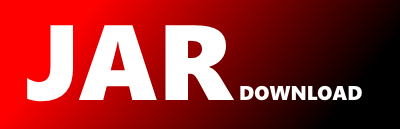
src-main.org.awakefw.sql.jdbc.http.JdbcHttpStatementTransfer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of awake-sql Show documentation
Show all versions of awake-sql Show documentation
Awake SQL is an open source framework that allows remote and secure JDBC access through HTTP.
The newest version!
/*
* This file is part of Awake SQL.
* Awake SQL: Remote JDBC access over HTTP.
* Copyright (C) 2013, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* Awake SQL is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Awake SQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*
* If you develop commercial activities using Awake SQL, you must:
* a) disclose and distribute all source code of your own product,
* b) license your own product under the GNU General Public License.
*
* You can be released from the requirements of the license by
* purchasing a commercial license. Buying such a license will allow you
* to ship Awake SQL with your closed source products without disclosing
* the source code.
*
* For more information, please contact KawanSoft SAS at this
* address: [email protected]
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.awakefw.sql.jdbc.http;
import java.io.File;
import java.io.IOException;
import java.sql.SQLException;
import java.util.List;
import java.util.Vector;
import org.apache.http.message.BasicNameValuePair;
import org.awakefw.commons.api.client.InvalidLoginException;
import org.awakefw.file.api.util.AwakeDebug;
import org.awakefw.file.http.HttpTransfer;
import org.awakefw.file.http.HttpTransferOne;
import org.awakefw.file.util.AwakeFileUtil;
import org.awakefw.file.util.KeepTempFilePolicyParms;
import org.awakefw.file.util.Tag;
import org.awakefw.file.util.parms.Parameter;
import org.awakefw.file.util.parms.ReturnCode;
import org.awakefw.sql.api.client.InvalidatedSessionException;
import org.awakefw.sql.jdbc.ConnectionHttp;
import org.awakefw.sql.jdbc.util.StatementHolderFileList;
import org.awakefw.sql.jdbc.util.StatementHolderListEncryptor;
import org.awakefw.sql.json.ConnectionHolder;
import org.awakefw.sql.json.ConnectionHolderTransport;
import org.awakefw.sql.json.StatementHolder;
import org.awakefw.sql.json.StatementHolderTransport;
import org.awakefw.sql.transport.TransportConverter;
import org.awakefw.sql.util.ConnectionParms;
import org.awakefw.sql.util.SqlAction;
import org.awakefw.sql.util.SqlReturnCode;
/**
*
* Class that execute all http transfer to the server, and gets back the results
*
*/
public class JdbcHttpStatementTransfer {
/** Set to true to display/log debug info */
private static boolean DEBUG = AwakeDebug
.isSet(JdbcHttpStatementTransfer.class);
/** The Http Connection */
private ConnectionHttp connectionHttp = null;
/** The Authentication Token */
private String authenticationToken = null;
/**
* Protected constructor, not callable
*/
protected JdbcHttpStatementTransfer() {
}
/**
* Constructor
*
* @param connectionHttp
* the http Connection
* @param authenticationToken
* the Http Connection Authentication Token
*/
public JdbcHttpStatementTransfer(ConnectionHttp connectionHttp,
String authenticationToken) {
this.connectionHttp = connectionHttp;
this.authenticationToken = authenticationToken;
debug(new java.util.Date() + " Uploading statement file...");
}
/**
* Execute a remote sql execute query statement and get the the file of the
* result
*
* @param statementHolder
* the statement to execute on server
*
* @return the received file
*/
public File getFileFromExecuteQueryOnServer(StatementHolder statementHolder)
throws SQLException {
HttpTransfer httpTransfer = null;
httpTransfer = new HttpTransferOne(connectionHttp.getUrl(),
connectionHttp.getHttpProxy(),
connectionHttp.getHttpProtocolParameters(),
connectionHttp.getAwakeProgressManager());
// Prepare the request parameters
List requestParams = new Vector();
requestParams.add(new BasicNameValuePair(Parameter.ACTION,
SqlAction.ACTION_SQL_STATEMENT));
requestParams.add(new BasicNameValuePair(Parameter.USERNAME,
connectionHttp.getUsername()));
requestParams.add(new BasicNameValuePair(Parameter.TOKEN,
authenticationToken));
requestParams.add(new BasicNameValuePair(ConnectionParms.CONNECTION_ID,
connectionHttp.getConnectionId() ));
// Store the Connections commit mode / read only mode / transaction
// isolation
ConnectionHolder connectionHolder = new ConnectionHolder(connectionHttp);
String jsonString = ConnectionHolderTransport.toJson(connectionHolder);
requestParams.add(new BasicNameValuePair(SqlAction.CONNECTION_HOLDER,
jsonString));
// Execute the statement waiting
List statementHolderList = new Vector();
statementHolderList.add(statementHolder); // Only one StatementHolder
StatementHolderListEncryptor.encrypt(statementHolderList, connectionHttp);
jsonString = StatementHolderTransport.toJson(statementHolderList);
requestParams.add(new BasicNameValuePair(SqlAction.STATEMENT_HOLDER,
jsonString));
httpTransfer.setReceiveInFile(true); // To say we get the result into a
// file
File receiveFile = null;
try {
httpTransfer.send(requestParams);
receiveFile = httpTransfer.getReceiveFile();
String receive = AwakeFileUtil.getFirstLineOfFile(receiveFile);
debug("httpTransfer.getReceiveFile(): " + receiveFile + ":");
if (receive.startsWith(ReturnCode.INVALID_LOGIN_OR_PASSWORD)) {
if (!DEBUG && !KeepTempFilePolicyParms.KEEP_TEMP_FILE) {
receiveFile.delete();
}
throw new InvalidLoginException();
}
if (receive.startsWith(SqlReturnCode.SESSION_INVALIDATED)) {
throw new SQLException(new InvalidatedSessionException());
}
} catch (Exception e) {
JdbcHttpTransferUtil.wrapExceptionAsSQLException(e);
}
// We are done: return the received string
return receiveFile;
}
/**
* Execute a remote sql execute update statement and get the string
*
* @param statementHolderFileList
* the list of statement + parameters to execute
* @return the result of the execute update
*/
public String getStringFromExecuteUpdateListOnServer(
StatementHolderFileList statementHolderFileList ) throws SQLException {
// First, we close the file!
statementHolderFileList.close();
if (statementHolderFileList.isEmpty()) {
String message = Tag.AWAKE_PRODUCT_FAIL
+ "Nothing to execute: statementHolderList is empty!";
throw new SQLException(message, new IOException(message));
}
HttpTransfer httpTransfer = null;
//debug("this.connectionHttp.getHost() : " + connectionHttp.getUrl());
// Launch the Servlet
httpTransfer = new HttpTransferOne(connectionHttp.getUrl(),
connectionHttp.getHttpProxy(),
connectionHttp.getHttpProtocolParameters(),
connectionHttp.getAwakeProgressManager());
List requestParams = new Vector();
requestParams.add(new BasicNameValuePair(Parameter.ACTION,
SqlAction.ACTION_SQL_STATEMENT));
requestParams.add(new BasicNameValuePair(Parameter.USERNAME,
connectionHttp.getUsername()));
requestParams.add(new BasicNameValuePair(Parameter.TOKEN,
this.authenticationToken));
requestParams.add(new BasicNameValuePair(ConnectionParms.CONNECTION_ID,
connectionHttp.getConnectionId() ));
// Store the Connections commit mode / read only mode / transaction
// isolation
ConnectionHolder connectionHolder = new ConnectionHolder(
this.connectionHttp);
String jsonString = ConnectionHolderTransport.toJson(connectionHolder);
requestParams.add(new BasicNameValuePair(SqlAction.CONNECTION_HOLDER,
jsonString));
// Execute all the statements waiting
if (statementHolderFileList.size() < connectionHttp
.getMaxStatementsForMemoryTransport()) {
jsonString = StatementHolderTransport.toJson(statementHolderFileList.getStatementHolderList());
requestParams.add(new BasicNameValuePair(
SqlAction.STATEMENT_HOLDER, jsonString));
// Delete the associated file
statementHolderFileList.delete();
try {
httpTransfer.send(requestParams);
} catch (Exception e) {
JdbcHttpTransferUtil.wrapExceptionAsSQLException(e);
}
} else {
//debug("StatementHolderFileList.size() >= maxStatementsForMemoryTransport)");
JdbcHttpFileTransfer jdbcHttpFileTransfer = new JdbcHttpFileTransfer(
this.connectionHttp);
jdbcHttpFileTransfer.uploadFile(
statementHolderFileList.getLocalFile(),
statementHolderFileList.getRemoteFileName());
String statementHolderParam = TransportConverter.AWAKE_STREAM_FILE
+ statementHolderFileList.getRemoteFileName();
requestParams.add(new BasicNameValuePair(
SqlAction.STATEMENT_HOLDER, statementHolderParam));
try {
httpTransfer.send(requestParams);
} catch (Exception e) {
JdbcHttpTransferUtil.wrapExceptionAsSQLException(e);
}
}
debug(new java.util.Date() + " Upload and processing done...");
String receive = httpTransfer.recv();
debug("httpTransfer.recv(): " + receive + ":");
if (receive.startsWith(ReturnCode.INVALID_LOGIN_OR_PASSWORD)) {
throw new SQLException(new InvalidLoginException());
}
if (receive.startsWith(SqlReturnCode.SESSION_INVALIDATED)) {
throw new SQLException(new InvalidatedSessionException());
}
// We are done: return the received string
return receive;
}
/**
* Debug tool
*
* @param s
*/
// @SuppressWarnings("unused")
private void debug(String s) {
if (DEBUG) {
//AwakeLogger.log(s);
System.out.println(s);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy