String
if (i < 3 && params[i] == null) {
params[i] = new String("NULL");
}
// The 4th is String[] for types
if (i == 3 && params[i] == null && methodName.equals("getTables")) {
String[] stringArray = new String[1];
stringArray[0] = "NULL";
params[i] = stringArray;
}
// The 4th is int[] for types
if (i == 3 && params[i] == null && methodName.equals("getUDTs")) {
int[] intArray = new int[1];
intArray[0] = -999; // says -999 for null
params[i] = intArray;
}
}
// //getPrimaryKeys
// if (methodName.equals("getPrimaryKeys")) {
// if (i < 2 && params[i] == null) {
// params[i] = new String("NULL");
// }
// }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy